RGB-Led driver, used to handle convertions between HEX and integer to regulate LEDS through the embed.
Embed:
(wiki syntax)
Show/hide line numbers
color.h
00001 #ifndef COLOR_H 00002 #define COLOR_H 00003 /** Color class 00004 * A Color class to manage the colors, from HEX to RGB or int. 00005 */ 00006 class Color 00007 { 00008 public: 00009 /** enum of colors 00010 */ 00011 enum colors { 00012 RED= 0xFF0000, 00013 GREEN = 0x00FF00, 00014 BLUE = 0x0000FF, 00015 CYAN = 0x00FFFF, 00016 MAGENTA = 0xFF00FF, 00017 YELLOW = 0xFFFFFF, 00018 WHITE = 0xFFFFFF, 00019 PINK = 0xFF69B4 00020 }; 00021 /** Color instance with red, green, blue as integers 00022 */ 00023 Color(int red, int green, int blue); 00024 /** Color instance with an integer 00025 */ 00026 Color(int color); 00027 /** Color instance with red, green, blue as floats 00028 */ 00029 Color(float red, float green, float blue); 00030 /** getHex method 00031 *Returns the color as a Hex 00032 */ 00033 int getHex(); 00034 /** getRed method 00035 *Returns the color red as an integer 00036 */ 00037 int getRed(); 00038 /** getGreen method 00039 *Returns the color green as an integer 00040 */ 00041 int getGreen(); 00042 /** getBlue method 00043 *Returns the color blue as an integer 00044 */ 00045 int getBlue(); 00046 00047 private: 00048 /** Declaration int red 00049 */ 00050 int red; 00051 /** Declaration int blue 00052 */ 00053 int blue; 00054 /** Declaration int green 00055 */ 00056 int green; 00057 /** Declaration int color 00058 */ 00059 int color; 00060 /** Declaration method floatToColorValue(float value) 00061 returns an int 00062 */ 00063 int floatToColorValue(float value); 00064 /**Sets the maximum color value, 255 for RGB 00065 */ 00066 static const int MAX_COLOR_VALUE = 255; 00067 }; 00068 00069 #endif
Generated on Tue Jul 12 2022 16:48:34 by
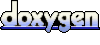