Library for MI0283QT-2 LCD
Embed:
(wiki syntax)
Show/hide line numbers
Touchlib.c
00001 /* mbed TouchScreen ADS7846 library. 00002 00003 Copyright (c) 2011 NXP 3803 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "string.h" 00026 #include "Touchlib.h" 00027 #include "calibrate.h" 00028 #include "crocino.h" 00029 00030 /* */ 00031 unsigned char TSCmd[cTS_CMDSIZE] = { 00032 cTS_GETY, 0x00, cTS_GETY, 0x00, /* y */ 00033 cTS_GETZ1, 0x00, cTS_GETZ1, 0x00, /* z1 */ 00034 cTS_GETZ2, 0x00, cTS_GETZ2, 0x00, /* z2 */ 00035 cTS_GETX, 0x00, cTS_END, 0x00, 0x00 /* x */ 00036 }; 00037 00038 #define cTS_SAMPLE 8 00039 #define MODE12 0 00040 00041 POINT crocino_coord[3] = { 00042 {80,120}, 00043 {240,60}, 00044 {240,180} 00045 }; 00046 00047 MATRIX TS_Matrix; 00048 00049 unsigned char TSValue[cTS_CMDSIZE]; 00050 unsigned int tap_debounce; 00051 00052 DigitalOut TS_DBG_LED(LED4); 00053 00054 TOUCHS::TOUCHS( PinName mosi, PinName miso, PinName sclk, PinName cs, PinName penirq) : _spi( mosi, miso, sclk), _cs( cs), _penirq( penirq) { 00055 // defaults params 00056 00057 } 00058 00059 unsigned int TOUCHS::setcalibration( _TS_COORD *ts) 00060 { 00061 POINT screen[3]; 00062 /* from struct TS_COORD to struct POINT. */ 00063 screen[0].x=ts[0].x; 00064 screen[0].y=ts[0].y; 00065 screen[1].x=ts[1].x; 00066 screen[1].y=ts[1].y; 00067 screen[2].x=ts[2].x; 00068 screen[2].y=ts[2].y; 00069 /* Now start the calibration process. */ 00070 ts_val.calibration_done=!setCalibrationMatrix( &crocino_coord[0], &screen[0], &TS_Matrix); 00071 /* Return the result. */ 00072 return( ts_val.calibration_done); 00073 } 00074 00075 unsigned int TOUCHS::calibrate( void) 00076 { 00077 POINT ts_tmp, coord; 00078 00079 coord.x=ts_val.coord.x; 00080 coord.y=ts_val.coord.y; 00081 // see calibrate.c 00082 getDisplayPoint( &ts_tmp, &coord, &TS_Matrix); 00083 // 00084 ts_val.coord.x = ts_tmp.x; 00085 ts_val.coord.y = ts_tmp.y; 00086 00087 return 0; 00088 } 00089 00090 unsigned int TOUCHS::crocino_size( void) 00091 { 00092 return( cTS_CROCINO); 00093 } 00094 00095 unsigned int TOUCHS::getcrocino_x( unsigned char idx) 00096 { 00097 if ( idx > 3) 00098 idx=3; 00099 00100 return ( crocino_coord[idx].x); 00101 } 00102 00103 unsigned int TOUCHS::getcrocino_y( unsigned char idx) 00104 { 00105 if ( idx > 3) 00106 idx=3; 00107 00108 return ( crocino_coord[idx].y); 00109 } 00110 00111 unsigned int TOUCHS::gettsmatrixsize( void) 00112 { 00113 return( sizeof( TS_Matrix)); 00114 } 00115 00116 void TOUCHS::gettsmatrix( unsigned char *pmatrix) 00117 { 00118 00119 *pmatrix++=((unsigned char*)&TS_Matrix.An)[0]; 00120 *pmatrix++=((unsigned char*)&TS_Matrix.An)[1]; 00121 *pmatrix++=((unsigned char*)&TS_Matrix.An)[2]; 00122 *pmatrix++=((unsigned char*)&TS_Matrix.An)[3]; 