Embed:
(wiki syntax)
Show/hide line numbers
USBMouse.h
00001 /* USBMouse.h */ 00002 /* USB device example: relative mouse */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef _RELATIVE_MOUSE_ 00006 #define _RELATIVE_MOUSE_ 00007 00008 #include "GenericMouse.h" 00009 #include "USBHID.h" 00010 00011 /** USB device: a relative mouse 00012 * 00013 * Warning: you can only instantiate one instance of a USB device: USBMouse, USBKeyboard, USBAbsMouse, USBMouseKeyboard, or USBAbsMouseKeyboard. 00014 * 00015 * Example: 00016 * @code 00017 * #include "mbed.h" 00018 * #include "USBMouse.h" 00019 * 00020 * USBMouse mouse; 00021 * 00022 * #define STEP (2) 00023 * #define SIZE (100) 00024 * 00025 * int main(void) 00026 * { 00027 * int32_t a; 00028 * 00029 * while (1) 00030 * { 00031 * for (a=0; a<SIZE; a++) 00032 * { 00033 * mouse.move(STEP,0); 00034 * } 00035 * 00036 * for (a=0; a<SIZE; a++) 00037 * { 00038 * mouse.move(0,STEP); 00039 * } 00040 * 00041 * for (a=0; a<SIZE; a++) 00042 * { 00043 * mouse.move(-STEP,0); 00044 * } 00045 * 00046 * for (a=0; a<SIZE; a++) 00047 * { 00048 * mouse.move(0,-STEP); 00049 * } 00050 * } 00051 * } 00052 * 00053 * @endcode 00054 */ 00055 class USBMouse: public GenericMouse, public USBHID 00056 { 00057 public: 00058 00059 /** 00060 * Constructor for a USBMouse (relative mouse) 00061 */ 00062 USBMouse(){}; 00063 00064 /** 00065 * Write a state of the mouse 00066 * 00067 * @param x x relative position 00068 * @param y y relative position 00069 * @param buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) 00070 * @param z wheel state (>0 to scroll down, <0 to scroll up) 00071 * @return true if there is no error, false otherwise 00072 */ 00073 virtual bool update(int16_t x, int16_t y, uint8_t buttons, int8_t z); 00074 00075 virtual uint8_t * ReportDesc(); 00076 00077 private: 00078 bool mouseSend(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00079 }; 00080 00081 #endif
Generated on Fri Jul 15 2022 02:22:27 by
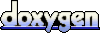