
A optical beam breaker detector that appears to aPC as a USB keyboard, typing characters when the beam is broken
usbdevice.h
00001 /* usbdevice.h */ 00002 /* Generic USB device */ 00003 /* Copyright (c) Phil Wright 2008 */ 00004 00005 #ifndef USBDEVICE_H 00006 #define USBDEVICE_H 00007 00008 #include "usbdc.h" 00009 00010 /* Endpoint packet sizes */ 00011 #define MAX_PACKET_SIZE_EP0 (64) 00012 00013 /* bmRequestType.dataTransferDirection */ 00014 #define HOST_TO_DEVICE (0) 00015 #define DEVICE_TO_HOST (1) 00016 00017 /* bmRequestType.Type*/ 00018 #define STANDARD_TYPE (0) 00019 #define CLASS_TYPE (1) 00020 #define VENDOR_TYPE (2) 00021 #define RESERVED_TYPE (3) 00022 00023 /* bmRequestType.Recipient */ 00024 #define DEVICE_RECIPIENT (0) 00025 #define INTERFACE_RECIPIENT (1) 00026 #define ENDPOINT_RECIPIENT (2) 00027 #define OTHER_RECIPIENT (3) 00028 00029 /* Descriptors */ 00030 #define DESCRIPTOR_TYPE(wValue) (wValue >> 8) 00031 #define DESCRIPTOR_INDEX(wValue) (wValue & 0xf) 00032 00033 /* Descriptor type */ 00034 #define DEVICE_DESCRIPTOR (1) 00035 #define CONFIGURATION_DESCRIPTOR (2) 00036 #define STRING_DESCRIPTOR (3) 00037 #define INTERFACE_DESCRIPTOR (4) 00038 #define ENDPOINT_DESCRIPTOR (5) 00039 00040 typedef struct { 00041 struct { 00042 unsigned char dataTransferDirection; 00043 unsigned char Type; 00044 unsigned char Recipient; 00045 } bmRequestType; 00046 unsigned char bRequest; 00047 unsigned short wValue; 00048 unsigned short wIndex; 00049 unsigned short wLength; 00050 } SETUP_PACKET; 00051 00052 typedef struct { 00053 SETUP_PACKET setup; 00054 unsigned char *ptr; 00055 unsigned long remaining; 00056 unsigned char direction; 00057 bool zlp; 00058 } CONTROL_TRANSFER; 00059 00060 typedef enum {ATTACHED, POWERED, DEFAULT, ADDRESS, CONFIGURED} DEVICE_STATE; 00061 00062 typedef struct { 00063 DEVICE_STATE state; 00064 unsigned char configuration; 00065 bool suspended; 00066 } USB_DEVICE; 00067 00068 class usbdevice : public usbdc 00069 { 00070 public: 00071 usbdevice(); 00072 protected: 00073 virtual void endpointEventEP0Setup(void); 00074 virtual void endpointEventEP0In(void); 00075 virtual void endpointEventEP0Out(void); 00076 virtual bool requestSetup(void); 00077 virtual bool requestOut(void); 00078 virtual void deviceEventReset(void); 00079 virtual bool requestGetDescriptor(void); 00080 bool requestSetAddress(void); 00081 virtual bool requestSetConfiguration(void); 00082 virtual bool requestGetConfiguration(void); 00083 bool requestGetStatus(void); 00084 virtual bool requestSetInterface(void); 00085 virtual bool requestGetInterface(void); 00086 bool requestSetFeature(void); 00087 bool requestClearFeature(void); 00088 CONTROL_TRANSFER transfer; 00089 USB_DEVICE device; 00090 private: 00091 bool controlIn(void); 00092 bool controlOut(void); 00093 bool controlSetup(void); 00094 void decodeSetupPacket(unsigned char *data, SETUP_PACKET *packet); 00095 }; 00096 00097 #endif
Generated on Tue Jul 12 2022 17:09:08 by
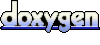