00123 *pmatrix++=((unsigned char*)&TS_Matrix.Bn)[0]; 00124 *pmatrix++=((unsigned char*)&TS_Matrix.Bn)[1]; 00125 *pmatrix++=((unsigned char*)&TS_Matrix.Bn)[2]; 00126 *pmatrix++=((unsigned char*)&TS_Matrix.Bn)[3]; 00127 *pmatrix++=((unsigned char*)&TS_Matrix.Cn)[0]; 00128 *pmatrix++=((unsigned char*)&TS_Matrix.Cn)[1]; 00129 *pmatrix++=((unsigned char*)&TS_Matrix.Cn)[2]; 00130 *pmatrix++=((unsigned char*)&TS_Matrix.Cn)[3]; 00131 *pmatrix++=((unsigned char*)&TS_Matrix.Dn)[0]; 00132 *pmatrix++=((unsigned char*)&TS_Matrix.Dn)[1]; 00133 *pmatrix++=((unsigned char*)&TS_Matrix.Dn)[2]; 00134 *pmatrix++=((unsigned char*)&TS_Matrix.Dn)[3]; 00135 *pmatrix++=((unsigned char*)&TS_Matrix.En)[0]; 00136 *pmatrix++=((unsigned char*)&TS_Matrix.En)[1]; 00137 *pmatrix++=((unsigned char*)&TS_Matrix.En)[2]; 00138 *pmatrix++=((unsigned char*)&TS_Matrix.En)[3]; 00139 *pmatrix++=((unsigned char*)&TS_Matrix.Fn)[0]; 00140 *pmatrix++=((unsigned char*)&TS_Matrix.Fn)[1]; 00141 *pmatrix++=((unsigned char*)&TS_Matrix.Fn)[2]; 00142 *pmatrix++=((unsigned char*)&TS_Matrix.Fn)[3]; 00143 *pmatrix++=((unsigned char*)&TS_Matrix.Divider)[0]; 00144 *pmatrix++=((unsigned char*)&TS_Matrix.Divider)[1]; 00145 *pmatrix++=((unsigned char*)&TS_Matrix.Divider)[2]; 00146 *pmatrix++=((unsigned char*)&TS_Matrix.Divider)[3]; 00147 00148 } 00149 00150 void TOUCHS::settsmatrix( unsigned char *pmatrix) 00151 { 00152 00153 ((unsigned char*)&TS_Matrix.An)[0]=*pmatrix++; 00154 ((unsigned char*)&TS_Matrix.An)[1]=*pmatrix++; 00155 ((unsigned char*)&TS_Matrix.An)[2]=*pmatrix++; 00156 ((unsigned char*)&TS_Matrix.An)[3]=*pmatrix++; 00157 ((unsigned char*)&TS_Matrix.Bn)[0]=*pmatrix++; 00158 ((unsigned char*)&TS_Matrix.Bn)[1]=*pmatrix++; 00159 ((unsigned char*)&TS_Matrix.Bn)[2]=*pmatrix++; 00160 ((unsigned char*)&TS_Matrix.Bn)[3]=*pmatrix++; 00161 ((unsigned char*)&TS_Matrix.Cn)[0]=*pmatrix++; 00162 ((unsigned char*)&TS_Matrix.Cn)[1]=*pmatrix++; 00163 ((unsigned char*)&TS_Matrix.Cn)[2]=*pmatrix++; 00164 ((unsigned char*)&TS_Matrix.Cn)[3]=*pmatrix++; 00165 ((unsigned char*)&TS_Matrix.Dn)[0]=*pmatrix++; 00166 ((unsigned char*)&TS_Matrix.Dn)[1]=*pmatrix++; 00167 ((unsigned char*)&TS_Matrix.Dn)[2]=*pmatrix++; 00168 ((unsigned char*)&TS_Matrix.Dn)[3]=*pmatrix++; 00169 ((unsigned char*)&TS_Matrix.En)[0]=*pmatrix++; 00170 ((unsigned char*)&TS_Matrix.En)[1]=*pmatrix++; 00171 ((unsigned char*)&TS_Matrix.En)[2]=*pmatrix++; 00172 ((unsigned char*)&TS_Matrix.En)[3]=*pmatrix++; 00173 ((unsigned char*)&TS_Matrix.Fn)[0]=*pmatrix++; 00174 ((unsigned char*)&TS_Matrix.Fn)[1]=*pmatrix++; 00175 ((unsigned char*)&TS_Matrix.Fn)[2]=*pmatrix++; 00176 ((unsigned char*)&TS_Matrix.Fn)[3]=*pmatrix++; 00177 ((unsigned char*)&TS_Matrix.Divider)[0]=*pmatrix++; 00178 ((unsigned