
Sistema de supervision y alarma para deposito de comida de animales
Embed:
(wiki syntax)
Show/hide line numbers
display.cpp
00001 //=====[Libraries]============================================================= 00002 00003 #include "mbed.h" 00004 #include "arm_book_lib.h" 00005 #include "display.h" 00006 00007 //=====[Declaration of private defines]======================================== 00008 00009 #define DISPLAY_IR_CLEAR_DISPLAY 0b00000001 00010 #define DISPLAY_IR_ENTRY_MODE_SET 0b00000100 00011 #define DISPLAY_IR_DISPLAY_CONTROL 0b00001000 00012 #define DISPLAY_IR_FUNCTION_SET 0b00100000 00013 #define DISPLAY_IR_SET_DDRAM_ADDR 0b10000000 00014 00015 #define DISPLAY_IR_ENTRY_MODE_SET_INCREMENT 0b00000010 00016 #define DISPLAY_IR_ENTRY_MODE_SET_DECREMENT 0b00000000 00017 #define DISPLAY_IR_ENTRY_MODE_SET_SHIFT 0b00000001 00018 #define DISPLAY_IR_ENTRY_MODE_SET_NO_SHIFT 0b00000000 00019 00020 #define DISPLAY_IR_DISPLAY_CONTROL_DISPLAY_ON 0b00000100 00021 #define DISPLAY_IR_DISPLAY_CONTROL_DISPLAY_OFF 0b00000000 00022 #define DISPLAY_IR_DISPLAY_CONTROL_CURSOR_ON 0b00000010 00023 #define DISPLAY_IR_DISPLAY_CONTROL_CURSOR_OFF 0b00000000 00024 #define DISPLAY_IR_DISPLAY_CONTROL_BLINK_ON 0b00000001 00025 #define DISPLAY_IR_DISPLAY_CONTROL_BLINK_OFF 0b00000000 00026 00027 #define DISPLAY_IR_FUNCTION_SET_8BITS 0b00010000 00028 #define DISPLAY_IR_FUNCTION_SET_4BITS 0b00000000 00029 #define DISPLAY_IR_FUNCTION_SET_2LINES 0b00001000 00030 #define DISPLAY_IR_FUNCTION_SET_1LINE 0b00000000 00031 #define DISPLAY_IR_FUNCTION_SET_5x10DOTS 0b00000100 00032 #define DISPLAY_IR_FUNCTION_SET_5x8DOTS 0b00000000 00033 00034 #define DISPLAY_20x4_LINE1_FIRST_CHARACTER_ADDRESS 0 00035 #define DISPLAY_20x4_LINE2_FIRST_CHARACTER_ADDRESS 64 00036 #define DISPLAY_20x4_LINE3_FIRST_CHARACTER_ADDRESS 20 00037 #define DISPLAY_20x4_LINE4_FIRST_CHARACTER_ADDRESS 84 00038 00039 #define DISPLAY_RS_INSTRUCTION 0 00040 #define DISPLAY_RS_DATA 1 00041 00042 #define DISPLAY_RW_WRITE 0 00043 #define DISPLAY_RW_READ 1 00044 00045 #define DISPLAY_PIN_RS 4 00046 #define DISPLAY_PIN_RW 5 00047 #define DISPLAY_PIN_EN 6 00048 #define DISPLAY_PIN_D0 7 00049 #define DISPLAY_PIN_D1 8 00050 #define DISPLAY_PIN_D2 9 00051 #define DISPLAY_PIN_D3 10 00052 #define DISPLAY_PIN_D4 11 00053 #define DISPLAY_PIN_D5 12 00054 #define DISPLAY_PIN_D6 13 00055 #define DISPLAY_PIN_D7 14 00056 00057 #define DISPLAY_PIN_A_PCF8574 3 00058 00059 #define I2C1_SDA PB_9 00060 #define I2C1_SCL PB_8 00061 00062 #define PCF8574_I2C_BUS_8BIT_WRITE_ADDRESS 78 00063 00064 //=====[Declaration of private data types]===================================== 00065 00066 typedef struct{ 00067 int address; 00068 char data; 00069 bool displayPin_RS; 00070 bool displayPin_RW; 00071 bool displayPin_EN; 00072 bool displayPin_A; 00073 bool displayPin_D4; 00074 bool displayPin_D5; 00075 bool displayPin_D6; 00076 bool displayPin_D7; 00077 } pcf8574_t; 00078 00079 //=====[Declaration and initialization of public global objects]=============== 00080 00081 DigitalOut displayD0( D0 ); 00082 DigitalOut displayD1( D1 ); 00083 DigitalOut displayD2( D2 ); 00084 DigitalOut displayD3( D3 ); 00085 DigitalOut displayD4( D4 ); 00086 DigitalOut displayD5( D5 ); 00087 DigitalOut displayD6( D6 ); 00088 DigitalOut