
Code for the first mbed for the drum hero project
Dependencies: 4DGL SDFileSystem mbed
Fork of drums2 by
main.cpp
00001 #include "mbed.h" 00002 #include "TFT_4DGL.h" 00003 #include "note.h" 00004 #include "song.h" 00005 #include <vector> 00006 00007 // Define all the ports 00008 // Serial pc(USBTX, USBRX); 00009 TFT_4DGL screen(p9,p10,p11); // serial tx, serial rx, reset pin; 00010 AnalogIn drum1(p18); 00011 AnalogIn drum2(p19); 00012 AnalogIn drum3(p20); 00013 DigitalOut musicOn(p22); 00014 DigitalIn button(p21); 00015 00016 // Define objects 00017 Ticker t1; 00018 DigitalOut led1(LED1); 00019 DigitalOut led2(LED2); 00020 DigitalOut led3(LED3); 00021 00022 // Define and initiate global variables 00023 std::vector<Note> noteArr; 00024 int beatNumber = 0; 00025 char buffer[2]; 00026 int skipped = 0; 00027 int temp; 00028 int score = 0; 00029 Song s = Song(1); 00030 00031 // Define the drum variables 00032 volatile int r1; 00033 volatile int r2; 00034 volatile int r3; 00035 volatile int r11; 00036 volatile int r22; 00037 volatile int r33; 00038 00039 // Function that draws the initial game frame 00040 void initiateScreen() { 00041 // Initiate the screen and the background 00042 screen.baudrate(3000000); 00043 screen.display_control(0x0c, 0x01); 00044 screen.background_color(0x000000); 00045 00046 // Draw the frame 00047 screen.rectangle(192, 120, 447, 450, 0xD38A41); 00048 screen.line(256, 120, 192, 450, 0x96411F); 00049 screen.line(296, 120, 277, 450, 0x96411F); 00050 screen.line(332, 120, 362, 450, 0x96411F); 00051 screen.line(372, 120, 447, 450, 0x96411F); 00052 screen.triangle(192, 120, 192, 450, 256, 120, 0x000000); 00053 screen.triangle(372, 120, 447, 450, 447, 120, 0x000000); 00054 screen.rectangle(447, 120, 450, 450, 0x000000); 00055 00056 screen.rectangle(256, 100, 296, 120, 0xFF0000); 00057 screen.rectangle(296, 100, 332, 120, 0x00FF00); 00058 screen.rectangle(332, 100, 372, 120, 0x0000FF); 00059 00060 // Define the points 00061 screen.graphic_string("POINTS:", 30, 100, FONT_8X8, WHITE, 2, 2); 00062 00063 screen.graphic_string("OB-LA-DI", 430, 100, FONT_8X8, WHITE, 2, 2); 00064 } 00065 00066 // The function that the ticker will be running, it checks if the piezo sensors 00067 // sense a hit two cycles (0.002 sec) in a row. Checking for two consecutive 00068 // high values discards the noise in the piezo sensors. 00069 void checkDrums() { 00070 if (drum1 == 1) { 00071 if (r1 == 1) { 00072 r11 = 1; 00073 } else { 00074 r1 = 1; 00075 }; 00076 } else if (drum2 == 1) { 00077 if (r2 == 1) { 00078 r22 = 1; 00079 } else { 00080 r2 = 1; 00081 }; 00082 } else if (drum3 == 1) { 00083 if (r3 == 1) { 00084 r33 = 1; 00085 } else { 00086 r3 = 1; 00087 }; 00088 } 00089 } 00090 00091 // Main methods that runs the main loop that checks the drums hits and draws the 00092 // next set of notes on the board. 00093 int main() { 00094 button.mode(PullDown); 00095 screen.text_string("DRUM HERO 1.0", 15, 8, FONT_8X8, WHITE); 00096 screen.text_string("PRESS THE BUTTON", 14, 16, FONT_8X8, WHITE); 00097 screen.text_string("TO START", 18, 19, FONT_8X8, WHITE); 00098 00099 musicOn = 0; 00100 while(!musicOn) { 00101 if(button) { 00102 musicOn = 1; 00103 } 00104 } 00105 00106 // Initiate the screen and the main graphics 00107 initiateScreen(); 00108 00109 t1.attach(&checkDrums, 0.001); 00110 00111 while (true) { 00112 // Check if the song is ended 00113 if (beatNumber < s.length) { 00114 // Check if the drum ticker sensed any hits 00115 if (r11) { 00116 // Draw hit drums for user feedback 00117 screen.rectangle(192,450,277,470,0xFF0000); 00118 led1 = 1; 00119 // Notes move down 5 times, check the note array in the index of 00120 // 5 notes before and see if it matches with the right drum 00121 if (s.notes[beatNumber - 5] == 1) { 00122 score++; 00123 } 00124 r1 = 0; 00125 r11 = 0; 00126 } else if (r22) { 00127 screen.rectangle(277,450,362,470,0x00FF00); 00128 led2 = 1; 00129 if (s.notes[beatNumber - 5] == 2) { 00130 score++; 00131 } 00132 r2 = 0; 00133 r22 = 0; 00134 } else if (r33) { 00135 screen.rectangle(362,450,447,470,0x0000FF); 00136 led3 = 1; 00137 if (s.notes[beatNumber - 5] == 3) { 00138 score++; 00139 } 00140 r3 = 0; 00141 r33 = 0; 00142 } else { 00143 screen.rectangle(192,450,447,470,0x000000); 00144 led1 = 0; 00145 led2 = 0; 00146 led3 = 0; 00147 } 00148 00149 // Read the next beat (0,1,2,3) 00150 temp = s.notes[beatNumber++]; 00151 // Check if there's a beat/note (not 0) 00152 if (temp) { 00153 // If beat/note exists, create the object for it and push 00154 // it to the array of current notes on the screen 00155 Note n1 = Note(temp); 00156 noteArr.push_back(n1); 00157 } 00158 00159 for (int i = skipped; i < noteArr.size(); i++) { 00160 if (noteArr[i].consumed) { 00161 skipped += 1; 00162 } else { 00163 noteArr[i].drawNote(); 00164 } 00165 } 00166 } else { 00167 // Game over screen 00168 screen.rectangle(0,0,640,480,0x000000); 00169 screen.graphic_string("GAME OVER", 240, 170, FONT_8X8, WHITE, 2, 2); 00170 screen.graphic_string("YOUR SCORE IS:", 210, 230, FONT_8X8, WHITE, 2, 2); 00171 screen.graphic_string(buffer, 290, 260, FONT_8X8, WHITE, 2, 2); 00172 wait(30); 00173 } 00174 // Update the score after each iteration 00175 sprintf(buffer,"%d",score); 00176 screen.graphic_string(buffer, 30, 120, FONT_8X8, WHITE, 2, 2); 00177 } 00178 }
Generated on Wed Jul 13 2022 12:26:10 by
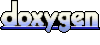