
Test program to send MAX!-Messages with a RFM22-Module
Dependencies: RF22 TextLCD TextLCDScroll mbed RF22Max
main.cpp
00001 // Testprogramm for RFM22B with RF22Max-Library to read ELV MAX! window Shutter-Contacts and PushBottons 00002 00003 //show debug output on Serial pc 00004 #define DEBUG 00005 00006 #include "mbed.h" 00007 #include <RF22Max.h> 00008 #include "TextLCDScroll.h" 00009 00010 // show debug-output on console 00011 //#define DEBUG 00012 00013 Serial pc(USBTX, USBRX); 00014 00015 Ticker SendTicker; 00016 00017 volatile int doSend = 0; // set to 1 by Ticker-Interrupt and read in main-loop 00018 00019 TextLCDScroll lcd(p30, p29, p28, p27, p26, p25, TextLCD::LCD16x2); // rs, e, d4-d7 00020 00021 // mbed LEDs 00022 DigitalOut led1(LED1); 00023 DigitalOut led2(LED2); 00024 DigitalOut led3(LED3); 00025 DigitalOut led4(LED4); 00026 00027 // Singleton instance of the radio 00028 //rf22(PinName slaveSelectPin , PinName mosi, PinName miso, PinName sclk, PinName interrupt ); 00029 RF22Max rf22(p14,p11,p12,p13,p15); 00030 00031 void Ticker_isr() 00032 { 00033 led1 = !led1; 00034 doSend = 1; 00035 } 00036 00037 00038 int main() 00039 { 00040 00041 00042 char lcdline[500]; 00043 00044 RF22Max::max_message MyMessage; 00045 int i; 00046 00047 uint8_t sbuf[RF22_MAX_MESSAGE_LEN]; 00048 00049 uint8_t slen = sizeof(sbuf); 00050 00051 pc.baud(115200); 00052 00053 pc.printf("\n\rConnected to mbed\n\r"); 00054 00055 00056 char version_str [80] = "RF22-Send-Test!-V1.0"; 00057 lcd.cls(); 00058 lcd.setLine(0,version_str); 00059 pc.printf("%s\n\r",version_str); 00060 00061 00062 pc.printf("Pre-init|"); 00063 if (!rf22.init()) 00064 pc.printf("RF22Max init failed\n\r"); 00065 pc.printf("Post-init\n\r"); 00066 00067 00068 rf22.setModeRx(); 00069 00070 00071 SendTicker.attach(&Ticker_isr, 5.0); // Send every 5 seconds 00072 00073 //wait forever and see what comes in 00074 while (1) { 00075 00076 // did we get a MAX!-Message? 00077 if (rf22.recv_max(&MyMessage)) { 00078 // we got a message 00079 led2 = !led2; 00080 sprintf(lcdline,"Got Message type: %s Msg-Nr:%i from Device-ID:%06X State:%s Battery %s", MyMessage.type_str,MyMessage.cnt, MyMessage.frm_adr, MyMessage.state, MyMessage.battery_state); 00081 pc.printf("%s\n\r",lcdline); 00082 lcd.setLine(0,lcdline); 00083 00084 } 00085 00086 if (doSend) { 00087 //we should send something 00088 doSend = 0; 00089 led4=1; 00090 // try to send a simulated window-shutterContact-message 00091 // wait some time 00092 sbuf[0] = 11; // MsgLen 00093 sbuf[1] = 0x21; // MsgCount ?? 00094 sbuf[2] = 0x00; // Flag 00095 sbuf[3] = 0x30; // Type = Cmd = ShutterContactState 00096 sbuf[4] = 0x11; // From Fake Address 00097 sbuf[5] = 0x11; // From 00098 sbuf[6] = 0x11; // From 00099 sbuf[7] = 0x00; // To Address : broadcast 00100 sbuf[8] = 0x00; 00101 sbuf[9] = 0x00; 00102 sbuf[10] = 0x00; // GroupId 00103 sbuf[11] = 0x02; //Payload is 0x02: State Open, Battery: good 00104 slen = 12+2; //+2Byte CRC???? 00105 /* Calculate CRC */ 00106 uint16_t scrc = rf22.calc_crc(sbuf, slen - 2); 00107 sbuf[12] = scrc >> 8; 00108 sbuf[13] = scrc & 0xff; 00109 00110 00111 if (rf22.send(sbuf,slen)) { 00112 #ifdef DEBUG 00113 pc.printf("Send window-shutterContact-message OK\n\r"); 00114 #endif 00115 } else { 00116 #ifdef DEBUG 00117 pc.printf("Send window-shutterContact-message NOT OK\n\r"); 00118 #endif 00119 } 00120 led4 = 0; 00121 00122 } 00123 00124 } 00125 } 00126 00127
Generated on Wed Jul 13 2022 05:31:52 by
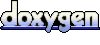