
Accepts RPC commands over bluetooth (RN42)
Embed:
(wiki syntax)
Show/hide line numbers
RPCFunction.cpp
00001 /** 00002 * @section LICENSE 00003 *Copyright (c) 2010 ARM Ltd. 00004 * 00005 *Permission is hereby granted, free of charge, to any person obtaining a copy 00006 *of this software and associated documentation files (the "Software"), to deal 00007 *in the Software without restriction, including without limitation the rights 00008 *to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 *copies of the Software, and to permit persons to whom the Software is 00010 *furnished to do so, subject to the following conditions: 00011 * 00012 *The above copyright notice and this permission notice shall be included in 00013 *all copies or substantial portions of the Software. 00014 * 00015 *THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 *IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 *FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 *AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 *LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 *OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 *THE SOFTWARE. 00022 * 00023 * @section Description 00024 *This class provides an object which can be called over RPC to run the function which is attached to it. 00025 * 00026 */ 00027 #include "RPCFunction.h" 00028 #include "rpc.h" 00029 00030 //Parse a char argument without delimiting by anything that is non alphanumeric - based on version in rpc.h line 153 00031 char *parse_arg_char(const char *arg, const char **next) { 00032 const char *ptr = arg; 00033 char *res = NULL; 00034 if(*arg == '"') { 00035 /* quoted string */ 00036 ptr = ++arg; 00037 int len = 0; 00038 /* find the end (and length) of the quoted string */ 00039 for(char c = *ptr; c != 0 && c != '"'; c = *++ptr) { 00040 len++; 00041 if(c == '\\') { 00042 ptr++; 00043 } 00044 } 00045 /* copy the quoted string, and unescape characters */ 00046 if(len != 0) { 00047 res = new char[len+1]; 00048 char *resptr = res; 00049 while(arg != ptr) { 00050 *resptr++ = parse_char(arg, &arg); 00051 } 00052 *resptr = 0; 00053 } 00054 } else { 00055 /* unquoted string */ 00056 while(isalnum(*ptr) || isgraph(*ptr) || *ptr=='_' || *ptr == ' ') { //Edit this line to change which types of characters are allowed and which delimit 00057 ptr++; 00058 } 00059 int len = ptr-arg; 00060 if(len!=0) { //Chnages made to just pass whole string with no next arg or delimiters, these changes just removes space at the beginning 00061 res = new char[len]; //was len+1 00062 memcpy(res, arg + 1, len - 1); // was arg, len 00063 res[len-1] = 0; //was len 00064 } 00065 } 00066 00067 if(next != NULL) { 00068 *next = ptr; 00069 } 00070 return res; 00071 } 00072 00073 //Custom rpc method caller for execute so that the string will not be delimited by anything 00074 //See line 436 of rpc.h 00075 void rpc_method_caller_run(Base *this_ptr, const char *arguments, char *result) { 00076 00077 const char *next = arguments; 00078 char* arg1 = parse_arg_char(next,NULL); 00079 00080 char * res = (static_cast<RPCFunction*>(this_ptr)->run)(arg1); 00081 if(result != NULL) { 00082 write_result<char*>(res, result); 00083 } 00084 } 00085 00086 RPCFunction::RPCFunction(void(*f)(char*, char*), const char* name) : Base(name){ 00087 _ftr = f; 00088 } 00089 00090 00091 //Just run the attached function using the string thats in private memory - or just using null values, 00092 char * RPCFunction::run(char * input){ 00093 strcpy(_input, input); 00094 (*_ftr)(_input,_output); 00095 return(_output); 00096 } 00097 00098 //Just read the output string 00099 char* RPCFunction::read(){ 00100 return(_output); 00101 } 00102 00103 00104 #ifdef MBED_RPC 00105 const rpc_method *RPCFunction::get_rpc_methods() { 00106 static const rpc_method rpc_methods[] = { 00107 { "run", rpc_method_caller_run }, //Run using custom caller, all characters accepted in string 00108 { "read", rpc_method_caller<char*, RPCFunction, &RPCFunction::read> }, 00109 RPC_METHOD_SUPER(Base) 00110 }; 00111 return rpc_methods; 00112 } 00113 00114 #endif
Generated on Wed Jul 13 2022 06:00:45 by
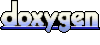