ble nano hid over gatt
Dependencies: BLE_API mbed-dev nRF51822
keymap.h
00001 #include "keyboard.h" 00002 #include "keyboard--short-names.h" 00003 00004 class Keymap; 00005 struct keyfunc_t { 00006 int8_t row; 00007 int8_t col; 00008 void (*func)(Keymap& keymap, bool pressed); 00009 }; 00010 00011 00012 static const uint8_t ROWS = 8; 00013 static const uint8_t COLS = 16; 00014 static const uint8_t LAYERS = 2; 00015 00016 class Keymap { 00017 static const uint8_t KEYMAP_DEFINITION[LAYERS][ROWS][COLS]; 00018 static const keyfunc_t KEYMAP_FUNCTIONS[]; 00019 00020 public: 00021 int8_t layer; 00022 00023 Keymap() : layer(0) { 00024 } 00025 00026 static void switch_layer(Keymap& keymap, const bool pressed) { 00027 if (pressed) { 00028 keymap.layer++; 00029 if (keymap.layer > LAYERS) { 00030 keymap.layer = LAYERS - 1; 00031 } 00032 } else { 00033 keymap.layer--; 00034 if (keymap.layer < 0) { 00035 keymap.layer = 0; 00036 } 00037 } 00038 DEBUG_PRINTF_KEYEVENT("LAYER->%d\r\n", keymap.layer); 00039 } 00040 00041 static void consumer_play_pause(Keymap& keymap, const bool pressed) { 00042 if (pressed) { 00043 HIDController::pressConsumerKey(0x00cd); 00044 } else { 00045 HIDController::releaseConsumerKey(); 00046 } 00047 } 00048 00049 void execute(const int row, const int col, const bool pressed) { 00050 for (int i = 0; ; i++) { 00051 const keyfunc_t& keyfunc = KEYMAP_FUNCTIONS[i]; 00052 if (keyfunc.row == -1) break; 00053 if (keyfunc.col == col && keyfunc.row == row) { 00054 DEBUG_PRINTF_KEYEVENT("& %d:%d\r\n", keyfunc.row, keyfunc.row); 00055 keyfunc.func(*this, pressed); 00056 return; 00057 } 00058 } 00059 00060 if (pressed) { 00061 uint8_t key = KEYMAP_DEFINITION[layer][row][col]; 00062 if (key) { 00063 DEBUG_PRINTF_KEYEVENT("D%d %x\r\n", layer, key); 00064 HIDController::appendReportData(key); 00065 } 00066 } else { 00067 // ensure delete all keys on layers 00068 for (int i = 0; i < LAYERS; i++) { 00069 uint8_t key = KEYMAP_DEFINITION[i][row][col]; 00070 DEBUG_PRINTF_KEYEVENT("U%d %x\r\n", layer, key); 00071 HIDController::deleteReportData(key); 00072 } 00073 } 00074 } 00075 }; 00076 00077 // unimplemented in hardware is __ 00078 #define __________ 0 00079 // unimplemented in firmware is _undef 00080 #define _undef 0 00081 00082 const uint8_t Keymap::KEYMAP_DEFINITION[LAYERS][ROWS][COLS] = { 00083 // layer 0 00084 { 00085 /* { 0 , 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 , 11 , 12 , 13 , 14 , 15 } */ 00086 /*0*/{ _esc , _F1 , _F2 , _F3 , _F4 , _F5 , _F6 , __________ , __________ , _F7 , _F8 , _F9 , _F10 , _F11 , _F12 , _undef } , 00087 /*1*/{ _esc , _1 , _2 , _3 , _4 , _5 , _6 , _7 , _6 , _7 , _8 , _9 , _0 , _dash , _equal , _backslash } , 00088 /*2*/{ _tab , _Q , _W , _E , _R , _T , _Y , __________ , _T , _Y , _U , _I , _O , _P , _bracketL , _grave } , 00089 /*3*/{ _ctrlL , _A , _S , _D , _F , _G , _H , __________ , _G , _H , _J , _K , _L , _semicolon , _quote , _bracketR } , 00090 /*4*/{ _shiftL , _Z , _X , _C , _V , _B , _N , __________ , _B , _N , _M , _comma , _period , _slash , _shiftR , _bs } , 00091 /*5*/{ _altL , _guiL , _space , __________ , __________ , _undef , __________ , __________ , __________ , _arrowU , _space , __________ , _guiR , _altR , _undef , _enter } , 00092 /*6*/{ __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , _arrowL , _arrowD , _arrowR , __________ , __________ , __________ , __________ , __________ } , 00093 /*7*/{ __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ } , 00094 }, 00095 // layer 1 00096 { 00097 /* { 0 , 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 , 11 , 12 , 13 , 14 , 15 } */ 00098 /*0*/{ _esc , _F1 , _F2 , _F3 , _F4 , _F5 , _F6 , __________ , __________ , _F7 , _F8 , _F9 , _F10 , _F11 , _F12 , _undef } , 00099 /*1*/{ _esc , _1 , _2 , _3 , _4 , _5 , _6 , _7 , _6 , _7 , _8 , _9 , _0 , _dash , _equal , _backslash } , 00100 /*2*/{ _tab , _Q , _W , _E , _R , _T , _Y , __________ , _T , _Y , _U , _I , _O , _P , _arrowU , _del } , 00101 /*3*/{ _ctrlL , _A , _S , _D , _F , _G , _H , __________ , _G , _H , _J , _K , _L , _arrowL , _arrowR , _bracketR } , 00102 /*4*/{ _shiftL , _Z , _X , _C , _V , _B , _N , __________ , _B , _N , _M , _comma , _period , _arrowD , _shiftR , _bs } , 00103 /*5*/{ _altL , _guiL , _space , __________ , __________ , _undef , __________ , __________ , __________ , _arrowU , _space , __________ , _guiR , _altR , _undef , _enter } , 00104 /*6*/{ __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , _arrowL , _arrowD , _arrowR , __________ , __________ , __________ , __________ , __________ } , 00105 /*7*/{ __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ , __________ } , 00106 }, 00107 }; 00108 00109 const keyfunc_t Keymap::KEYMAP_FUNCTIONS[] = { 00110 { 5, 14, &Keymap::switch_layer }, 00111 { 5, 5, &Keymap::consumer_play_pause }, 00112 { -1, -1, 0 } /* for iteration */ 00113 }; 00114 00115 #undef __________ 00116 #undef _undef 00117
Generated on Tue Jul 12 2022 14:16:48 by
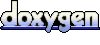