
Sistema de supervision y alarma para deposito de comida de animales
Embed:
(wiki syntax)
Show/hide line numbers
arm_book_lib.h
00001 /* Copyright 2020, Eric Pernia, Pablo Gomez and Ariel Lutemberg. 00002 * All rights reserved. 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions are met: 00006 * 00007 * 1. Redistributions of source code must retain the above copyright notice, 00008 * this list of conditions and the following disclaimer. 00009 * 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 00014 * 3. Neither the name of the copyright holder nor the names of its 00015 * contributors may be used to endorse or promote products derived from this 00016 * software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00019 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00020 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00021 * ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE 00022 * LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00023 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00024 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00025 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00026 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00027 * ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00028 * POSSIBILITY OF SUCH DAMAGE. 00029 */ 00030 00031 #ifndef _ARM_BOOK_LIBRARY_H_ 00032 #define _ARM_BOOK_LIBRARY_H_ 00033 00034 /*==================[inclusions]=============================================*/ 00035 00036 #include <cstdint> 00037 #include <mbed.h> 00038 00039 /*==================[c++]====================================================*/ 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /*==================[macros]=================================================*/ 00045 00046 // Functional states 00047 #ifndef OFF 00048 #define OFF 0 00049 #endif 00050 #ifndef ON 00051 #define ON (!OFF) 00052 #endif 00053 00054 // Electrical states 00055 #ifndef LOW 00056 #define LOW 0 00057 #endif 00058 #ifndef HIGH 00059 #define HIGH (!LOW) 00060 #endif 00061 00062 // Logical states 00063 00064 #ifndef FALSE 00065 #define FALSE 0 00066 #endif 00067 #ifndef TRUE 00068 #define TRUE (!FALSE) 00069 #endif 00070 00071 #ifndef false 00072 #define false 0 00073 #endif 00074 #ifndef true 00075 #define true (!false) 00076 #endif 00077 00078 // __I Defines 'read only' permissions: volatile const 00079 // __O Defines 'write only' permissions: volatile 00080 // __IO Defines 'read / write' permissions: volatile 00081 00082 #define HW_REG_8_R(x) (*((__I uint8_t *)(x))) 00083 #define HW_REG_16_R(x) (*((__I uint16_t *)(x))) 00084 #define HW_REG_32_R(x) (*((__I uint32_t *)(x))) 00085 00086 #define HW_REG_8_W(x) (*((__O uint8_t *)(x))) 00087 #define HW_REG_16_W(x) (*((__O uint16_t *)(x))) 00088 #define HW_REG_32_W(x) (*((__O uint32_t *)(x))) 00089 00090 #define HW_REG_8_RW(x) (*((__IO uint8_t *)(x))) 00091 #define HW_REG_16_RW(x) (*((__IO uint16_t *)(x))) 00092 #define HW_REG_32_RW(x) (*((__IO uint32_t *)(x))) 00093 00094 // Example: 00095 // #define REG_NAME (HW_REG_32_RW(0x4544555)) 00096 00097 /*==================[Function-like macros]===================================*/ 00098 00099 #define delay(ms) thread_sleep_for( ms ) 00100 00101 /*==================[typedef]================================================*/ 00102 00103 // ST Zio connector namings 00104 00105 // CN7 00106 # define D16 PC_6 00107 # define D17 PB_15 00108 # define D18 PB_13 00109 # define D19 PB_12 00110 # define D20 PA_15 00111 # define D21 PC_7 00112 # define D22 PB_5 00113 # define D23 PB_3 00114 # define D24 PA_4 00115 # define D25 PB_4 00116 00117 // CN10 00118 # define D26 PB_6 00119 # define D27 PB_2 00120 # define D28 PD_13 00121 # define D29 PD_12 00122 # define D30 PD_11 00123 # define D31 PE_2 00124 # define D32 PA_0 00125 # define D33 PB_0 00126 # define D34 PE_0 00127 # define D35 PB_11 00128 # define D36 PB_10 00129 # define D37 PE_15 00130 # define D38 PE_14 00131 # define D39 PE_12 00132 # define D40 PE_10 00133 # define D41 PE_7 00134 # define D42 PE_8 00135 00136 // CN8 00137 # define D43 PC_8 00138 # define D44 PC_9 00139 # define D45 PC_10 00140 # define D46 PC_11 00141 # define D47 PC_12 00142 # define D48 PD_1 00143 # define D49 PG_2 00144 # define D50 PG_3 00145 00146 // CN9 00147 # define D51 PD_7 00148 # define D52 PD_6 00149 # define D53 PD_5 00150 # define D54 PD_4 00151 # define D55 PD_3 00152 # define D56 PE_2 00153 # define D57 PE_4 00154 # define D58 PE_5 00155 # define D59 PE_6 00156 # define D60 PE_3 00157 # define D61 PF_8 00158 # define D62 PF_7 00159 # define D63 PF_9 00160 # define D64 PG_1 00161 # define D65 PG_0 00162 # define D66 PD_1 00163 # define D67 PD_0 00164 # define D68 PF_0 00165 # define D69 PF_1 00166 # define D70 PF_2 00167 # define D71 PA_7 00168 # define D72 NC 00169 00170 /*==================[c++]====================================================*/ 00171 #ifdef __cplusplus 00172 } 00173 #endif 00174 00175 /*==================[end of file]============================================*/ 00176 #endif // _ARM_BOOK_LIBRARY_H_
Generated on Tue Jan 24 2023 09:13:28 by
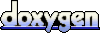