
init
Dependencies: mbed C12832 EthernetInterface MQTT mbed-rtos picojson
MathAlgo.hpp
00001 // 00002 // Created by Marc Le Labourier 00003 // 14-Feb-16. 00004 // 00005 00006 #ifndef MATHALGO_H 00007 #define MATHALGO_H 00008 00009 #include <cmath> 00010 #include <sstream> 00011 #define VAR_PI 3.141592653589793238462643383279502884L /* pi */ 00012 #define DIFF(x, y) (x - y) 00013 #define ABS(x) (x < 0 ? x * -1.0f : x) 00014 00015 /* Library of many useful peace of code */ 00016 00017 enum Mode { 00018 Digital, 00019 Degree, 00020 Radian 00021 }; 00022 00023 namespace mathalgo 00024 { 00025 00026 inline std::string modeToString(Mode m) 00027 { 00028 switch (m) { 00029 case Digital: return "DIGITAL"; 00030 case Degree: return "DEGREE"; 00031 case Radian: return "RADIAN"; 00032 } 00033 return "DIGITAL"; 00034 } 00035 00036 inline Mode modeFromString(const std::string& s) 00037 { 00038 if (s.compare("DEGREE") == 0) 00039 return Degree; 00040 else if (s.compare("RADIAN") == 0) 00041 return Radian; 00042 return Digital; 00043 } 00044 00045 // How to round a double to a given precision. 00046 // From: http://stackoverflow.com/questions/11208971/round-a-float-to-a-given-precision 00047 inline double pround(double x, int precision) 00048 { 00049 if (x == 0.) 00050 return x; 00051 int ex = (int)std::floor(std::log10(std::abs(x))) - precision + 1; 00052 double div = std::pow(10., ex); 00053 return std::floor(x / div + 0.5) * div; 00054 } 00055 00056 inline double limit(double val, double upper, double lower) 00057 { 00058 return (val > upper ? upper : (val < lower ? lower : val)); 00059 } 00060 00061 // Conversion of digital value from [0, 1] to degree or rad. 00062 inline double digitalToDegree(double val) 00063 { 00064 return val * 360.0; 00065 } 00066 00067 inline double degreeToDigital(double val) 00068 { 00069 return val / 360.0; 00070 } 00071 00072 inline double digitalToRadian(double val) 00073 { 00074 return (digitalToDegree(val) * (VAR_PI / 180.0)); 00075 } 00076 00077 inline double radianToDigital(double val) 00078 { 00079 return degreeToDigital(val / (VAR_PI / 180.0)); 00080 } 00081 00082 // Convert float to string without c++11 00083 inline std::string stof (float number){ 00084 std::ostringstream buff; 00085 buff << number; 00086 return buff.str(); 00087 } 00088 00089 // Convert int to string without c++11 00090 inline std::string stoi (int number){ 00091 std::ostringstream buff; 00092 buff << number; 00093 return buff.str(); 00094 } 00095 } 00096 00097 #endif // MATHALGO_H
Generated on Tue Jul 26 2022 08:32:45 by
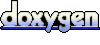