displayD7( D7 ); 00089 DigitalOut displayRS( D8 ); 00090 DigitalOut displayEN( D9 ); 00091 00092 I2C I2C_PCF8574( I2C1_SDA, I2C1_SCL ); 00093 00094 //=====[Declaration of external public global variables]======================= 00095 00096 //=====[Declaration and initialization of public global variables]============= 00097 00098 //=====[Declaration and initialization of private global variables]============ 00099 00100 static display_t display; 00101 static pcf8574_t pcf8574; 00102 static bool initial8BitCommunicationIsCompleted; 00103 00104 //=====[Declarations (prototypes) of private functions]======================== 00105 00106 static void displayPinWrite( uint8_t pinName, int value ); 00107 static void displayDataBusWrite( uint8_t dataByte ); 00108 static void displayCodeWrite( bool type, uint8_t dataBus ); 00109 00110 //=====[Implementations of public functions]=================================== 00111 00112 void displayInit( displayConnection_t connection ) 00113 { 00114 display.connection = connection; 00115 00116 if( display.connection == DISPLAY_CONNECTION_I2C_PCF8574_IO_EXPANDER) { 00117 pcf8574.address = PCF8574_I2C_BUS_8BIT_WRITE_ADDRESS; 00118 pcf8574.data = 0b00000000; 00119 I2C_PCF8574.frequency(100000); 00120 displayPinWrite( DISPLAY_PIN_A_PCF8574, ON ); 00121 } 00122 00123 initial8BitCommunicationIsCompleted = FALSE; 00124 00125 delay( 50 ); 00126 00127 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00128 DISPLAY_IR_FUNCTION_SET | 00129 DISPLAY_IR_FUNCTION_SET_8BITS ); 00130 delay( 5 ); 00131 00132 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00133 DISPLAY_IR_FUNCTION_SET | 00134 DISPLAY_IR_FUNCTION_SET_8BITS ); 00135 delay( 1 ); 00136 00137 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00138 DISPLAY_IR_FUNCTION_SET | 00139 DISPLAY_IR_FUNCTION_SET_8BITS ); 00140 delay( 1 ); 00141 00142 switch( display.connection ) { 00143 case DISPLAY_CONNECTION_GPIO_8BITS: 00144 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00145 DISPLAY_IR_FUNCTION_SET | 00146 DISPLAY_IR_FUNCTION_SET_8BITS | 00147 DISPLAY_IR_FUNCTION_SET_2LINES | 00148 DISPLAY_IR_FUNCTION_SET_5x8DOTS ); 00149 delay( 1 ); 00150 break; 00151 00152 case DISPLAY_CONNECTION_GPIO_4BITS: 00153 case DISPLAY_CONNECTION_I2C_PCF8574_IO_EXPANDER: 00154 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00155 DISPLAY_IR_FUNCTION_SET | 00156 DISPLAY_IR_FUNCTION_SET_4BITS ); 00157 delay( 1 ); 00158 00159 initial8BitCommunicationIsCompleted = TRUE; 00160 00161 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00162 DISPLAY_IR_FUNCTION_SET | 00163 DISPLAY_IR_FUNCTION_SET_4BITS | 00164 DISPLAY_IR_FUNCTION_SET_2LINES | 00165 DISPLAY_IR_FUNCTION_SET_5x8DOTS ); 00166 delay( 1 ); 00167 break; 00168 } 00169 00170 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00171 DISPLAY_IR_DISPLAY_CONTROL | 00172 DISPLAY_IR_DISPLAY_CONTROL_DISPLAY_OFF | 00173 DISPLAY_IR_DISPLAY_CONTROL_CURSOR_OFF | 00174 DISPLAY_IR_DISPLAY_CONTROL_BLINK_OFF ); 00175 delay( 1 ); 00176 00177 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00178 DISPLAY_IR_CLEAR_DISPLAY ); 00179 delay( 1 ); 00180 00181 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00182 DISPLAY_IR_ENTRY_MODE_SET | 00183 DISPLAY_IR_ENTRY_MODE_SET_INCREMENT | 00184 