
An example program that uses a Wifly module to send messages over web sockets
Dependencies: WebSocketClient WiflyInterface mbed LM75B MMA7660
Fork of Websocket_Wifly_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "Websocket.h" 00004 #include "LM75B.h" 00005 #include "MMA7660.h" 00006 00007 00008 /* wifly interface: 00009 * - p9 and p10 are for the serial communication 00010 * - p30 is for the reset pin 00011 * - p29 is for the connection status 00012 * - "mbed" is the ssid of the network 00013 * - "password" is the password 00014 * - WPA is the security 00015 */ 00016 00017 WiflyInterface wifly(p9, p10, p30, p29, "mbed", "password", WPA); 00018 00019 // accelerometer 00020 MMA7660 acc(p28, p27); 00021 00022 // temperature sensor 00023 LM75B tmp(p28,p27); 00024 00025 00026 int main() 00027 { 00028 char json_str[100]; 00029 00030 wifly.init(); //Use DHCP 00031 while (!wifly.connect()); 00032 printf("IP Address is %s\n\r", wifly.getIPAddress()); 00033 00034 // See the output on http://sockets.mbed.org/app-board/viewer 00035 Websocket ws("ws://sockets.mbed.org:443/ws/app-board/wo"); 00036 00037 // connect WS server 00038 while (!ws.connect()); 00039 00040 while (1) { 00041 // create json string with acc/tmp data 00042 sprintf(json_str, "{\"id\":\"app_board_wifly_EW2013\",\"ax\":%d,\"ay\":%d,\"az\":%d, \"tmp\":%d}", (int)(acc.x()*360), (int)(acc.y()*360), (int)(acc.z()*360), (int)tmp.read()); 00043 00044 // send str 00045 ws.send(json_str); 00046 00047 wait(0.1); 00048 } 00049 }
Generated on Tue Jul 12 2022 12:11:02 by
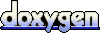