
mbed1
Dependencies: 4DGL SDFileSystem mbed-rtos mbed
Fork of drums by
note.cpp
00001 #include "mbed.h" 00002 #include "note.h" 00003 #include "TFT_4DGL.h" 00004 00005 #define RATIO 0.66 00006 #define minY 130 00007 #define maxY 430 00008 00009 // extern Serial pc; 00010 extern TFT_4DGL screen; 00011 00012 // Initate an empty note 00013 Note::Note() { 00014 // Do nothing 00015 } 00016 00017 // Initiate the note object with a certain type 00018 Note::Note(int t) { 00019 type = t; 00020 if (t == 1) { 00021 x = 276.0; 00022 color = 0xFF0000; 00023 } else if (t == 2) { 00024 x = 314.0; 00025 color = 0x00FF00; 00026 } else if (t == 3) { 00027 x = 358.0; 00028 color = 0x0000FF; 00029 } 00030 y = 130.0; 00031 w = 12.0; 00032 h = w * RATIO; 00033 speed = 38.0; 00034 consumed = 0; 00035 } 00036 00037 Note::~Note() { 00038 // deletes the node 00039 } 00040 00041 void Note::updatePosition() { 00042 y += speed; 00043 if (type == 1) { 00044 x += -0.14 * speed; 00045 } else if (type == 3) { 00046 x += 0.14 * speed; 00047 } 00048 w = 12.0 * (1 + (y - 130.0) / 300.0); 00049 h = w * RATIO; 00050 } 00051 00052 void Note::drawNote() { 00053 float oldX = x; 00054 float oldY = y; 00055 float oldW = w; 00056 float oldH = h; 00057 updatePosition(); 00058 screen.ellipse((int)oldX, (int)oldY, (int)oldW, (int)oldH, 0xD38A41); 00059 if (y > 420) { 00060 consumed = 1; 00061 // delete this; 00062 return; 00063 } 00064 screen.ellipse((int)x, (int)y, (int)w, (int)h, color); 00065 }
Generated on Thu Jul 14 2022 05:04:17 by
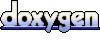