Embed:
(wiki syntax)
Show/hide line numbers
GenericKeyboard.h
00001 /* GenericKeyboard.h */ 00002 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00003 00004 #ifndef _GENERIC_KEYBOARD_ 00005 #define _GENERIC_KEYBOARD_ 00006 00007 #include "USBHID.h" 00008 #include "Stream.h" 00009 00010 #define REPORT_ID_KEYBOARD 1 00011 #define REPORT_ID_VOLUME 3 00012 00013 00014 ///All media keys 00015 enum MEDIA_KEY 00016 { 00017 KEY_NEXT_TRACK, ///< next Track Button 00018 KEY_PREVIOUS_TRACK, ///< Previous track Button 00019 KEY_STOP, ///< Stop Button 00020 KEY_PLAY_PAUSE, ///< Play/Pause Button 00021 KEY_MUTE, ///< Mute Button 00022 KEY_VOLUME_UP, ///< Volume Up Button 00023 KEY_VOLUME_DOWN, ///< Volume Down Button 00024 }; 00025 00026 00027 ///Different keys 00028 enum KEY 00029 { 00030 KEY_F1 = 128, ///< F1 key 00031 KEY_F2, ///< F2 key 00032 KEY_F3, ///< F3 key 00033 KEY_F4, ///< F4 key 00034 KEY_F5, ///< F5 key 00035 KEY_F6, ///< F6 key 00036 KEY_F7, ///< F7 key 00037 KEY_F8, ///< F8 key 00038 KEY_F9, ///< F9 key 00039 KEY_F10, ///< F10 key 00040 KEY_F11, ///< F11 key 00041 KEY_F12, ///< F12 key 00042 PRINT_SCREEN, ///< Print Screen key 00043 INSERT, ///< Insert key 00044 HOME, ///< Home key 00045 PAGE_UP, ///< Page Up key 00046 PAGE_DOWN, ///< Page Down key 00047 }; 00048 00049 00050 /** Generic keyboard 00051 * 00052 * This class is just an API to use in a child class. See USBKeyboard.h for instance for more information. 00053 * 00054 */ 00055 class GenericKeyboard: public Stream 00056 { 00057 public: 00058 /** 00059 * Constructor for a Generic keyboard 00060 */ 00061 GenericKeyboard(){}; 00062 00063 /** 00064 * Send all kinds of characters (ctrl + key, ...). 00065 * 00066 * @code 00067 * //To send CTRL + s (save) 00068 * keyboard.keyCode(CTRL, 's'); 00069 * @endcode 00070 * 00071 * @param modifier bit 0: CTRL, bit 1: SHIFT, bit 2: ALT 00072 * @param key character to send 00073 * @return true if there is no error, false otherwise 00074 */ 00075 bool keyCode(uint8_t modifier, uint8_t key); 00076 00077 /** 00078 * Send a character 00079 * 00080 * @param c character to be sent 00081 * @return true if there is no error, false otherwise 00082 */ 00083 virtual int _putc(int c); 00084 00085 /** 00086 * Control media keys 00087 * 00088 * @param key media key pressed (KEY_NEXT_TRACK, KEY_PREVIOUS_TRACK, KEY_STOP, KEY_PLAY_PAUSE, KEY_MUTE, KEY_VOLUME_UP, KEY_VOLUME_DOWN) 00089 * @return true if there is no error, false otherwise 00090 */ 00091 bool mediaControl(MEDIA_KEY key); 00092 00093 private: 00094 //dummy otherwise it doesn,t compile (we must define all methods of an abstract class) 00095 virtual int _getc() { return -1;} 00096 00097 00098 }; 00099 00100 #endif
Generated on Fri Jul 15 2022 02:22:27 by
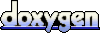