
WordClock-Program to display time in words on WS2812B-LED-Stripe. With DS3231 RTC
Dependencies: PixelArray WordClock_de ds3231 mbed
Fork of mbed_ws2812b by
main.cpp
00001 // Wordclock with WS2812-LED-Stripe 00002 // with 11x10 LED-Matrix and 4 minute-LEDS 00003 // and DS3231 RTC 00004 /* 00005 00006 ESKISTLFÜNF 00007 ZEHNZWANZIG 00008 DREIVIERTEL 00009 TGNACHVORJM 00010 HALBXZWÖLFP 00011 ZWEINSIEBEN 00012 KDREIRHFÜNF 00013 ELFNEUNVIER 00014 WACHTZEHNRS 00015 BSECHSFMUHR 00016 **** 00017 */ 00018 00019 00020 #include "mbed.h" 00021 #include "neopixel.h" 00022 #include "WordClock.h" 00023 #include "ds3231.h" 00024 00025 /** add simple DST info to nvram of ds3231 00026 * we abuse bit RS1(bit3) from control-register which normally controls frequency of output-pin 00027 */ 00028 class Ds3231_dst : public Ds3231 00029 { 00030 public: 00031 Ds3231_dst(PinName sda, PinName scl) : Ds3231(sda,scl) {} 00032 00033 /** set_DST(bool dst) 00034 */ 00035 uint16_t set_DST(bool dst) { 00036 ds3231_cntl_stat_t data = {0xAA, 0xAA}; 00037 uint16_t rtn_val; 00038 // first read CR 00039 rtn_val = get_cntl_stat_reg(&data); 00040 // applay dst 00041 if (dst) { 00042 data.control |= 1<<3; 00043 } else { 00044 data.control &= ~(1<<3); 00045 } 00046 //write back 00047 rtn_val = set_cntl_stat_reg(data); 00048 return 0; 00049 } 00050 /** bool get_DST() 00051 */ 00052 bool get_DST() { 00053 ds3231_cntl_stat_t data = {0xAA, 0xAA}; 00054 uint16_t rtn_val; 00055 // first read CR 00056 rtn_val = get_cntl_stat_reg(&data); 00057 // get dst 00058 if (data.control & (1<<3)) { 00059 return true; 00060 } else { 00061 return false; 00062 } 00063 } 00064 }; 00065 00066 int main() 00067 { 00068 00069 // WordClock object with leds connected to p5 (MOSI) 00070 WordClock clock(p5); 00071 00072 //RTC with DS3231 : sda, scl 00073 Ds3231_dst rtc(p9, p10); 00074 00075 Timer timer; 00076 00077 //DaylightSavingTIme? 00078 bool DST = false; 00079 00080 //Serial pc(USBTX, USBRX); // tx, rx 00081 { 00082 //pc.baud(115200); 00083 //pc.printf("\n\rconnected to mbed - WordClock...\n\r"); 00084 00085 // set time and date 00086 //time and calendar variables 00087 ds3231_time_t time = {0, 39, 21, 0, 0}; //sec, min, hour, am/pm(1=pm), mode(1=12h) 00088 ds3231_calendar_t calendar = {1, 6, 11, 17}; //day(1=mon), date, month, year(2 digits) 00089 uint16_t rtn_val; 00090 // only set RTC when required! 00091 // rtn_val = rtc.set_time(time); 00092 // rtn_val = rtc.set_calendar(calendar); 00093 // rtn_val = rtc.set_DST(false); 00094 00095 // initial display 00096 00097 // show Winterzeit/Sommerzeit as stored in RTC 00098 if (rtc.get_DST()){ 00099 // SZ for Sommerzeit 00100 clock.sz(); 00101 DST = true; 00102 }else{ 00103 //WZ for Winterzeit 00104 clock.wz(); 00105 DST = false; 00106 } 00107 wait(2); 00108 00109 while (1) { //display loop 00110 00111 //read time 00112 rtn_val = rtc.get_time(&time); 00113 //pc.printf("%2d:%2d ",time.hours,time.minutes); 00114 00115 // read date 00116 rtn_val = rtc.get_calendar(&calendar); 00117 //pc.printf("%2d.%2d.%2d - day:%d \n\r",calendar.date, calendar.month,calendar.year, calendar.day); 00118 00119 00120 // check for DST time change 00121 // only valid for europe!!! 00122 // see http://www.instructables.com/id/The-Arduino-and-Daylight-Saving-Time-Europe/ 00123 00124 //switch to ~DST on last sunday in october 00125 if (calendar.day == 7 && calendar.month == 10 && calendar.date >= 25 && time.hours == 3 && DST==true){ 00126 time.hours=2; 00127 DST=false; 00128 rtc.set_time(time); 00129 rtc.set_DST(DST); 00130 //show WZ 00131 clock.wz(); 00132 wait(2); 00133 } 00134 //switch to DST on last sunday in march 00135 if (calendar.day == 7 && calendar.month == 3 && calendar.date >= 25 && time.hours == 2 && DST==false){ 00136 time.hours=3; 00137 DST=true; 00138 rtc.set_time(time); 00139 rtc.set_DST(DST); 00140 //show SZ 00141 clock.sz(); 00142 wait(2); 00143 } 00144 00145 //display time 00146 clock.display_time(time.hours,time.minutes,time.seconds); 00147 wait_ms(100); 00148 } 00149 00150 //testprograms from here on 00151 00152 timer.start(); 00153 00154 while(1) { 00155 // time fast forward 00156 for (int h=0; h<=23; h++) { 00157 for (int m=0; m<=59; m++) { 00158 for (int s=0; s<=59; s++) { 00159 clock.display_time(h,m,s); 00160 wait_ms(5); 00161 } 00162 } 00163 } 00164 00165 // all words 00166 for (int i=1; i<=NUMWORDS; i++) { 00167 clock.test_display(3,i); 00168 wait_ms(800); 00169 } 00170 //all leds on with rainbow colors 00171 while ( int(timer.read()/10.0) %2 == 0) { 00172 clock.test_display(1); 00173 wait_ms(100); 00174 } 00175 // every led on for 250ms 00176 for (int i=0; i<NUMLEDS; i++) { 00177 clock.test_display(2,i); 00178 wait_ms(250); 00179 } 00180 timer.reset(); 00181 } 00182 } 00183 00184 }
Generated on Wed Jul 13 2022 04:30:48 by
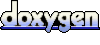