
Lab exercise 2.3 Interrupt
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "C12832.h" 00003 00004 InterruptIn joystickcenter(p14); 00005 InterruptIn button(p9); 00006 DigitalOut led(LED1); 00007 DigitalOut flash(LED4); 00008 C12832 lcd(p5, p7, p6, p8, p11); 00009 00010 00011 DigitalIn fire(p14); 00012 PwmOut spkr(p26); 00013 AnalogIn pot1(p19); 00014 00015 00016 00017 int x = 0; 00018 Timer debounce; 00019 00020 void flip() { 00021 led = !led; // toggles the led when the joystick button is pressed. 00022 x = 1; 00023 lcd.printf("debounce = "+debounce.read_ms()); 00024 if(debounce.read_ms()==1) 00025 { 00026 lcd.printf("ms 150"); 00027 //assignment 00028 } 00029 debounce.reset(); 00030 } 00031 int main() { 00032 00033 joystickcenter.rise(&flip); // attach the function address to the rising edge 00034 button.mode(PullUp); // With this, no external pullup resistor needed 00035 button.rise(&flip); // attach the function address to the rising edge 00036 00037 while(1) { // wait around, interrupts will interrupt this! 00038 flash = !flash; // turns LED4 on if off, off if on 00039 //wait(0.25); // the instruction to wait for a quarter-second 00040 if(x==1) // check loop 00041 { 00042 //lcd.printf("Variable set"); 00043 x = 0; 00044 } 00045 00046 for (float i=2000.0; i<1000.0; i+=100) { 00047 spkr.period(1.0/i); 00048 spkr=0.5; 00049 wait(0.1); 00050 } 00051 spkr=0.0; 00052 while(pot1.read() < 0.0000005) {} // this uses the pot to control the program 00053 00054 00055 00056 00057 } 00058 } 00059 00060 00061 //What's needed is a global variable with its own name, for example: 00062 //int x; 00063 00064 //Now, you set x to 1 inside the flip() function. In main(), inside the infinite loop starting with while (1) { , you check whether x ==1 and if it is, you print to the LCD and set x to 0. 00065 00066 //(Be very careful about this. x=1 sets x to 1. x==1 compares x to 1.)
Generated on Thu Jul 28 2022 22:59:30 by
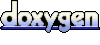