
for frequency correction testing
Dependencies: FreescaleIAP SimpleDMA mbed-rtos mbed
Fork of CDMS_CODE by
Structures.h
00001 // TC TM 00002 #define TC_SHORT_SIZE 11 00003 #define TC_LONG_SIZE 135 00004 #define TM_LONG_SIZE 134 00005 #define TM_SHORT_SIZE 13 00006 00007 #define SHORT_TC_CODE 0 00008 #define LONG_TC_CODE 1 00009 00010 #define SHORT_TM_CODE 1 00011 #define LONG_TM_CODE 0 00012 00013 // COM_RX 00014 #define RX_BUFFER_LENGTH 60 00015 // 60+4 = 64 00016 00017 struct data_node{ 00018 uint8_t values[RX_BUFFER_LENGTH]; 00019 struct data_node* next_node; 00020 }; 00021 typedef struct data_node COM_RX_DATA_NODE; 00022 00023 //TELECOMMAND: 00024 00025 /* 00026 exec_status: 00027 0: unexecuted 00028 1: successfully executed 00029 2: Execution Failed 00030 3: Disabled 00031 4: Marked For retry 00032 */ 00033 #define TC_STATE_UNEXECUTED 0x00 00034 #define TC_STATE_SUCCESSFULLY_EXECUTED 0x01 00035 #define TC_STATE_EXECUTION_FAILED 0x02 00036 #define TC_STATE_EXECUTION_UNKNOWN 0x03 00037 #define TC_STATE_DISABLED 0x04 00038 #define TC_STATE_MARKED_RETRY 0x05 00039 00040 //MASKS 00041 #define SHORT_LONG_TC_MASK 0x10 00042 #define CRC_MASK 0x08 00043 #define EXEC_STATUS_MASK 0x07 00044 00045 //USE ONLY THE BELOW MACROS TO MODIFY 'flags' VARIABLE 00046 //x should be a Base_tc pointer 00047 #define GETshort_or_long_tc(x) ( ( (x->flags) & SHORT_LONG_TC_MASK ) >> 4 ) 00048 #define GETcrc_pass(x) ( ( (x->flags) & CRC_MASK ) >> 3 ) 00049 #define GETabort_on_nack(x) ( ( (x->TC_string[1]) & 0x08 ) >> 3 ) 00050 #define GETapid(x) ( ( (x->TC_string[1]) & 0xC0 ) >> 6 ) 00051 #define GETexec_status(x) ( (x->flags) & EXEC_STATUS_MASK ) 00052 #define GETpacket_seq_count(x) (x->TC_string[0]) 00053 #define GETservice_type(x) ( (x->TC_string[2]) & 0xF0 ) 00054 #define GETservice_subtype(x) ( (x->TC_string[2]) & 0x0F ) 00055 #define GETpid(x) (x->TC_string[3]) 00056 00057 //x should be a Base_tc pointer 00058 //y should be a 8-bit number with relevant data in LSB 00059 //use in a seperate line with ; at the end: similar to a function 00060 #define PUTshort_or_long(x,y) x->flags = ( (x->flags) & ~(SHORT_LONG_TC_MASK)) | ( (y << 4) & SHORT_LONG_TC_MASK ) 00061 #define PUTcrc_pass(x,y) x->flags = ( (x->flags) & ~(CRC_MASK)) | ( (y << 3) & CRC_MASK) 00062 #define PUTexec_status(x,y) x->flags = ( (x->flags) & ~(EXEC_STATUS_MASK)) | ( y & EXEC_STATUS_MASK) 00063 00064 //PARENT TELECOMMAND CLASS 00065 class Base_tc{ 00066 public: 00067 uint8_t flags; 00068 uint8_t *TC_string; 00069 Base_tc *next_TC; 00070 00071 virtual ~Base_tc(){} 00072 }; 00073 00074 //DERIVED CLASS - SHORT TC 00075 class Short_tc : public Base_tc{ 00076 private: 00077 uint8_t fix_str[TC_SHORT_SIZE]; 00078 public: 00079 Short_tc(){ 00080 TC_string = fix_str; 00081 flags = 0; 00082 } 00083 00084 ~Short_tc(){} 00085 }; 00086 00087 //DERIVED CLASS - LONG TC 00088 class Long_tc : public Base_tc{ 00089 private: 00090 uint8_t fix_str[TC_LONG_SIZE]; 00091 public: 00092 Long_tc(){ 00093 TC_string = fix_str; 00094 flags = 0; 00095 } 00096 00097 ~Long_tc(){} 00098 }; 00099 00100 // TELEMETRY: 00101 // MASK 00102 #define SHORT_LONG_TM_MASK 0x80 00103 00104 //x should be 'Base tm' pointer 00105 #define GETshort_or_long_tm(x) ((x->TM_string[0] & SHORT_LONG_TM_MASK) >> 7) 00106 00107 // PARENT TELEMETRY CLASS 00108 class Base_tm{ 00109 public: 00110 uint8_t *TM_string; 00111 Base_tm *next_TM; 00112 00113 virtual ~Base_tm(){} 00114 }; 00115 00116 // DERIVED CLASS : Long tm [type 0] 00117 // type 0 00118 class Long_tm : public Base_tm{ 00119 private: 00120 uint8_t fix_str[TM_LONG_SIZE]; 00121 public: 00122 Long_tm(){ 00123 TM_string = fix_str; 00124 // type 0 00125 } 00126 00127 ~Long_tm(){} 00128 }; 00129 00130 // DERIVED CLASS : Short tm [type 1] 00131 // type 1 00132 class Short_tm : public Base_tm{ 00133 private: 00134 uint8_t fix_str[TM_SHORT_SIZE]; 00135 public: 00136 Short_tm(){ 00137 TM_string = fix_str; 00138 // type 1 00139 } 00140 00141 ~Short_tm(){} 00142 }; 00143 00144 00145 // CDMS HK 00146 00147 #define tstart -50 00148 #define tstep 1 //to be finalized by thermal team 00149 #define tstart_thermistor -40 00150 #define tstep_thermistor 1 00151 00152 typedef struct CDMS_HK_actual 00153 { 00154 float temp_actual[16]; 00155 float CDMS_temp_actual; 00156 00157 }CDMS_HK_actual; 00158 00159 typedef struct CDMS_HK_quant 00160 { 00161 char temp_quant[16]; 00162 char CDMS_temp_quant; 00163 00164 }CDMS_HK_quant; 00165 00166 typedef struct CDMS_HK_min_max 00167 { 00168 char temp_max[16]; 00169 char temp_min[16]; 00170 char CDMS_temp_min; 00171 char CDMS_temp_max; 00172 }CDMS_HK_min_max;
Generated on Sat Jul 16 2022 01:27:53 by
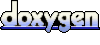