A simply library for the LM75B I2C temperature sensor
Dependents: LM75B-HelloWorld app-board-Sprint-SMS-LM75B SprintUSBModemWebsocketTest-Temp app-board-Ethernet-Websocket ... more
LM75B.h
00001 /* Copyright (c) 2012 cstyles, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef LM75B_H 00020 #define LM75B_H 00021 00022 #include "mbed.h" 00023 00024 // LM75B IIC address 00025 #define LM75B_ADDR 0x90 00026 00027 // LM75B registers 00028 #define LM75B_Conf 0x01 00029 #define LM75B_Temp 0x00 00030 #define LM75B_Tos 0x03 00031 #define LM75B_Thyst 0x02 00032 00033 //!Library for the LM75B temperature sensor. 00034 /*! 00035 The LM75B is an I2C digital temperature sensor, with a range of -55C to +125C and a 0.125C resolution. 00036 */ 00037 class LM75B 00038 { 00039 public: 00040 //!Creates an instance of the class. 00041 /*! 00042 Connect module at I2C address addr using I2C port pins sda and scl. 00043 LM75B 00044 */ 00045 LM75B(PinName sda, PinName scl); 00046 00047 /*! 00048 Destroys instance. 00049 */ 00050 ~LM75B (); 00051 00052 //!Reads the current temperature. 00053 /*! 00054 Reads the temperature register of the LM75B and converts it to a useable value. 00055 */ 00056 float read(); 00057 00058 private: 00059 00060 I2C i2c; 00061 00062 }; 00063 00064 #endif
Generated on Tue Jul 12 2022 14:46:37 by
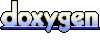