BLE_API
DiscoveredCharacteristic Class Reference
Representation of a characteristic discovered during a GattClient discovery procedure (see GattClient::launchServiceDiscovery ). More...
#include <DiscoveredCharacteristic.h>
Data Structures | |
struct | Properties_t |
Structure that encapsulates the properties of a discovered characteristic. More... | |
Public Member Functions | |
ble_error_t | read (uint16_t offset=0) const |
Initiate (or continue) a read for the value attribute, optionally at a given offset. | |
ble_error_t | read (uint16_t offset, const GattClient::ReadCallback_t &onRead) const |
Same as read(uint16_t) const but allow the user to register a callback which will be fired once the read is done. | |
ble_error_t | writeWoResponse (uint16_t length, const uint8_t *value) const |
Perform a write without response procedure. | |
ble_error_t | discoverDescriptors (const CharacteristicDescriptorDiscovery::DiscoveryCallback_t &onDescriptorDiscovered, const CharacteristicDescriptorDiscovery::TerminationCallback_t &onTermination) const |
Initiate a GATT Characteristic Descriptor Discovery procedure for descriptors within this characteristic. | |
ble_error_t | write (uint16_t length, const uint8_t *value) const |
Perform a write procedure. | |
ble_error_t | write (uint16_t length, const uint8_t *value, const GattClient::WriteCallback_t &onWrite) const |
Same as write(uint16_t, const uint8_t *) const but register a callback which will be called once the data has been written. | |
const UUID & | getUUID (void) const |
Get the UUID of the discovered characteristic. | |
const Properties_t & | getProperties (void) const |
Get the properties of this characteristic. | |
GattAttribute::Handle_t | getDeclHandle (void) const |
Get the declaration handle of this characteristic. | |
GattAttribute::Handle_t | getValueHandle (void) const |
Return the handle used to access the value of this characteristic. | |
GattAttribute::Handle_t | getLastHandle (void) const |
Return the last handle of the characteristic definition. | |
GattClient * | getGattClient () |
Return the GattClient which can operate on this characteristic. | |
const GattClient * | getGattClient () const |
Return the GattClient which can operate on this characteristic. | |
Gap::Handle_t | getConnectionHandle () const |
Return the connection handle to the GattServer which contain this characteristic. | |
Protected Attributes | |
GattClient * | gattc |
Pointer to the underlying GattClient for this DiscoveredCharacteristic object. | |
UUID | uuid |
Discovered characteristic's UUID. | |
Properties_t | props |
Hold the configured properties of the discovered characteristic. | |
GattAttribute::Handle_t | declHandle |
Value handle of the discovered characteristic's declaration attribute. | |
GattAttribute::Handle_t | valueHandle |
Value handle of the discovered characteristic's value attribute. | |
GattAttribute::Handle_t | lastHandle |
Value handle of the discovered characteristic's last attribute. | |
Gap::Handle_t | connHandle |
Handle for the connection where the characteristic was discovered. | |
Friends | |
bool | operator== (const DiscoveredCharacteristic &lhs, const DiscoveredCharacteristic &rhs) |
"Equal to" operator for DiscoveredCharacteristic | |
bool | operator!= (const DiscoveredCharacteristic &lhs, const DiscoveredCharacteristic &rhs) |
"Not equal to" operator for DiscoveredCharacteristic |
Detailed Description
Representation of a characteristic discovered during a GattClient discovery procedure (see GattClient::launchServiceDiscovery ).
Provide detailed informations about a discovered characteristic like:
- Its UUID (see getUUID()).
- The most important handles of the characteristic definition (see getDeclHandle(), getValueHandle(), getLastHandle())
- Its properties (see getProperties()). This class also provide functions to operate on the characteristic:
- Read the characteristic value (see read())
- Writing a characteristic value (see write() or writeWoResponse())
- Discover descriptors inside the characteristic definition. These descriptors extends the characteristic. More information about descriptor usage is available in DiscoveredCharacteristicDescriptor class.
Definition at line 43 of file DiscoveredCharacteristic.h.
