Serial Monitor using a specific PC application: XMON
Embed:
(wiki syntax)
Show/hide line numbers
XMONlib.cpp
00001 /* mbed XMON library. 00002 00003 XMON Micro controller serial port debugger variable view realtime curve tracer 00004 http://webx.dk/XMON/index.htm 00005 00006 XMON is a windows program that show and plot value received fomr the serial port. 00007 This is the banner from the XMON documentation: 00008 // XMON 2.1 ALPHA (autoscaling on/off/min/max) 15 feb 2008 00009 // XMON is a free utility for monitoring and communication with MCU development 00010 // Made by DZL (help ideas support testing TST) 00011 // if this program help you and you are happy to use it, please donate a fee to thomas@webx.dk via paypal 00012 // any donation will be put into improving XMON, any size of donation small or big will be most appliciated. 00013 // suggestions and bugs to thomas@webx.dk 00014 // we will setup a homepage with features and tips and tricks and so on soon, see webx.dk and dzl.dk 00015 00016 Copyright (c) 2011 NXP 3786 00017 00018 Permission is hereby granted, free of charge, to any person obtaining a copy 00019 of this software and associated documentation files (the "Software"), to deal 00020 in the Software without restriction, including without limitation the rights 00021 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00022 copies of the Software, and to permit persons to whom the Software is 00023 furnished to do so, subject to the following conditions: 00024 00025 The above copyright notice and this permission notice shall be included in 00026 all copies or substantial portions of the Software. 00027 00028 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00029 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00030 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00031 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00032 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00033 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00034 THE SOFTWARE. 00035 */ 00036 #include "mbed.h" 00037 #include "XMONlib.h" 00038 00039 XMON::XMON( PinName tx, PinName rx) : Serial(tx, rx) 00040 { 00041 } 00042 00043 /** Clear and restart x axis for the specified trace 00044 * 00045 * @param trace trace value 00046 */ 00047 void XMON::plotZero( unsigned int trace) 00048 { // value is the plot trace you want to zero on XMON 00049 putc('*'); 00050 putc('T'); // re Trigger 'T', clear all traces and restart X axis scaling 'C' 00051 putc( (unsigned char)trace); 00052 } 00053 00054 /** Clear all trace and restart x axis 00055 * @param none 00056 */ 00057 void XMON::plotZero( void) 00058 { 00059 putc('*'); 00060 putc('T'); // re Trigger 'T', clear 00061 putc( 0); 00062 00063 putc('*'); 00064 putc('T'); // re Trigger 'T', clear 00065 putc( 1); 00066 00067 putc('*'); 00068 putc('T'); // re Trigger 'T', clear 00069 putc( 2); 00070 00071 putc('*'); 00072 putc('T'); // re Trigger 'T', clear 00073 putc( 3); 00074 } 00075 00076 /** Plot float data 00077 * 00078 * @param data float value to plot. 00079 * @param trace color graph 00080 */ 00081 void XMON::plotf( float data, unsigned int trace) 00082 { 00083 putc('*'); 00084 putc('d'); 00085 putc(((char*)&data)[3]); 00086 putc('D'); 00087 putc(((char*)&data)[2]); 00088 putc('D'); 00089 putc(((char*)&data)[1]); 00090 putc('D'); 00091 putc(((char*)&data)[0]); 00092 putc('F'); // here is the data handler selector indicator to XMON 00093 putc( (unsigned char)trace); 00094 } 00095 00096 /** View float data 00097 * 00098 * @param data float value to view. 00099 * @param line line label 00100 */ 00101 void XMON::viewf( float data, unsigned int trace) 00102 { 00103 putc('*'); 00104 putc('d'); 00105 putc(((char*)&data)[3]); 00106 putc('D'); 00107 putc(((char*)&data)[2]); 00108 putc('D'); 00109 putc(((char*)&data)[1]); 00110 putc('D'); 00111 putc(((char*)&data)[0]); 00112 putc('f');// here is the data handler selector indicator to XMON 00113 putc( (unsigned char)trace); 00114 } 00115 00116 /** Plot signed 32 bits data 00117 * 00118 * @param data signed 32bit value to plot. 00119 * @param trace color graph 00120 */ 00121 void XMON::plotl( int data,unsigned int trace) 00122 { 00123 putc('*'); 00124 putc('d'); 00125 putc(((char*)&data)[3]); 00126 putc('D'); 00127 putc(((char*)&data)[2]); 00128 putc('D'); 00129 putc(((char*)&data)[1]); 00130 putc('D'); 00131 putc(((char*)&data)[0]); 00132 putc('I'); // here is the data handler selector indicator to XMON 00133 putc( (unsigned char)trace); 00134 } 00135 00136 /** View signed 32 bits data 00137 * 00138 * @param data unsigned short value to view. 00139 * @param line line label 00140 */ 00141 void XMON::viewl( int data, unsigned int trace) 00142 { 00143 putc('*'); 00144 putc('d'); 00145 putc(((char*)&data)[3]); 00146 putc('D'); 00147 putc(((char*)&data)[2]); 00148 putc('D'); 00149 putc(((char*)&data)[1]); 00150 putc('D'); 00151 putc(((char*)&data)[0]); 00152 putc('i'); // here is the data handler selector indicator to XMON 00153 putc( (unsigned char)trace); 00154 } 00155 00156 00157 /** Plot unsigned 16 bits data 00158 * 00159 * @param data unsigned short value to plot. 00160 * @param trace color graph 00161 */ 00162 void XMON::plot( unsigned short data, unsigned int trace) 00163 { 00164 putc('*'); 00165 putc('d'); 00166 putc( (unsigned char)(data>>8)); 00167 putc('D'); 00168 putc( (unsigned char)data); 00169 putc('P'); // here is the data handler selector indicator to XMON 00170 putc( (unsigned char)trace); 00171 } 00172 00173 /** View unsigned 16 bits data 00174 * 00175 * @param data unsigned short value to view. 00176 * @param line line label 00177 */ 00178 void XMON::view( unsigned short data, unsigned int line) // Unsigned Integers 16 bits values in the VIEW variable value area 00179 { 00180 putc('*'); 00181 putc('d'); 00182 putc( (unsigned char)(data>>8)); 00183 putc('D'); 00184 putc( (unsigned char)data); 00185 putc('V');// here is the data handler selector indicator to XMON 00186 putc( (unsigned char)line); 00187 } 00188 00189 /** Put the PLOT GRAPH in auto scale mode 00190 * 00191 * @param s 0 auto scale OFF, 1 auto scale ON 00192 */ 00193 void XMON::autoscale( char s) // 00194 { 00195 putc('*'); 00196 putc('A'); 00197 putc(s); 00198 } 00199 00200 /* 00201 00202 void ViewFreqBin( int data) 00203 { 00204 viewl( data, XMON_RED); 00205 00206 } 00207 00208 void PlotSpectrum( int * specarray, float data) 00209 { 00210 unsigned int i=0; 00211 00212 plotZero( XMON_RED); 00213 00214 viewf( data, XMON_RED); 00215 00216 while ( i < NBIN/2) 00217 { 00218 plot( specarray[i], XMON_RED); 00219 i++; 00220 } 00221 } 00222 00223 */
Generated on Wed Jul 13 2022 02:57:20 by
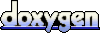