
Lab4
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Documentation: 00003 * http://www.zagrosrobotics.com/files/easyvr-user-manual-30.pdf 00004 */ 00005 00006 #include "easyvr.h" 00007 00008 #define BUF_SIZE 32 00009 #define NUM_NOTES 8 00010 00011 EasyVR easyVR( p28, p27 ); 00012 Serial term( USBTX, USBRX ); 00013 PwmOut speaker( p21 ); 00014 InterruptIn hold_button( p8 ); 00015 00016 // C major scale starting at C4 00017 char *note_table[] = { "C4", "D4", "E4", "F4", "G4", "A4", "B4", "C5" }; 00018 float freq_table[] = { 261.63, 293.66, 329.63, 349.23, 392.0, 440.0, 493.88, 523.25 }; 00019 00020 char note_buffer[BUF_SIZE]; 00021 short note_buffer_pos = 0; 00022 bool do_read = false; 00023 00024 void print_error( int error ) 00025 { 00026 switch( error ) 00027 { 00028 case ERR_DATACOL_TOO_NOISY: 00029 term.printf( "Too noisy, try again\r\n" ); 00030 break; 00031 case ERR_DATACOL_TOO_SOFT: 00032 term.printf( "Spoke too softly, try again\r\n" ); 00033 break; 00034 case ERR_DATACOL_TOO_LOUD: 00035 term.printf( "Spoke too loudly, try again\r\n" ); 00036 break; 00037 case ERR_DATACOL_TOO_SOON: 00038 term.printf( "Spoke too soon, try again\r\n" ); 00039 break; 00040 case ERR_DATACOL_TOO_CHOPPY: 00041 term.printf( "Too complex, try again\r\n" ); 00042 break; 00043 case ERR_RECOG_FAIL: 00044 term.printf( "Recognition failed, try again\r\n" ); 00045 break; 00046 case ERR_RECOG_LOW_CONF: 00047 term.printf( "Recognition result status: doubtful, try again\r\n" ); 00048 break; 00049 case ERR_RECOG_MID_CONF: 00050 term.printf( "Recognition result status: maybe, try again\r\n" ); 00051 break; 00052 case ERR_RECOG_BAD_TEMPLATE: 00053 term.printf( "Invalid command stored in memory\r\n" ); 00054 break; 00055 case ERR_RECOG_DURATION: 00056 term.printf( "Bad duration patterns\r\n" ); 00057 break; 00058 case ERR_SYNTH_BAD_VERSION: 00059 term.printf( "Bad release number in speech file\r\n" ); 00060 break; 00061 case ERR_SYNTH_BAD_MSG: 00062 term.printf( "Bad data in speech file\r\n" ); 00063 break; 00064 case ERR_NOT_A_WORD: 00065 term.printf( "Spoken word is not in vocabulary\r\n" ); 00066 break; 00067 default: 00068 break; 00069 } 00070 } 00071 00072 void add_command( int index ) 00073 { 00074 char command_num = i2a( index ); 00075 char note = note_table[index][0]; 00076 char scale = (char)( note_table[index][1] + 17 ); 00077 00078 char err; 00079 00080 easyVR.send( CMD_GROUP_SD ); 00081 easyVR.send( i2a( 1 ) ); 00082 easyVR.send( command_num ); 00083 00084 if( ( err = easyVR.receive() ) != STS_SUCCESS ) 00085 term.printf( "(in add_command( %s ) line %d) Error %c\r\n", note_table[index], __LINE__, err ); 00086 00087 easyVR.send( CMD_NAME_SD ); 00088 easyVR.send( i2a( 1 ) ); 00089 easyVR.send( command_num ); 00090 easyVR.send( i2a( 3 ) ); 00091 easyVR.send( note ); 00092 easyVR.send( '^' ); 00093 easyVR.send( scale ); 00094 00095 if( ( err = easyVR.receive() ) != STS_SUCCESS ) 00096 term.printf( "(in add_command( %s ) line %d) Error %c\r\n", note_table[index], __LINE__, err ); 00097 } 00098 00099 char train_command( int index ) 00100 { 00101 char command_num = i2a( index ); 00102 char note = note_table[index][0]; 00103 char scale = (char)( note_table[index][1] + 17 ); 00104 00105 term.printf( "Please say: %s\r\n", note_table[index] ); 00106 00107 easyVR.send( CMD_TRAIN_SD ); 00108 easyVR.send( i2a( 1 ) ); 00109 easyVR.send( command_num ); 00110 00111 char recv, err, retval; 00112 00113 switch( ( recv = easyVR.receive() ) ) 00114 { 00115 case STS_SUCCESS: 00116 term.printf( "Successfully trained command %c^%c\r\n", note, scale ); 00117 retval = recv; 00118 break; 00119 case STS_RESULT: 00120 easyVR.send( ARG_ACK ); 00121 err = a2i( easyVR.receive() ); 00122 term.printf( "Successfully trained command %c^%c, but similar to SD command %d\r\n", note, scale, err ); 00123 retval = STS_SUCCESS; 00124 break; 00125 case STS_SIMILAR: 00126 easyVR.send( ARG_ACK ); 00127 err = a2i( easyVR.receive() ); 00128 term.printf( "Successfully trained command %c^%c, but similar to SI command %d\r\n", note, scale, err ); 00129 retval = STS_SUCCESS; 00130 break; 00131 case STS_TIMEOUT: 00132 term.printf( "Timed out trying to train %c^%c!