
Mbed Cloud Example Project - LPC546xx (Completed Version)
Fork of mbed-cloud-example-lpc546xx by
main.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "mbed.h" 00020 #include "mbed-trace/mbed_trace.h" 00021 #include "mbed-trace-helper.h" 00022 #include "simple-mbed-cloud-client.h" 00023 #include "key-config-manager/kcm_status.h" 00024 #include "key-config-manager/key_config_manager.h" 00025 #include "SDBlockDevice.h" 00026 #include "FATFileSystem.h" 00027 #include "EthernetInterface.h" 00028 00029 // Placeholder to hardware that trigger events (timer, button, etc) 00030 Ticker timer; 00031 InterruptIn sw2(SW2); 00032 DigitalOut led(LED1,1); 00033 00034 // Placeholder for storage 00035 SDBlockDevice sd(D11, D12, D13, D10); //MOSI, MISO, SCLK, CS 00036 FATFileSystem fs("sd"); 00037 00038 // To-Do #2: Add global pointer declaration for Rate Resource 00039 // Pointer declaration for Rate Resource 00040 static MbedCloudClientResource* rate_ptr; 00041 00042 static bool button_pressed = false; 00043 void button_press() { 00044 button_pressed = true; 00045 } 00046 00047 void led_toggle() { 00048 led = !led; 00049 } 00050 00051 // To-Do #3: Add Cloud Client Resource Callback Functions 00052 // Resource Callback Functions 00053 void blink_rate_updated(const char *) { 00054 printf("PUT received, Storing LED Blink Rate: %s (ms)\r\n", rate_ptr->get_value().c_str()); 00055 } 00056 00057 void blink_enable_callback(void *) { 00058 String pattern_str = rate_ptr->get_value(); 00059 const char *rate = pattern_str.c_str(); 00060 printf("POST received. Enabling LED Blink Rate = %s (ms)\n", rate); 00061 00062 float value = atoi(rate_ptr->get_value().c_str())/1000.0; 00063 timer.detach(); 00064 timer.attach(&led_toggle,value); 00065 } 00066 00067 void button_callback(const M2MBase& object, const NoticationDeliveryStatus status) 00068 { 00069 printf("Button notification. Callback: (%s)\n", object.uri_path()); 00070 } 00071 00072 00073 int main(void) 00074 { 00075 // Requires DAPLink 245+ (https://github.com/ARMmbed/DAPLink/pull/364) 00076 // Older versions: workaround to prevent possible deletion of credentials: 00077 wait(2); 00078 00079 // Misc OS setup 00080 srand(time(NULL)); 00081 00082 // Placeholder for network 00083 EthernetInterface net; 00084 00085 printf("Start Simple Mbed Cloud Client\n"); 00086 00087 // Initialize SD card 00088 int status = sd.init(); 00089 if (status != BD_ERROR_OK) { 00090 printf("Failed to init SD card\r\n"); 00091 return -1; 00092 } 00093 00094 // Mount the file system (reformatting on failure) 00095 status = fs.mount(&sd); 00096 if (status) { 00097 printf("Failed to mount FAT file system, reformatting...\r\n"); 00098 status = fs.reformat(&sd); 00099 if (status) { 00100 printf("Failed to reformat FAT file system\r\n"); 00101 return -1; 00102 } else { 00103 printf("Reformat and mount complete\r\n"); 00104 } 00105 } 00106 00107 // Connect to Network (obtain IP address) 00108 printf("Connecting to the network using Ethernet...\n"); 00109 status = net.connect(); 00110 if (status) { 00111 printf("Connection to Network Failed %d!\n", status); 00112 return -1; 00113 } else { 00114 const char *ip_addr = net.get_ip_address(); 00115 printf("Connected successfully\n"); 00116 printf("IP address %s\n", ip_addr); 00117 } 00118 00119 // Mbed Cloud Client Initialization 00120 SimpleMbedCloudClient mbedClient(&net); 00121 status = mbedClient.init(); 00122 if (status) { 00123 return -1; 00124 } 00125 printf("Client initialized\r\n"); 00126 00127 // To-Do #1: Add Mbed Cloud Client resources 00128 // Setup Mbed Cloud Client Resources 00129 MbedCloudClientResource *button = mbedClient.create_resource("3200/0/5501", "button_resource"); // Digital Input / Instance / Counter 00130 button->set_value("0"); 00131 button->methods(M2MMethod::GET); 00132 button->observable(true); 00133 button->attach_notification_callback(button_callback); 00134 00135 MbedCloudClientResource *rate = mbedClient.create_resource("3201/0/5521", "blink_rate_resource"); // Digital Output / Instance / Delay Duration 00136 rate->set_value("500"); 00137 rate->methods(M2MMethod::GET | M2MMethod::PUT); 00138 rate->observable(false); 00139 rate->attach_put_callback(blink_rate_updated); 00140 rate_ptr = rate; 00141 00142 MbedCloudClientResource *blink = mbedClient.create_resource("3201/0/5823", "blink_enable_resource"); // Digital Output / Instance / Event ID 00143 blink->methods(M2MMethod::POST); 00144 blink->attach_post_callback(blink_enable_callback); 00145 00146 // Mbed Cloud Register Client and Connect 00147 mbedClient.register_and_connect(); 00148 00149 // Wait for client to finish registering 00150 while (!mbedClient.is_client_registered()) { 00151 wait_ms(100); 00152 } 00153 00154 // Setup LED and Push BUtton 00155 timer.attach(&led_toggle, 0.5); 00156 sw2.fall(&button_press); 00157 00158 // Check if client is registering or registered, if true sleep and repeat. 00159 while (mbedClient.is_register_called()) { 00160 static int button_count = 0; 00161 wait_ms(100); 00162 00163 if (button_pressed) { 00164 button_pressed = false; 00165 printf("Button Pressed %d time(s).\r\n",(++button_count)); 00166 // To-Do #4: Add function to set button resource value 00167 // Call to update button resource count 00168 button->set_value(button_count); 00169 } 00170 } 00171 00172 // Client unregistered, exit program. 00173 return 0; 00174 }
Generated on Thu Jul 14 2022 00:55:18 by
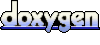