char*)&TS_Matrix.Divider)[1]=*pmatrix++; 00179 ((unsigned char*)&TS_Matrix.Divider)[2]=*pmatrix++; 00180 ((unsigned char*)&TS_Matrix.Divider)[3]=*pmatrix++; 00181 00182 ts_val.calibration_done=1; 00183 00184 } 00185 00186 void TOUCHS::init( void) 00187 { 00188 _spi.format( 8, BUS_MODE); 00189 _spi.frequency( TS_SPEED); 00190 00191 } 00192 00193 00194 void TOUCHS::do_tap( void) 00195 { 00196 unsigned int i, x, y; 00197 00198 if ( TOUCHS::pressure() && ts_val.touched==0) { 00199 printf("press: %d\r\n", tap_debounce ); 00200 tap_debounce++; 00201 if ( tap_debounce>= cTS_DEBOUNCE) { 00202 tap_debounce=0; 00203 ts_val.touched=1; 00204 for ( i=4, x=0,y=0; i!=0; i--) { 00205 TOUCHS::read( &ts_val.coord); 00206 x+=ts_val.coord.x; 00207 y+=ts_val.coord.y; 00208 } 00209 ts_val.coord.x=x>>2; 00210 ts_val.coord.y=y>>2; 00211 // If the calibration process was successful complited... 00212 if ( ts_val.calibration_done) { 00213 // ...run the convertion from touch to screen coord. 00214 TOUCHS::calibrate(); 00215 } 00216 } 00217 } 00218 } 00219 00220 unsigned int TOUCHS::pressure( void) 00221 { 00222 unsigned char pressure; 00223 unsigned char iZ2, iZ1; 00224 00225 _spi.frequency( TS_SPEED); 00226 00227 _cs = 0; /* Seleziono il CS del TS */ 00228 00229 _spi.write( 0xB0); 00230 iZ1 = _spi.write( 0x00); 00231 // 00232 _spi.write( 0xC0); 00233 iZ2 = 127-_spi.write( 0x00); 00234 _cs = 1; /* De-Seleziono il CS del TS */ 00235 00236 pressure=iZ1+iZ2; 00237 00238 if ( pressure > 3) { 00239 // ts_val.touched=1; 00240 return( 1); 00241 } else { 00242 // ts_val.touched=0; 00243 return( 0); 00244 } 00245 00246 } 00247 00248 /* Read the value from the touch. Write the raw value inside the struct TS_COORD. */ 00249 void TOUCHS::read( _TS_COORD *ts) 00250 { 00251 unsigned int i, idx; 00252 unsigned int iZ1, iZ2; 00253 00254 _spi.frequency( TS_SPEED); 00255 00256 idx=0; 00257 while( 1) { 00258 // 00259 i=0; 00260 _cs = 0; /* Assert the CS */ 00261 00262 while ( i<cTS_CMDSIZE) { 00263 TSValue[i] = _spi.write( TSCmd[i]); 00264 /* */ 00265 i++; 00266 } 00267 00268 _cs = 1; /* Deasser the CS */ 00269 00270 /* */ 00271 ts->x = ((unsigned int)TSValue[15]<<5) | (TSValue[16]>>3); 00272 ts->y = ((unsigned int)TSValue[3]<<5) | (TSValue[4]>>3); 00273 iZ2 = ((unsigned int)TSValue[11]<<5) | (TSValue[12]>>3); 00274 iZ1 = ((unsigned int)TSValue[7]<<5) | (TSValue[8]>>3); 00275 ts->z = (int)(330.0 * (((double)ts->x)/4096.0)*((((double)iZ2)/((double)iZ1))-1.0)); 00276 00277 /* Reduce the value to 10bit */ 00278 ts->y = (ts->y >> MODE12); 00279 ts->x = (ts->x >> MODE12); 00280 00281 idx++; 00282 if ( ts->z < 10000 || idx>10) 00283 break; 00284 } 00285 } 00286
Generated on Fri Jul 15 2022 19:54:13 by
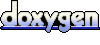