DISPLAY_IR_ENTRY_MODE_SET_NO_SHIFT ); 00185 delay( 1 ); 00186 00187 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00188 DISPLAY_IR_DISPLAY_CONTROL | 00189 DISPLAY_IR_DISPLAY_CONTROL_DISPLAY_ON | 00190 DISPLAY_IR_DISPLAY_CONTROL_CURSOR_OFF | 00191 DISPLAY_IR_DISPLAY_CONTROL_BLINK_OFF ); 00192 delay( 1 ); 00193 } 00194 00195 void displayCharPositionWrite( uint8_t charPositionX, uint8_t charPositionY ) 00196 { 00197 switch( charPositionY ) { 00198 case 0: 00199 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00200 DISPLAY_IR_SET_DDRAM_ADDR | 00201 ( DISPLAY_20x4_LINE1_FIRST_CHARACTER_ADDRESS + 00202 charPositionX ) ); 00203 delay( 1 ); 00204 break; 00205 00206 case 1: 00207 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00208 DISPLAY_IR_SET_DDRAM_ADDR | 00209 ( DISPLAY_20x4_LINE2_FIRST_CHARACTER_ADDRESS + 00210 charPositionX ) ); 00211 delay( 1 ); 00212 break; 00213 00214 case 2: 00215 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00216 DISPLAY_IR_SET_DDRAM_ADDR | 00217 ( DISPLAY_20x4_LINE3_FIRST_CHARACTER_ADDRESS + 00218 charPositionX ) ); 00219 delay( 1 ); 00220 break; 00221 00222 case 3: 00223 displayCodeWrite( DISPLAY_RS_INSTRUCTION, 00224 DISPLAY_IR_SET_DDRAM_ADDR | 00225 ( DISPLAY_20x4_LINE4_FIRST_CHARACTER_ADDRESS + 00226 charPositionX ) ); 00227 delay( 1 ); 00228 break; 00229 } 00230 } 00231 00232 void displayStringWrite( char const * str ) 00233 { 00234 while (*str) { 00235 displayCodeWrite(DISPLAY_RS_DATA, *str++); 00236 } 00237 } 00238 00239 //=====[Implementations of private functions]================================== 00240 00241 static void displayCodeWrite( bool type, uint8_t dataBus ) 00242 { 00243 if ( type == DISPLAY_RS_INSTRUCTION ) 00244 displayPinWrite( DISPLAY_PIN_RS, DISPLAY_RS_INSTRUCTION); 00245 else 00246 displayPinWrite( DISPLAY_PIN_RS, DISPLAY_RS_DATA); 00247 displayPinWrite( DISPLAY_PIN_RW, DISPLAY_RW_WRITE ); 00248 displayDataBusWrite( dataBus ); 00249 } 00250 00251 static void displayPinWrite( uint8_t pinName, int value ) 00252 { 00253 switch( display.connection ) { 00254 case DISPLAY_CONNECTION_GPIO_8BITS: 00255 switch( pinName ) { 00256 case DISPLAY_PIN_D0: displayD0 = value; break; 00257 case DISPLAY_PIN_D1: displayD1 = value; break; 00258 case DISPLAY_PIN_D2: displayD2 = value; break; 00259 case DISPLAY_PIN_D3: displayD3 = value; break; 00260 case DISPLAY_PIN_D4: displayD4 = value; break; 00261 case DISPLAY_PIN_D5: displayD5 = value; break; 00262 case DISPLAY_PIN_D6: displayD6 = value; break; 00263 case DISPLAY_PIN_D7: displayD7 = value; break; 00264 case DISPLAY_PIN_RS: displayRS = value; break; 00265 case DISPLAY_PIN_EN: displayEN = value; break; 00266 case DISPLAY_PIN_RW: break; 00267 default: break; 00268 } 00269 case DISPLAY_CONNECTION_GPIO_4BITS: 00270 switch( pinName ) { 00271 case DISPLAY_PIN_D4: displayD4 = value; break; 00272 case DISPLAY_PIN_D5: displayD5 = value; break; 00273 case DISPLAY_PIN_D6: displayD6 = value; break; 00274 case DISPLAY_PIN_D7: displayD7 = value; break; 00275 case DISPLAY_PIN_RS: displayRS = value; break; 00276 case DISPLAY_PIN_EN: displayEN = value; break; 00277 case DISPLAY_PIN_RW: break; 00278 default: break; 00279 } 00280 break; 00281 00282 case DISPLAY_CONNECTION_I2C_PCF8574_IO_EXPANDER: 00283 if ( value ) { 00284 switch( pinName ) { 00285 case DISPLAY_PIN_D4: pcf8574.displayPin_D4 = ON; break; 00286 case DISPLAY_PIN_D5: pcf8574.displayPin_D5 = ON; break; 00287 case DISPLAY_PIN_D6: pcf8574.displayPin_D6 = ON; break; 00288 case DISPLAY_PIN_D7: pcf8574.displayPin_D7 = ON; break; 00289 case DISPLAY_PIN_RS: pcf8574.displayPin_RS = ON; break; 00290 case DISPLAY_PIN_EN: pcf8574.displayPin_EN = ON; break; 00291 case DISPLAY_PIN_RW: pcf8574.displayPin_RW = ON; break; 00292 case DISPLAY_PIN_A_PCF8574: pcf8574.displayPin_A = ON; break; 00293 default: break; 00294 } 00295 } 00296 else { 00297 switch( pinName ) { 00298 case DISPLAY_PIN_D4: pcf8574.displayPin_D4 = OFF; break; 00299 case DISPLAY_PIN_D5: pcf8574.displayPin_D5 = OFF; break; 00300 case DISPLAY_PIN_D6: pcf8574.displayPin_D6 = OFF; break; 00301 case DISPLAY_PIN_D7: pcf8574.displayPin_D7 = OFF; break; 00302 case DISPLAY_PIN_RS: pcf8574.displayPin_RS = OFF; break; 00303 case DISPLAY_PIN_EN: pcf8574.displayPin_EN = OFF; break; 00304 case DISPLAY_PIN_RW: pcf8574.displayPin_RW = OFF; break; 00305 case DISPLAY_PIN_A_PCF8574: pcf8574.displayPin_A = OFF; break; 00306 default: break; 00307 } 00308 } 00309 pcf8574.data = 0b00000000; 00310 if ( pcf8574.displayPin_RS ) pcf8574.data |= 0b00000001; 00311 if ( pcf8574.displayPin_RW ) pcf8574.data |= 0b00000010; 00312 if ( pcf8574.displayPin_EN ) pcf8574.data |= 0b00000100; 00313 if ( pcf8574.displayPin_A ) pcf8574.data |= 0b00001000; 00314 if ( pcf8574.displayPin_D4 ) pcf8574.data |= 0b00010000; 00315 if ( pcf8574.displayPin_D5 ) pcf8574.data |= 0b00100000; 00316 if ( pcf8574.displayPin_D6 ) pcf8574.data |= 0b01000000; 00317 if ( pcf8574.displayPin_D7 ) pcf8574.data |= 0b10000000; 00318 I2C_PCF8574.write( pcf8574.address, &pcf8574.data, 1); 00319 break; 00320 } 00321 } 00322 00323 static void displayDataBusWrite( uint8_t dataBus ) 00324 { 00325 displayPinWrite( DISPLAY_PIN_EN, OFF ); 00326 displayPinWrite( DISPLAY_PIN_D7, dataBus & 0b10000000 ); 00327 displayPinWrite( DISPLAY_PIN_D6, dataBus & 0b01000000 ); 00328 displayPinWrite( DISPLAY_PIN_D5, dataBus & 0b00100000 ); 00329 displayPinWrite( DISPLAY_PIN_D4, dataBus & 0b00010000 ); 00330 switch( display.connection ) { 00331 case DISPLAY_CONNECTION_GPIO_8BITS: 00332 displayPinWrite( DISPLAY_PIN_D3, dataBus & 0b00001000 ); 00333 displayPinWrite( DISPLAY_PIN_D2, dataBus & 0b00000100 ); 00334 displayPinWrite( DISPLAY_PIN_D1, dataBus & 0b00000010 ); 00335 displayPinWrite( DISPLAY_PIN_D0, dataBus & 0b00000001 ); 00336 break; 00337 00338 case DISPLAY_CONNECTION_GPIO_4BITS: 00339 case DISPLAY_CONNECTION_I2C_PCF8574_IO_EXPANDER: 00340 if ( initial8BitCommunicationIsCompleted == TRUE) { 00341 displayPinWrite( DISPLAY_PIN_EN, ON ); 00342 delay( 1 ); 00343 displayPinWrite( DISPLAY_PIN_EN, OFF ); 00344 delay( 1 ); 00345 displayPinWrite( DISPLAY_PIN_D7, dataBus & 0b00001000 ); 00346 displayPinWrite( DISPLAY_PIN_D6, dataBus & 0b00000100 ); 00347 displayPinWrite( DISPLAY_PIN_D5, dataBus & 0b00000010 ); 00348 displayPinWrite( DISPLAY_PIN_D4, dataBus & 0b00000001 ); 00349 } 00350 break; 00351 00352 } 00353 displayPinWrite( DISPLAY_PIN_EN, ON ); 00354 delay( 1 ); 00355 displayPinWrite( DISPLAY_PIN_EN, OFF ); 00356 delay( 1 ); 00357 }
Generated on Tue Jan 24 2023 09:13:28 by
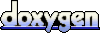