
Build upon MMA7660_HelloWorld to pull out x, y, z axes from device and print to LCD on mbed Application Board
Dependencies: C12832_lcd MMA7660 mbed
Fork of MMA7660_HelloWorld by
main.cpp
00001 //Iteration for 3 axis, ... 00002 //This code is being developed for use with 00003 //Sphere and Ring type Physiotherapy Balance Boards 00004 //example images at - 00005 //http://www.balance360.com/servlet/the-Balance-360-Boards/Categories 00006 //The author has no commercial, or other, relationship with [Balance 360] or other manufacturers 00007 //The author is simply having fun 00008 //C B P Chapman 00009 00010 #include "mbed.h" 00011 #include "MMA7660.h" 00012 #include "C12832_lcd.h" 00013 00014 C12832_LCD lcd; 00015 MMA7660 MMA(p28, p27); 00016 00017 DigitalOut connectionLed(LED1);//operation confirmed 00018 DigitalOut servoProxyLed(LED2);//score outcome, proxy for servo 00019 00020 const int n = 10; //number of readings to be averaged, change globally here 00021 float score = 0; //reserved for later 00022 int angle = 0; //reserved for later 00023 float pulseXT =0, pulseYT = 0; //Total holders for summation from axis arrays 00024 00025 float pulseXa, pulseYa; //averaged values for each axis over n 00026 float pulseX[n], pulseY[n]; //arrays to hold n readings for each axis 00027 int i, j; //indexing variables 00028 00029 int main() 00030 { 00031 00032 while(1) { 00033 00034 for (i = 0; i < n; i = i + 1) { //read n values into each axis array 00035 pulseX[i] = MMA.x(); 00036 pulseY[i] = MMA.y(); 00037 } 00038 pulseXT = 0; //reset Totala 00039 pulseYT = 0; //reset Totala 00040 for (j = 0; j < n; j = j + 1) { //summation of the contents of each array into axis Totals 00041 pulseXT = pulseXT+pulseX[j]; 00042 pulseYT = pulseYT+pulseY[j]; 00043 } 00044 pulseXa = pulseXT/n; //axis average over n 00045 00046 pulseYa = pulseYT/n; //axis average over n 00047 00048 if (MMA.testConnection()) 00049 connectionLed = 1; 00050 00051 if (pulseXa > (-0.2) && pulseXa < (0.2) && pulseYa > (-0.2) && pulseYa < (0.2)) {//average result within stability range; x, y 00052 lcd.cls();//clear LCD for next reading round 00053 lcd.locate(3,3);//first LCD column label 00054 lcd.printf("x-axis | ");//label column 00055 lcd.locate(3,12);//xdata location 00056 lcd.printf("%.2f\n",pulseXa);//print x to LCD 00057 lcd.locate(40,3);//second LCD column label 00058 lcd.printf("y-axis | ");//label column 00059 lcd.locate(40,12);//ydata location 00060 lcd.printf("%.2f\n",pulseYa);//print y to LCD 00061 lcd.locate(77,3);//initial LCD location 00062 lcd.printf("z-axis");//label column 00063 lcd.locate(77,12);//zdata location 00064 lcd.printf("%.2f\n",MMA.z());//print z to LCD 00065 lcd.locate(3,21);//flag location 00066 lcd.printf("STABLE");//flag 00067 if (score != 10) {//if score has not reached 10 00068 ++score;//add 1 to score 00069 lcd.locate(70,21);//score location 00070 lcd.printf("SCORE = "); 00071 lcd.printf("%.0f\n",score);//print score 00072 } else { 00073 servoProxyLed = 1;//LED2 HIGH 00074 lcd.locate(70,21);//notice location 00075 lcd.printf("NEXT LEVEL"); 00076 wait (10); 00077 servoProxyLed = 0;//LED@ LOW 00078 score = 0;//reset score 00079 00080 } 00081 } 00082 00083 else {////average result not within stability range; x, y 00084 lcd.cls();//clear LCD for next reading round 00085 lcd.locate(3,3);//first LCD column label 00086 lcd.printf("x-axis | ");//label column 00087 lcd.locate(3,12);//xdata location 00088 lcd.printf("%.2f\n",pulseXa);//print x to LCD 00089 lcd.locate(40,3);//second LCD column label 00090 lcd.printf("y-axis | ");//label column 00091 lcd.locate(40,12);//ydata location 00092 lcd.printf("%.2f\n",pulseYa);//print y to LCD 00093 lcd.locate(77,3);//initial LCD location 00094 lcd.printf("z-axis");//label column 00095 lcd.locate(77,12);//zdata location 00096 lcd.printf("%.2f\n",MMA.z());//print z to LCD 00097 lcd.locate(3,21);//flag location 00098 lcd.printf("UNSTABLE");//flag 00099 score = 0;//reset score 00100 lcd.locate(70,21);//score location 00101 lcd.printf("SCORE = "); 00102 lcd.printf("%.0f\n",score);//print score 00103 00104 } 00105 } 00106 }
Generated on Wed Jul 13 2022 13:07:14 by
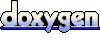