
Testprogram for TMC2209-Library. Uses Speed-Control via VACTUAL instead of Step/Dir
Dependencies: TMCStepper mRotaryEncoder-os
main.cpp
00001 #include "mbed.h" 00002 #include "platform/mbed_thread.h" 00003 #include "TMCStepper.h" 00004 #include "mRotaryEncoder.h" 00005 00006 /* 00007 Testprogram for TMCStepper-Library 00008 TMCStepper based on https://github.com/teemuatlut/TMCStepper for Arduino 00009 by https://github.com/teemuatlut 00010 +++++ 00011 Tested with https://github.com/bigtreetech/BIGTREETECH-TMC2209-V1.2 00012 */ 00013 00014 00015 DigitalOut ledCW(LED1); // Show rotation clockwise // Gren LED LD3 00016 DigitalOut ledCCW(NC); // Show rotation counterclockwise // no more LEDs on L432 00017 00018 //Virtual serial port over USB with 15200 baud 8N1 00019 static BufferedSerial host(USBTX, USBRX,115200); 00020 00021 //mRotaryEncoder(PinName pinA, PinName pinB, PinName pinSW, PinMode pullMode=PullUp, int debounceTime_us=1000) 00022 mRotaryEncoder wheel(D2, D3, D6,PullUp,3000); // default 1500 00023 //D7, D8 not available on L432KC with default solder bridges 00024 00025 // hardware parameters: 00026 // MOTOR Steps per Revolution ( 1/8 Microsteps, 200Steps per Rev / 1.8 degrees per FullStep) 00027 #define MSPR 1600 00028 // Gear Ratio 00029 #define GR 288 00030 00031 #define DRIVER_ADDRESS 0b00 // TMC2209 Driver address according to MS1 and MS2 00032 #define R_SENSE 0.11f // R-Sense in OHM. Match to your driver 00033 #define MICROSTEPS 256 // # of microsteps 00034 #define RMSCURRENT 800 // RMS current of Stepper Coil in mA 00035 #define MAXSPEED 5500 // Maximaum speed (5500 with RMS800 @12V/0.6Amax) 00036 00037 // A TMCStepper-object with UART and given address and R-Sense 00038 //RX, TX, RS, Addr 00039 TMC2209Stepper stepper(D0, D1, R_SENSE, DRIVER_ADDRESS); 00040 00041 InterruptIn diag(D12); 00042 DigitalOut enn(D11); 00043 00044 volatile bool enc_pressed = false; // Button of rotaryencoder was pressed 00045 volatile bool enc_rotated = false; // rotary encoder was totaded left or right 00046 volatile bool enc_action = false; // any change happened 00047 volatile bool diag_event = false; // DIAG-Pin of TMC 00048 int lastGet; 00049 int thisGet; 00050 00051 //interrup-Handler for button on rotary-encoder 00052 void trigger_sw() { 00053 enc_pressed = true; // just set the flag, rest is done outside isr 00054 } 00055 00056 //interrup-Handler for rotary-encoder rotation 00057 void trigger_rotated() { 00058 enc_rotated = true; // just set the flag, rest is done outside isr 00059 } 00060 00061 //interrup-Handler for TMC2209-DIAG-Pin 00062 void trigger_diag() { 00063 diag_event = true; // just set the flag, rest is done outside isr 00064 } 00065 00066 // Assumes little endian 00067 void printBits(size_t const size, void const * const ptr) 00068 { 00069 unsigned char *b = (unsigned char*) ptr; 00070 unsigned char byte; 00071 int i, j; 00072 // puts("#"); 00073 for (i = size-1; i >= 0; i--) { 00074 for (j = 7; j >= 0; j--) { 00075 byte = (b[i] >> j) & 1; 00076 printf("%u", byte); 00077 } 00078 } 00079 // puts("#"); 00080 } 00081 00082 int main() 00083 { 00084 printf("\r\nConnected to mbed\r\n"); 00085 printf("This is Mbed OS %d.%d.%d.\n", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00086 //Intitiallize RotaryEncoder 00087 // call trigger_sw() when button of rotary-encoder is pressed 00088 wheel.attachSW(&trigger_sw); 00089 // call trigger_rot() when the shaft is rotaded left or right 00090 wheel.attachROT(&trigger_rotated); 00091 lastGet = 0; 00092 // set enc_rotated, so startup 00093 enc_rotated = true; 00094 enc_action = true; 00095 ledCW = 1; 00096 ledCCW = 1; 00097 00098 // disable Driver 00099 enn=1; 00100 // wait for Hhardware to settle 00101 ThisThread::sleep_for(100ms); 00102 00103 // Initialize Stepper 00104 printf("connecting to TMC-Module...