
Accepts RPC commands over bluetooth (RN42)
Embed:
(wiki syntax)
Show/hide line numbers
RPCFunction.h
00001 /** 00002 * @section LICENSE 00003 *Copyright (c) 2010 ARM Ltd. 00004 * 00005 *Permission is hereby granted, free of charge, to any person obtaining a copy 00006 *of this software and associated documentation files (the "Software"), to deal 00007 *in the Software without restriction, including without limitation the rights 00008 *to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 *copies of the Software, and to permit persons to whom the Software is 00010 *furnished to do so, subject to the following conditions: 00011 * 00012 *The above copyright notice and this permission notice shall be included in 00013 *all copies or substantial portions of the Software. 00014 * 00015 *THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 *IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 *FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 *AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 *LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 *OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 *THE SOFTWARE. 00022 * 00023 * @section Description 00024 *This class provides an object which can be called over RPC to run the function which is attached to it. 00025 * 00026 */ 00027 #ifndef RPCFUNCTION_RPC 00028 #define RPCFUNCTION_RPC 00029 /** 00030 *Includes 00031 */ 00032 #include "mbed.h" 00033 #include "platform.h" 00034 #include "rpc.h" 00035 #define STR_LEN 64 00036 #include "platform.h" 00037 00038 #ifdef MBED_RPC 00039 #include "rpc.h" 00040 #endif 00041 /** 00042 * 00043 *Class to call custom functions over RPC 00044 * 00045 */ 00046 class RPCFunction : public Base{ 00047 public: 00048 /** 00049 * Constructor 00050 * 00051 *@param f Pointer to the function to call. the function must be of the form void foo(char * input, char * output) 00052 *@param name The name of this object 00053 */ 00054 RPCFunction(void(*f)(char*, char*), const char* = NULL); 00055 00056 /** 00057 *run 00058 * 00059 *Calls the attached function passing the string in but doesn't return the result. 00060 *@param str The string to be passed into the attached function. This string can consist of any ASCII characters apart from escape codes. The usual limtations on argument content for RPC strings has been removed 00061 *@return A string output from the function 00062 */ 00063 char * run(char* str); 00064 00065 /** 00066 *Reads the value of the output string. 00067 * 00068 *@returns the string outputted from the last time the function was called 00069 */ 00070 char * read(); 00071 00072 00073 #ifdef MBED_RPC 00074 virtual const struct rpc_method *get_rpc_methods(); 00075 #endif 00076 00077 private: 00078 void (*_ftr)(char*, char*); 00079 00080 char _input[STR_LEN]; 00081 char _output[STR_LEN]; 00082 00083 }; 00084 #endif
Generated on Wed Jul 13 2022 06:00:45 by
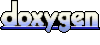