Member Function Documentation
ble_error_t discoverDescriptors | ( | const CharacteristicDescriptorDiscovery::DiscoveryCallback_t & | onDescriptorDiscovered, |
const CharacteristicDescriptorDiscovery::TerminationCallback_t & | onTermination | ||
) | const |
Initiate a GATT Characteristic Descriptor Discovery procedure for descriptors within this characteristic.
- Parameters:
-
[in] onDescriptorDiscovered This callback will be called every time a descriptor is discovered [in] onTermination This callback will be called when the discovery process is over.
- Returns:
- BLE_ERROR_NONE if descriptor discovery is launched successfully; else an appropriate error.
Definition at line 154 of file DiscoveredCharacteristic.cpp.
Gap::Handle_t getConnectionHandle | ( | ) | const |
Return the connection handle to the GattServer which contain this characteristic.
- Returns:
- the connection handle to the GattServer which contain this characteristic.
Definition at line 349 of file DiscoveredCharacteristic.h.
GattAttribute::Handle_t getDeclHandle | ( | void | ) | const |
Get the declaration handle of this characteristic.
The declaration handle is the first handle of a characteristic definition. The value accessible at this handle contains the following informations:
- The characteristics properties (see Properties_t). This value can be accessed by using getProperties .
- The characteristic value attribute handle. This field can be accessed by using getValueHandle .
- The characteristic UUID, this value can be accessed by using the function getUUID .
- Returns:
- the declaration handle of this characteristic.
Definition at line 298 of file DiscoveredCharacteristic.h.
const GattClient* getGattClient | ( | ) | const |
Return the GattClient which can operate on this characteristic.
- Returns:
- The GattClient which can operate on this characteristic.
Definition at line 339 of file DiscoveredCharacteristic.h.
GattClient* getGattClient | ( | ) |
Return the GattClient which can operate on this characteristic.
- Returns:
- The GattClient which can operate on this characteristic.
Definition at line 331 of file DiscoveredCharacteristic.h.
GattAttribute::Handle_t getLastHandle | ( | void | ) | const |
Return the last handle of the characteristic definition.
A Characteristic definition can contain a lot of handles:
- one for the declaration (see getDeclHandle)
- one for the value (see getValueHandle)
- zero of more for the characteristic descriptors. This handle is the last handle of the characteristic definition.
- Returns:
- The last handle of this characteristic definition.
Definition at line 323 of file DiscoveredCharacteristic.h.
const Properties_t& getProperties | ( | void | ) | const |
Get the properties of this characteristic.
- Returns:
- the set of properties of this characteristic
Definition at line 281 of file DiscoveredCharacteristic.h.
const UUID& getUUID | ( | void | ) | const |
Get the UUID of the discovered characteristic.
- Returns:
- the UUID of this characteristic
Definition at line 273 of file DiscoveredCharacteristic.h.
GattAttribute::Handle_t getValueHandle | ( | void | ) | const |
Return the handle used to access the value of this characteristic.
This handle is the one provided in the characteristic declaration value. Usually, it is equal to getDeclHandle() + 1. But it is not always the case. Anyway, users are allowed to use getDeclHandle() + 1 to access the value of a characteristic.
- Returns:
- The handle to access the value of this characteristic.
Definition at line 310 of file DiscoveredCharacteristic.h.
ble_error_t read | ( | uint16_t | offset, |
const GattClient::ReadCallback_t & | onRead | ||
) | const |
Same as read(uint16_t) const but allow the user to register a callback which will be fired once the read is done.
- Parameters:
-
[in] offset The position - in the characteristic value bytes stream - where the read operation begin. [in] onRead Continuation of the read operation
Definition at line 69 of file DiscoveredCharacteristic.cpp.
ble_error_t read | ( | uint16_t | offset = 0 ) |
const |
Initiate (or continue) a read for the value attribute, optionally at a given offset.
If the characteristic or descriptor to be read is longer than ATT_MTU - 1, this function must be called multiple times with appropriate offset to read the complete value.
- Parameters:
-
[in] offset The position - in the characteristic value bytes stream - where the read operation begin.
- Returns:
- BLE_ERROR_NONE if a read has been initiated, or BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or BLE_STACK_BUSY if some client procedure is already in progress, or BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties.