\r\n"); 00105 stepper.begin(); // UART: Init SW UART (if selected) with default baudrate 00106 00107 //read and check version - must be 0x21 00108 uint8_t tmc_version = stepper.version(); 00109 printf("TMC-Version: %02X\r\n",tmc_version); 00110 if (tmc_version != 0x21) { 00111 printf("Wrong TMC-Version(not 0x21) or communication error!! STOPPING!!!\r\n"); 00112 if (stepper.CRCerror) { 00113 printf("CRC-Error!!!\r\n"); 00114 } 00115 while (1) { 00116 ledCW = 1; 00117 ledCCW = 0; 00118 ThisThread::sleep_for(50ms); 00119 ledCW = 0; 00120 ledCCW = 1; 00121 ThisThread::sleep_for(50ms); 00122 }; 00123 } 00124 00125 stepper.push(); // initialize all registers??? required? 00126 00127 stepper.toff(3); // Enables driver in software - 3, 5 ???? 00128 stepper.rms_current(RMSCURRENT); // Set motor RMS current in mA / min 500 for 24V/speed:3000 00129 // 1110, 800 00130 // working: 800 12V/0,6Amax, Speed up to 5200=4U/min 00131 00132 stepper.microsteps(MICROSTEPS); // Set microsteps to 1:Fullstep ... 256: 1/256th 00133 stepper.en_spreadCycle(true); // Toggle spreadCycle on TMC2208/2209/2224: default false, true: much faster!!!! 00134 stepper.pwm_autoscale(true); // Needed for stealthChop 00135 00136 // enable driver 00137 enn = 0; 00138 00139 uint8_t gstat = stepper.GSTAT(); 00140 printf("GSTAT(): "); printBits(sizeof(gstat),&gstat);printf("\r\n"); 00141 00142 uint32_t gconf = stepper.GCONF(); 00143 printf("GCONF(): "); printBits(sizeof(gconf),&gconf);printf("\r\n"); 00144 00145 uint32_t status = stepper.DRV_STATUS(); 00146 printf("DRV_STATUS(): "); printBits(sizeof(status),&status);printf("\r\n"); 00147 00148 uint32_t ioin = stepper.IOIN(); 00149 printf("IOIN(): "); printBits(sizeof(ioin),&ioin);printf("\r\n"); 00150 00151 uint8_t ihold = stepper.ihold(); 00152 printf("IHOLD(): "); printBits(sizeof(ihold),&ihold);printf("\r\n"); 00153 00154 uint8_t irun = stepper.irun(); 00155 printf("IRUN(): "); printBits(sizeof(irun),&irun);printf("\r\n"); 00156 00157 uint8_t iholddelay = stepper.iholddelay(); 00158 printf("IHOLDDELAY(): "); printBits(sizeof(iholddelay),&iholddelay);printf("\r\n"); 00159 00160 // do a peep by setting vactual to a too high speed 00161 00162 //stepper.VACTUAL(50000*MICROSTEPS); 00163 //ThisThread::sleep_for(1s); 00164 00165 // initialize Automatic tunig (Chap 6.1) 00166 printf("Start automatic tunig Chap6.1 ....."); 00167 stepper.VACTUAL(1); 00168 stepper.VACTUAL(0); 00169 ThisThread::sleep_for(100ms); 00170 stepper.VACTUAL(500*MICROSTEPS); 00171 ThisThread::sleep_for(2s); 00172 stepper.VACTUAL(0); 00173 printf("done\r\n"); 00174 00175 diag.rise(&trigger_diag); 00176 00177 //bool shaft = false; //direction CW or CCW 00178 00179 while(1) { 00180 /* Spped-UP/Down-Cycles 00181 // printf("TSTEP(): %i\r\n", stepper.TSTEP()); 00182 uint32_t status = stepper.DRV_STATUS(); 00183 printf("DRV_STATUS(): "); printBits(sizeof(status),&status);printf("\r\n"); 00184 uint32_t ioin = stepper.IOIN(); 00185 printf("IOIN(): "); printBits(sizeof(ioin),&ioin);printf("\r\n"); 00186 // uint32_t otp = stepper.OTP_READ(); 00187 // printf("OTP_READ(): ");printBits(sizeof(otp),&otp);printf("\r\n"); 00188 00189 printf("VACTUAL(): %zu \r\n", stepper.VACTUAL()); 00190 // increase 00191 uint32_t maxspeed = 3000; //max 3400 or 3000 00192 uint32_t actspeed = 0; 00193 while (actspeed < maxspeed) { 00194 actspeed += 200; 00195 if (actspeed > maxspeed) { 00196 actspeed = maxspeed; 00197 } 00198 printf("actspeed: %i",actspeed); 00199 stepper.VACTUAL(actspeed*MICROSTEPS);// Set Speed to value 00200 ThisThread::sleep_for(25ms); //wait 00201 } 00202 printf("VACTUAL(): %zu \r\n", stepper.VACTUAL()); 00203 ThisThread::sleep_for(5s); 00204 // decrease 00205 maxspeed = 0; 00206 while (actspeed > maxspeed) { 00207 actspeed -= 200; 00208 if (actspeed < 0) { 00209 actspeed = 0; 00210 } 00211 printf("actspeed: %i",actspeed); 00212 stepper.VACTUAL(actspeed*MICROSTEPS);// Set Speed to value 00213 ThisThread::sleep_for(25ms); //wait 00214 } 00215 00216 // stepper.VACTUAL(400*MICROSTEPS);// Set Speed to value 00217 ThisThread::sleep_for(5s); //wait 00218 // inverse direction 00219 shaft = !shaft; 00220 stepper.shaft(shaft); 00221 // Read Interace-Count 00222 printf("IFCNT(): %zu \r\n",stepper.IFCNT()); 00223 printf("...