Embed:
(wiki syntax)
Show/hide line numbers
GenericKeyboard.c
00001 /* GenericKeyboard.c */ 00002 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00003 00004 #include "stdint.h" 00005 00006 #include "USBKeyboard.h" 00007 #include "GenericKeyboard.h" 00008 00009 00010 int GenericKeyboard::_putc(int c) { 00011 return keyCode(keymap[c].modifier, c); 00012 } 00013 00014 bool GenericKeyboard::keyCode(uint8_t modifier, uint8_t key) { 00015 /* Send a simulated keyboard keypress. Returns true if successful. */ 00016 00017 HID_REPORT report; 00018 00019 report.data[0] = REPORT_ID_KEYBOARD; 00020 report.data[1] = modifier; 00021 report.data[2] = 0; 00022 report.data[3] = keymap[key].usage; 00023 report.data[4] = 0; 00024 report.data[5] = 0; 00025 report.data[6] = 0; 00026 report.data[7] = 0; 00027 report.data[8] = 0; 00028 00029 report.length = 9; 00030 00031 if (!USBClass_HID_sendInputReport(EPINT_IN, &report)) { 00032 return false; 00033 } 00034 00035 report.data[1] = 0; 00036 report.data[3] = 0; 00037 00038 if (!USBClass_HID_sendInputReport(EPINT_IN, &report)) { 00039 return false; 00040 } 00041 00042 return true; 00043 00044 } 00045 00046 00047 bool GenericKeyboard::mediaControl(MEDIA_KEY key) { 00048 HID_REPORT report; 00049 00050 report.data[0] = REPORT_ID_VOLUME; 00051 report.data[1] = (1 << key) & 0x7f; 00052 00053 report.length = 2; 00054 00055 return USBClass_HID_sendInputReport(EPINT_IN, &report); 00056 }
Generated on Fri Jul 15 2022 02:22:27 by
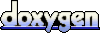