Definition at line 21 of file DiscoveredCharacteristic.cpp.
ble_error_t write | ( | uint16_t | length, |
const uint8_t * | value | ||
) | const |
Perform a write procedure.
- Parameters:
-
[in] length The amount of data being written. [in] value The bytes being written.
- Note:
- It is important to note that a write will generate an onDataWritten() callback when the peer acknowledges the request.
- Return values:
-
BLE_ERROR_NONE Successfully started the Write procedure, or BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or BLE_STACK_BUSY if some client procedure is already in progress, or BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties.
Definition at line 81 of file DiscoveredCharacteristic.cpp.
ble_error_t write | ( | uint16_t | length, |
const uint8_t * | value, | ||
const GattClient::WriteCallback_t & | onWrite | ||
) | const |
Same as write(uint16_t, const uint8_t *) const but register a callback which will be called once the data has been written.
- Parameters:
-
[in] length The amount of bytes to write. [in] value The bytes to write. [in] onWrite Continuation callback for the write operation
- Return values:
-
BLE_ERROR_NONE Successfully started the Write procedure, or BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or BLE_STACK_BUSY if some client procedure is already in progress, or BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties.
Definition at line 143 of file DiscoveredCharacteristic.cpp.
ble_error_t writeWoResponse | ( | uint16_t | length, |
const uint8_t * | value | ||
) | const |
Perform a write without response procedure.
- Parameters:
-
[in] length The amount of data being written. [in] value The bytes being written.
- Note:
- It is important to note that a write without response will generate an onDataSent() callback when the packet has been transmitted. There will be a BLE-stack specific limit to the number of pending writeWoResponse operations; the user may want to use the onDataSent() callback for flow-control.
- Return values:
-
BLE_ERROR_NONE Successfully started the Write procedure, or BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or BLE_STACK_BUSY if some client procedure is already in progress, or BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties.
Definition at line 95 of file DiscoveredCharacteristic.cpp.
Friends And Related Function Documentation
bool operator!= | ( | const DiscoveredCharacteristic & | lhs, |
const DiscoveredCharacteristic & | rhs | ||
) | [friend] |
"Not equal to" operator for DiscoveredCharacteristic
- Parameters:
-
[in] lhs The right hand side of the expression [in] rhs The left hand side of the expression
- Returns:
- true if operands are not equal, false otherwise.
Definition at line 383 of file DiscoveredCharacteristic.h.
bool operator== | ( | const DiscoveredCharacteristic & | lhs, |
const DiscoveredCharacteristic & | rhs | ||
) | [friend] |
"Equal to" operator for DiscoveredCharacteristic
- Parameters:
-
[in] lhs The left hand side of the equality expression [in] rhs The right hand side of the equality expression
- Returns:
- true if operands are equals, false otherwise.
Definition at line 363 of file DiscoveredCharacteristic.h.
Field Documentation
Gap::Handle_t connHandle [protected] |
Handle for the connection where the characteristic was discovered.
Definition at line 430 of file DiscoveredCharacteristic.h.
GattAttribute::Handle_t declHandle [protected] |
Value handle of the discovered characteristic's declaration attribute.
Definition at line 417 of file DiscoveredCharacteristic.h.
GattClient* gattc [protected] |
Pointer to the underlying GattClient for this DiscoveredCharacteristic object.
Definition at line 402 of file DiscoveredCharacteristic.h.
GattAttribute::Handle_t lastHandle [protected] |
Value handle of the discovered characteristic's last attribute.
Definition at line 425 of file DiscoveredCharacteristic.h.
Properties_t props [protected] |
Hold the configured properties of the discovered characteristic.
For more information refer to Properties_t.
Definition at line 413 of file DiscoveredCharacteristic.h.
Discovered characteristic's UUID.
Definition at line 408 of file DiscoveredCharacteristic.h.
GattAttribute::Handle_t valueHandle [protected] |
Value handle of the discovered characteristic's value attribute.
Definition at line 421 of file DiscoveredCharacteristic.h.
Generated on Thu Jul 14 2022 21:15:42 by
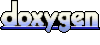