Library to display menus on TextLCDs. Interaction with functions Up,Down and Select (Buttons or RPG) Based on menu-library from pyeh9
Fork of Menu by
Navigator.h
00001 #ifndef NAVIGATOR_H 00002 #define NAVIGATOR_H 00003 00004 #include "mbed.h" 00005 #include "Menu.h" 00006 #include "TextLCD.h" 00007 00008 /** Class Navigator does the Navigation in the menu and updates the display. 00009 * Interaction from outside is done by calling moveUp(), moveDown() or select(). 00010 * Could be done by an RPG or via Buttons. 00011 * 00012 * Example: 00013 * 00014 * @code 00015 * 00016 * #include "MenuItem.h" 00017 * #include "Menu.h" 00018 * #include "Navigator.h" 00019 * #include <vector> 00020 * #include <string> 00021 * PinDetect T1 ( p21,PullNone); //Button 1 - UP 00022 * PinDetect T2 ( p22,PullNone); //Button 2 - Down 00023 * PinDetect T3 ( p23,PullNone); //Button 3 - Select 00024 * ... 00025 * // Here is the heart of the system: the navigator. 00026 * // The navigator takes in a reference to the root and a reference to an lcd 00027 * Navigator navigator(&rootMenu, &lcd); 00028 * 00029 * // attach the methods for buttons Up, Down, Select to the navigator 00030 * T1.attach_asserted( &navigator, &Navigator::moveUp); 00031 * T2.attach_asserted( &navigator, &Navigator::moveDown); 00032 * T3.attach_asserted( &navigator, &Navigator::select); 00033 * // do whatever you need to do in your main-loop. 00034 * while( 1 ) { 00035 * led4 = !led4; 00036 * wait( 1 ); 00037 * } 00038 * @endcode 00039 */ 00040 class Navigator 00041 { 00042 00043 public: 00044 Navigator(Menu *, TextLCD_Base *); 00045 Menu *activeMenu; // the current menu - can change when Menue-item is selected on selection with child menu 00046 00047 TextLCD_Base *lcd; 00048 00049 /** Move up one line in menu. 00050 * call this method when user moves up one line. 00051 * can be triggered by RPG or Button (PinDetect) or otherwise. 00052 */ 00053 void moveUp(); 00054 00055 /** Move down one line in menu. 00056 * call this method when user moves down one line. 00057 * can be triggered by RPG or Button (PinDetect) or otherwise. 00058 */ 00059 void moveDown(); 00060 00061 /** User presses Select Button. 00062 * call this method when user wants to select an item. 00063 * can be triggered by RPG or Button (PinDetect) or otherwise. 00064 */ 00065 void select(); 00066 00067 /** print the menu on LCD 00068 */ 00069 void printMenu(); 00070 00071 /** print cursor on the beginning of line 00072 */ 00073 void printCursor(); 00074 00075 private: 00076 /** Show Yes/No Dialog and wait fo Selection 00077 */ 00078 void show_yes_no(bool yesorno); 00079 /** Show a long Text and wait for Select. Scroll Up/Down in Text 00080 */ 00081 void show_longtext(void); 00082 00083 int _display_rows; // number of rows the LCD can display 00084 int _display_cols; // number of lines of LCD 00085 int _cursorPos; // what selection the cursor points to 00086 int _cursorLine; // what line of the lcd the cursor is on. 1 = first line, 2 = second line 00087 int _start_line; // display a long text starting from this line 00088 bool _wait_for_select; // only accept Select Button to go Back 00089 bool _wait_for_yesno; // up/don change selection ; Select accepts 00090 00091 }; 00092 00093 #endif
Generated on Fri Jul 15 2022 06:41:56 by
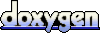