
Wheelchair control
Fork of wheelchaircontrol by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "wheelchair.h" 00002 00003 // Initialize Pins 00004 AnalogIn x(A0); 00005 AnalogIn y(A1); 00006 00007 DigitalOut off(D0); 00008 DigitalOut on(D1); 00009 DigitalOut up(D2); 00010 DigitalOut down(D3); 00011 00012 Wheelchair smart(xDir,yDir); 00013 00014 int main(void) 00015 { 00016 on = 1; 00017 while(1){ 00018 /* 00019 // Waiting for command 00020 if (pc.readable()) { 00021 char c = pc.getc(); 00022 00023 // Move Forward 00024 if (c == 'w') { 00025 pc.printf("up \n"); 00026 smart.forward(); 00027 } 00028 00029 // Move Left 00030 else if (c == 'd') { 00031 pc.printf("left \n"); 00032 smart.left(); 00033 } 00034 00035 // Move Right 00036 else if (c == 'a') { 00037 pc.printf("right \n"); 00038 smart.right(); 00039 } 00040 00041 // Move Backward 00042 else if (c == 's') { 00043 pc.printf("down \n"); 00044 smart.backward(); 00045 } 00046 00047 // Read non-move commands 00048 else { 00049 pc.printf("none \n"); 00050 smart.stop(); 00051 00052 // Turn On 00053 if (c == 'o') { 00054 pc.printf("Turning on.\n"); 00055 on = 0; 00056 wait(process); 00057 on = 1; 00058 } 00059 00060 // Turn Off 00061 else if (c == 'k') { 00062 off = 0; 00063 wait(process); 00064 off = 1; 00065 } 00066 00067 00068 else if (c == 'u') { 00069 up = 0; 00070 wait(process); 00071 up = 1; 00072 } 00073 00074 else if (c == 'p') { 00075 down = 0; 00076 wait(process); 00077 down = 1; 00078 } 00079 } 00080 } 00081 00082 else { 00083 pc.printf("Nothing pressed.\n"); 00084 smart.stop(); 00085 } 00086 */ 00087 00088 smart.move(x,y); 00089 wait(process); 00090 } 00091 } 00092
Generated on Thu Jul 14 2022 08:30:56 by
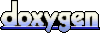