
fft test software using cmsis DSP library FRDM k22f
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Print messages when the AnalogIn is greater than 50% 00002 00003 #include "mbed.h" 00004 #include "arm_math.h" 00005 #include "arm_const_structs.h" 00006 00007 00008 #define led_on 0x00 00009 #define led_off 0x01 00010 00011 DigitalOut led_r(PTA1); 00012 DigitalOut led_g(PTA2); 00013 DigitalOut led_b(PTD5); 00014 00015 const int FFT_LEN = 1024; 00016 const int bins = 32; 00017 00018 00019 const static arm_cfft_instance_f32 *S; 00020 00021 float samples[FFT_LEN*2]; 00022 float magnitudes[FFT_LEN]; 00023 float freq_window[bins]; 00024 00025 00026 int main() 00027 { //initialize led to all off 00028 led_r.write(led_off); 00029 led_g.write(led_off); 00030 led_b.write(led_off); 00031 00032 00033 int32_t i = 0; 00034 printf("\r\n\r\nFFT test program!\r\n"); 00035 printf("by Curtis Mattull\r\n\r\n\r\n"); 00036 00037 00038 // Init arm_ccft_32 00039 switch (FFT_LEN) 00040 { 00041 case 16: 00042 S = & arm_cfft_sR_f32_len16; 00043 break; 00044 case 32: 00045 S = & arm_cfft_sR_f32_len32; 00046 break; 00047 case 64: 00048 S = & arm_cfft_sR_f32_len64; 00049 break; 00050 case 128: 00051 S = & arm_cfft_sR_f32_len128; 00052 break; 00053 case 256: 00054 S = & arm_cfft_sR_f32_len256; 00055 break; 00056 case 512: 00057 S = & arm_cfft_sR_f32_len512; 00058 break; 00059 case 1024: 00060 S = & arm_cfft_sR_f32_len1024; 00061 break; 00062 case 2048: 00063 S = & arm_cfft_sR_f32_len2048; 00064 break; 00065 case 4096: 00066 S = & arm_cfft_sR_f32_len4096; 00067 break; 00068 } 00069 00070 /*populate some dummy sin data*/ 00071 for(i = 0; i< FFT_LEN*2; i+=2) 00072 { 00073 //fast math sine table[512+1] 00074 samples[i] = sinTable_f32[i/4]; 00075 samples[i+1] = 0; 00076 } 00077 00078 /*multiply the dummy data with more dummy data*/ 00079 // for(i = 0; i< FFT_LEN*2; i+=2) 00080 // { 00081 // //fast math sine table[512+1] 00082 // samples[i] +=sinTable_f32[i/2] ; 00083 // samples[i+1] = 0; 00084 // } 00085 00086 // Run FFT on sample data. 00087 arm_cfft_f32(S, samples, 0, 1); 00088 // Calculate magnitude of complex numbers output by the FFT. 00089 arm_cmplx_mag_f32(samples, magnitudes, FFT_LEN); 00090 00091 printf("\r\nThe first 20 bins:\r\n"); 00092 00093 for(i = 0; i< 20; i++) 00094 { 00095 printf("magnitudes[%i]: %f\r\n",i,magnitudes[i]); 00096 } 00097 00098 led_g.write(led_on); 00099 00100 printf("test complete\r\n"); 00101 wait(1); 00102 while(1) 00103 { 00104 00105 } 00106 }
Generated on Wed Jul 13 2022 21:57:07 by
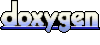