\r\n", note, scale ); 00133 retval = recv; 00134 break; 00135 case STS_ERROR: 00136 easyVR.send( ARG_ACK ); 00137 err = a2i( easyVR.receive() ) * 16; 00138 easyVR.send( ARG_ACK ); 00139 err += a2i( easyVR.receive() ); 00140 print_error( err ); 00141 retval = err; 00142 break; 00143 default: 00144 term.printf( "(in train_command( %s ) line %d) Error %c\r\n", note_table[index], __LINE__, recv ); 00145 retval = recv; 00146 break; 00147 } 00148 00149 return retval; 00150 } 00151 00152 void enable_read() 00153 { 00154 do_read = true; 00155 } 00156 00157 void play_buffer() 00158 { 00159 do_read = false; 00160 easyVR.send( CMD_BREAK ); 00161 00162 for( int i = 0; i < note_buffer_pos; i++ ) 00163 { 00164 speaker.period( 1.0 / freq_table[note_buffer[i]] ); 00165 speaker = 0.25; 00166 wait( 0.5 ); 00167 } 00168 00169 speaker = 0; 00170 note_buffer_pos = 0; 00171 } 00172 00173 int main( void ) 00174 { 00175 term.baud( 19200 ); 00176 00177 easyVR.wakeup(); 00178 easyVR.setup( 0, 3 ); 00179 00180 char err; 00181 00182 easyVR.baud( 9600 ); 00183 easyVR.send( CMD_BAUDRATE ); 00184 easyVR.send( i2a( 12 ) ); 00185 00186 if( ( err = easyVR.receive() ) != STS_SUCCESS ) 00187 term.printf( "(in main() line %d) Error: %c\r\n", __LINE__, err ); 00188 00189 easyVR.send( CMD_LEVEL ); 00190 easyVR.send( i2a( 1 ) ); 00191 00192 if( ( err = easyVR.receive() ) != STS_SUCCESS ) 00193 term.printf( "(in main() line %d) Error: %c\r\n", __LINE__, err ); 00194 00195 /* 00196 int result; 00197 00198 for( int i = 0; i < NUM_NOTES; i++ ) 00199 { 00200 add_command( i ); 00201 00202 do 00203 { 00204 result = train_command( i ); 00205 wait( 0.5 ); 00206 } while( result != STS_SUCCESS ); 00207 } 00208 */ 00209 00210 hold_button.rise( enable_read ); 00211 hold_button.fall( play_buffer ); 00212 00213 char note, recv; 00214 00215 while( 1 ) 00216 { 00217 while( do_read ) 00218 { 00219 term.printf( "Ready!\r\n" ); 00220 00221 easyVR.send( CMD_RECOG_SI ); 00222 easyVR.send( i2a( 3 ) ); 00223 00224 switch( ( recv = easyVR.receive() ) ) 00225 { 00226 case STS_SIMILAR: 00227 easyVR.send( ARG_ACK ); 00228 note = a2i( easyVR.receive() ); 00229 00230 if( note < NUM_NOTES ) 00231 { 00232 term.printf( "Heard: %s\r\n", note_table[note] ); 00233 00234 if( note_buffer_pos < BUF_SIZE ) 00235 { 00236 note_buffer[note_buffer_pos] = note; 00237 note_buffer_pos++; 00238 } 00239 else 00240 term.printf( "Buffer full\r\n" ); 00241 } 00242 else 00243 term.printf( "Heard SI command %d\r\n", note ); 00244 00245 break; 00246 /* 00247 case STS_SIMILAR: 00248 easyVR.send( ARG_ACK ); 00249 err = a2i( easyVR.receive() ); 00250 term.printf( "Heard similar to SI command %d\r\n", err ); 00251 break; 00252 */ 00253 case STS_TIMEOUT: 00254 term.printf( "Timed out\r\n" ); 00255 break; 00256 case STS_ERROR: 00257 easyVR.send( ARG_ACK ); 00258 err = a2i( easyVR.receive() ) * 16; 00259 easyVR.send( ARG_ACK ); 00260 err += a2i( easyVR.receive() ); 00261 print_error( err ); 00262 break; 00263 default: 00264 if( recv != STS_INTERR ) 00265 term.printf( "(in main() line %d) Error %c\r\n", __LINE__, recv ); 00266 00267 break; 00268 } 00269 } 00270 } 00271 }
Generated on Sun Jul 17 2022 16:13:19 by
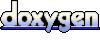