Embed:
(wiki syntax)
Show/hide line numbers
USBAbsMouse.c
00001 /* USBAbsMouse.c */ 00002 00003 /* USB device example: an absolute mouse */ 00004 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00005 00006 #include "stdint.h" 00007 00008 #include "USBAbsMouse.h" 00009 00010 /* 00011 * Descriptors 00012 */ 00013 00014 uint8_t * USBAbsMouse::ReportDesc() { 00015 static uint8_t reportDescriptor[] = { 00016 00017 /* Based on Appendix E.10 of "Device Class Definition for Human Interface 00018 Devices (HID)" Version 1.11 and modified to use absolute coordinates and a wheel. */ 00019 00020 USAGE_PAGE(1), 0x01, /* Generic Desktop */ 00021 USAGE(1), 0x02, /* Mouse */ 00022 COLLECTION(1), 0x01, /* Application*/ 00023 USAGE(1), 0x01, /* Pointer */ 00024 COLLECTION(1), 0x00, /* Physical */ 00025 00026 USAGE_PAGE(1), 0x01, /* Generic Desktop */ 00027 USAGE(1), 0x30, /* X */ 00028 USAGE(1), 0x31, /* Y */ 00029 LOGICAL_MINIMUM(1), 0x00, /* 0 */ 00030 LOGICAL_MAXIMUM(2), 0xff, 0x7f, /* 32767 */ 00031 REPORT_SIZE(1), 0x10, 00032 REPORT_COUNT(1), 0x02, 00033 INPUT(1), 0x02, /* Data, Variable, Absolute */ 00034 00035 USAGE_PAGE(1), 0x01, /* Generic Desktop */ 00036 USAGE(1), 0x38, /* scroll */ 00037 LOGICAL_MINIMUM(1), 0x81, /* -127 */ 00038 LOGICAL_MAXIMUM(1), 0x7f, /* 127 */ 00039 REPORT_SIZE(1), 0x08, 00040 REPORT_COUNT(1), 0x01, 00041 INPUT(1), 0x06, /* Data, Variable, Relative */ 00042 00043 USAGE_PAGE(1), 0x09, /* Buttons */ 00044 USAGE_MINIMUM(1), 0x01, 00045 USAGE_MAXIMUM(1), 0x03, 00046 LOGICAL_MINIMUM(1), 0x00, /* 0 */ 00047 LOGICAL_MAXIMUM(1), 0x01, /* 1 */ 00048 REPORT_COUNT(1), 0x03, 00049 REPORT_SIZE(1), 0x01, 00050 INPUT(1), 0x02, /* Data, Variable, Absolute */ 00051 REPORT_COUNT(1), 0x01, 00052 REPORT_SIZE(1), 0x05, 00053 INPUT(1), 0x01, /* Constant */ 00054 00055 END_COLLECTION(0), 00056 END_COLLECTION(0) 00057 }; 00058 reportLength = sizeof(reportDescriptor); 00059 return reportDescriptor; 00060 } 00061 00062 00063 bool USBAbsMouse::update(int16_t x, int16_t y, uint8_t buttons, int8_t z) { 00064 HID_REPORT report; 00065 00066 report.data[0] = x & 0xff; 00067 report.data[1] = (x >> 8) & 0xff; 00068 report.data[2] = y & 0xff; 00069 report.data[3] = (y >> 8) & 0xff; 00070 report.data[4] = -z; 00071 report.data[5] = buttons & 0x07; 00072 00073 report.length = 6; 00074 00075 return USBClass_HID_sendInputReport(EPINT_IN, &report); 00076 } 00077 00078
Generated on Fri Jul 15 2022 02:22:27 by
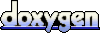