\r\n"); 00224 */ 00225 ////// Control motor-speed by rotary-encoder 00226 00227 // DIAG-PIN showed Error-Condition? 00228 if (diag_event) { 00229 diag_event = false; 00230 printf("DIAG occured!\r\n"); 00231 gstat = stepper.GSTAT(); 00232 printf("GSTAT(): "); printBits(sizeof(gstat),&gstat);printf("\r\n"); 00233 status = stepper.DRV_STATUS(); 00234 printf("DRV_STATUS(): "); printBits(sizeof(status),&status);printf("\r\n"); 00235 //safty turn off friver 00236 printf("Shutting Down Motordriver...\r\n"); 00237 enn=1; 00238 stepper.toff(0); 00239 status = stepper.DRV_STATUS(); 00240 printf("DRV_STATUS(): "); printBits(sizeof(status),&status);printf("\r\n"); 00241 ioin = stepper.IOIN(); 00242 printf("IOIN(): "); printBits(sizeof(ioin),&ioin);printf("\r\n"); 00243 ihold = stepper.ihold(); 00244 printf("IHOLD(): "); printBits(sizeof(ihold),&ihold);printf("\r\n"); 00245 irun = stepper.irun(); 00246 printf("IRUN(): "); printBits(sizeof(irun),&irun);printf("\r\n"); 00247 iholddelay = stepper.iholddelay(); 00248 printf("IHOLDDELAY(): "); printBits(sizeof(iholddelay),&iholddelay);printf("\r\n"); 00249 printf("stopping programm - manual RESET required!!!!!!\r\n"); 00250 while (1) { 00251 ledCW = 1; 00252 ledCCW = 1; 00253 ThisThread::sleep_for(200ms); 00254 ledCW = 0; 00255 ledCCW = 0; 00256 ThisThread::sleep_for(200ms); 00257 }; 00258 00259 } 00260 00261 // shaft has been rotated? 00262 if (enc_rotated) { 00263 enc_rotated = false; 00264 enc_action = true; 00265 thisGet = wheel.Get(); 00266 if (thisGet*100 > MAXSPEED) { //on upper limit? 00267 wheel.Set( MAXSPEED/100); 00268 thisGet = wheel.Get(); 00269 } 00270 if (thisGet*100 < MAXSPEED*(-1)) { //on lower limit? 00271 wheel.Set( MAXSPEED*(-1)/100); 00272 thisGet = wheel.Get(); 00273 } 00274 stepper.VACTUAL(thisGet*100*MICROSTEPS);// Set Speed to value 00275 printf("actspeed: %i\r\n",thisGet*100); 00276 00277 } 00278 // Button pressed? 00279 if (enc_pressed) { 00280 enc_pressed = false; 00281 enc_action = true; 00282 wheel.Set(0); 00283 thisGet = wheel.Get(); 00284 stepper.VACTUAL(thisGet*100*MICROSTEPS);// Set Speed to value 00285 printf("actspeed: %i\r\n",thisGet*100); 00286 00287 gstat = stepper.GSTAT(); 00288 printf("GSTAT(): "); printBits(sizeof(gstat),&gstat);printf("\r\n"); 00289 gconf = stepper.GCONF(); 00290 printf("GCONF(): "); printBits(sizeof(gconf),&gconf);printf("\r\n"); 00291 status = stepper.DRV_STATUS(); 00292 printf("DRV_STATUS(): "); printBits(sizeof(status),&status);printf("\r\n"); 00293 ioin = stepper.IOIN(); 00294 printf("IOIN(): "); printBits(sizeof(ioin),&ioin);printf("\r\n"); 00295 ihold = stepper.ihold(); 00296 printf("IHOLD(): "); printBits(sizeof(ihold),&ihold);printf("\r\n"); 00297 irun = stepper.irun(); 00298 printf("IRUN(): "); printBits(sizeof(irun),&irun);printf("\r\n"); 00299 iholddelay = stepper.iholddelay(); 00300 printf("IHOLDDELAY(): "); printBits(sizeof(iholddelay),&iholddelay);printf("\r\n"); 00301 00302 } 00303 // anything changed? 00304 if (enc_action) { 00305 enc_action = false; 00306 // show direction of motor on leds 00307 if (thisGet > 0) { 00308 ledCW = 1; 00309 ledCCW= 0; 00310 } 00311 if (thisGet < 0) { 00312 ledCW = 0; 00313 ledCCW= 1; 00314 } 00315 if (thisGet == 0) { 00316 ledCW = 1; 00317 ledCCW= 1; 00318 } 00319 } 00320 } // while 1 00321 }
Generated on Mon Jul 18 2022 19:14:43 by
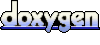