Embed:
(wiki syntax)
Show/hide line numbers
USBAbsMouseKeyboard.h
00001 /* USBAbsMouseKeyboard.h */ 00002 /* USB device example: keyboard and an absolute mouse */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef _USB_KEYBOARD_ABS_MOUSE_ 00006 #define _USB_KEYBOARD_ABS_MOUSE_ 00007 00008 #include "GenericMouse.h" 00009 #include "GenericKeyboard.h" 00010 #include "USBHID.h" 00011 00012 00013 /** USB device: a keyboard and an absolute mouse 00014 * 00015 * Warning: you can only instantiate one instance of a USB device: USBMouse, USBKeyboard, USBAbsMouse, USBMouseKeyboard, or USBAbsMouseKeyboard. 00016 * 00017 * Example: 00018 * @code 00019 * 00020 * #include "mbed.h" 00021 * #include "USBAbsMouseKeyboard.h" 00022 * 00023 * USBAbsMouseKeyboard key_mouse; 00024 * 00025 * #define STEP (1000) 00026 * 00027 * int main(void) 00028 * { 00029 * int32_t a = X_MIN; 00030 * int32_t dir = STEP; 00031 * 00032 * while (1) 00033 * { 00034 * key_mouse.move(a, a); 00035 * key_mouse.puts("Hello From Mbed\r\n"); 00036 * 00037 * if (((a+dir) > X_MAX) || ((a+dir) < X_MIN)) 00038 * { 00039 * // Change direction 00040 * dir = -dir; 00041 * } 00042 * a += dir; 00043 * 00044 * wait(1); 00045 * } 00046 * } 00047 * @endcode 00048 */ 00049 class USBAbsMouseKeyboard: public GenericMouse, public GenericKeyboard, public USBHID 00050 { 00051 public: 00052 00053 /** 00054 * Constructor for an absolute mouse and a keyboard 00055 */ 00056 USBAbsMouseKeyboard(){}; 00057 00058 /** 00059 * Write a state of the mouse 00060 * 00061 * @param x x absolute position 00062 * @param y y absolute position 00063 * @param buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) 00064 * @param z wheel state (>0 to scroll down, <0 to scroll up) 00065 * @return true if there is no error, false otherwise 00066 */ 00067 virtual bool update(int16_t x, int16_t y, uint8_t buttons, int8_t z); 00068 00069 virtual uint8_t * ReportDesc(); 00070 }; 00071 00072 #endif
Generated on Fri Jul 15 2022 02:22:27 by
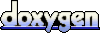