Library for MAX31850 thermocouple temperature sensor
Embed:
(wiki syntax)
Show/hide line numbers
MAX31850.h
00001 /* mbed MAX31850 00002 Library, for the Dallas (Maxim) 1-Wire Digital Thermometer 00003 * Copyright (c) 2010, Michael Hagberg Michael@RedBoxCode.com 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 * 00023 * 00024 * This library has been adapted from the DS1820 library to work with MAX31850 thermocouple sensor 00025 * Chris Roberts (croberts21@my.whitworth.edu) 00026 * Craig Russell (crussell21@my.whitworth.edu) 00027 * 00028 * For our application, we modified readAll() (previously convertTemperature) to force a small 00029 * wait rather than it being optional, and removed the ability to select a single sensor. 00030 * 00031 * getTemp() (previously temperature) has been updated to deal with the correct number of bits that 00032 * the temperature is stored in as well as updated convertions to all four units 00033 * 00034 * as well as adding a setUnits() function to set the 00035 * units that the sensor will return. 00036 * 00037 * We removed some functionality that dealt with parasite power. 00038 * 00039 * 00040 */ 00041 00042 #ifndef MBED_MAX31850_H 00043 #define MBED_MAX31850_H 00044 00045 #include "mbed.h" 00046 #include "LinkedList.h" 00047 00048 #define C 0 //celsius 00049 #define K 1 //Kelvin 00050 #define F 2 //fahrenheit 00051 #define R 3 //Rankin 00052 00053 extern Serial pc; 00054 00055 /** MAX31850 00056 * 00057 * Example: 00058 * @code 00059 * #include "mbed.h" 00060 * #include "MAX31850.h" 00061 * 00062 * MAX31850 probe(DATA_PIN); 00063 * 00064 * int main() { 00065 * while(true) { 00066 * probe.convertTemperature(true, MAX31850::all_devices); //Start temperature conversion, wait until ready 00067 * printf("It is %3.1foC\r\n", probe.temperature()); 00068 * wait(1); 00069 * } 00070 * } 00071 * @endcode 00072 */ 00073 class MAX31850 00074 { 00075 public: 00076 enum devices{ 00077 this_device, // command applies to only this device 00078 all_devices }; // command applies to all devices 00079 00080 enum { 00081 invalid_conversion = -1000 00082 }; 00083 00084 /** Create a probe object connected to the specified pins 00085 * 00086 * The probe might either by regular powered or parasite powered. If it is parasite 00087 * powered and power_pin is set, that pin will be used to switch an external mosfet connecting 00088 * data to Vdd. If it is parasite powered and the pin is not set, the regular data pin 00089 * is used to supply extra power when required. This will be sufficient as long as the 00090 * number of probes is limitted. 00091 * 00092 * For our application, we have the functions set up to only use external power. The constructor still supports 00093 * parasite power but the functions to not support it. 00094 */ 00095 MAX31850 (PinName data_pin, PinName power_pin = NC, bool power_polarity = 0); // Constructor with parasite power pin 00096 00097 ~MAX31850(); 00098 00099 /** Function to see if there are MAX31850 00100 * devices left on a pin which do not have a corresponding MAX31850 00101 * object 00102 * 00103 * @return - true if there are one or more unassigned devices, otherwise false 00104 */ 00105 static bool unassignedProbe(PinName pin); 00106 00107 /** This routine will initiate the temperature conversion within 00108 * all MAX31850 00109 * probes. 00110 */ 00111 void readAll(); 00112 00113 /** This function will return the probe temperature. Approximately 10ms per 00114 * probe to read its scratchpad, do CRC check and convert temperature on the LPC1768. 00115 * 00116 * @scratchpad scale, may be either 'c', 'f', 'k', and 'r' 00117 * @returns temperature for that scale, or MAX31850::invalid_conversion (-1000) if CRC error detected. 00118 */ 00119 float getTemp(int scale = 4); 00120 00121 /** This function returns the 64 bit address associated with a 00122 * particular DS18B20. 00123 * 00124 * Contributed by John M. Larkin (jlarkin@whitworth.edu) 00125 * 00126 * @returns unsigned long long 00127 */ 00128 unsigned long long getId(); 00129 00130 00131 /** This function sets the _units variable to the 00132 * users desired temperature units 00133 */ 00134 void setUnits(int); 00135 00136 00137 private: 00138 bool _parasite_power; 00139 bool _power_mosfet; 00140 bool _power_polarity; 00141 00142 static char CRC_byte(char _CRC, char byte ); 00143 static bool onewire_reset(DigitalInOut *pin); 00144 void match_ROM(); 00145 void skip_ROM(); 00146 static bool search_ROM_routine(DigitalInOut *pin, char command, char *ROM_address); 00147 static void onewire_bit_out (DigitalInOut *pin, bool bit_data); 00148 void onewire_byte_out(char data); 00149 static bool onewire_bit_in(DigitalInOut *pin); 00150 char onewire_byte_in(); 00151 static bool ROM_checksum_error(char *_ROM_address); 00152 bool scratchpad_checksum_error(); 00153 void read_scratchpad(); 00154 static bool unassignedProbe(DigitalInOut *pin, char *ROM_address); 00155 00156 DigitalInOut _datapin; 00157 DigitalOut _parasitepin; 00158 00159 char _ROM[8]; 00160 char scratchpad[9]; 00161 int _units; 00162 00163 static LinkedList<node> probes; 00164 }; 00165 #endif
Generated on Wed Jul 13 2022 22:04:08 by
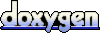