Library for MAX31850 thermocouple temperature sensor
LinkedList< retT > Class Template Reference
Example using the LinkedList Class. More...
#include <LinkedList.h>
Public Member Functions | |
LinkedList () | |
Create the LinkedList object. | |
~LinkedList () | |
Deconstructor for the LinkedList object Removes any members. | |
retT * | push (void *data) |
Add a member to the begining of the list. | |
retT * | append (void *data) |
Add a member to some position in the list. | |
retT * | remove (uint32_t loc) |
Remove a member from the list. | |
retT * | pop (uint32_t loc) |
Get access to a member from the list. | |
uint32_t | length (void) |
Get the length of the list. |
Detailed Description
template<class retT>
class LinkedList< retT >
Example using the LinkedList Class.
#include "mbed.h" #include "LinkedList.h" LinkedList<node> list; int main() { node *tmp; list.push((char *)"Two\n"); list.append((char *)"Three\n"); list.append((char *)"Four\n"); list.push((char*)"One\n"); list.append((char*)"Five\n"); for(int i=1; i<=list.length(); i++) { tmp = list.pop(i); printf("%s", (char *)tmp->data ); } error("done\n"); }
API abstraction for a Linked List
Definition at line 72 of file LinkedList.h.
Constructor & Destructor Documentation
LinkedList | ( | ) |
Create the LinkedList object.
Definition at line 26 of file LinkedList.cpp.
~LinkedList | ( | ) |
Deconstructor for the LinkedList object Removes any members.
Definition at line 35 of file LinkedList.cpp.
Member Function Documentation
retT * append | ( | void * | data ) |
Add a member to some position in the list.
- Parameters:
-
data - Some data type that is added to the list loc - Place in the list to put the data
- Returns:
- The member that was just inserted (NULL if empty) Add a member to the end of the list
- Parameters:
-
data - Some data type that is added to the list
- Returns:
- The member that was just inserted (NULL if empty)
Definition at line 92 of file LinkedList.cpp.
uint32_t length | ( | void | ) |
Get the length of the list.
- Returns:
- The number of members in the list
Definition at line 174 of file LinkedList.cpp.
retT * pop | ( | uint32_t | loc ) |
Get access to a member from the list.
- Parameters:
-
loc - The location of the member to access
- Returns:
- The member that was just requested (NULL if empty or out of bounds)
Definition at line 156 of file LinkedList.cpp.
retT * push | ( | void * | data ) |
Add a member to the begining of the list.
- Parameters:
-
data - Some data type that is added to the list
- Returns:
- The member that was just inserted (NULL if empty)
Definition at line 44 of file LinkedList.cpp.
retT * remove | ( | uint32_t | loc ) |
Remove a member from the list.
- Parameters:
-
loc - The location of the member to remove
- Returns:
- The head of the list
Definition at line 126 of file LinkedList.cpp.
Generated on Wed Jul 13 2022 22:04:08 by
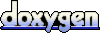