
embernet project fire detection system sensor test
Dependencies: mbed CCS811 BME280
main.cpp
00001 #include "mbed.h" 00002 #include "BME280.h" 00003 #include "CCS811.h" 00004 #include "Adafruit_SGP30.h " 00005 #include <iostream> 00006 00007 using namespace std; 00008 /*------------------------------------------------------------------------------ 00009 Before to use this example, ensure that you an hyperterminal installed on your 00010 computer. More info here: https://developer.mbed.org/handbook/Terminals 00011 00012 The default serial comm port uses the SERIAL_TX and SERIAL_RX pins (see their 00013 definition in the PinNames.h file). 00014 00015 The default serial configuration in this case is 9600 bauds, 8-bit data, no parity 00016 00017 If you want to change the baudrate for example, you have to redeclare the 00018 serial object in your code: 00019 00020 Serial pc(SERIAL_TX, SERIAL_RX); 00021 00022 Then, you can modify the baudrate and print like this: 00023 00024 pc.baud(115200); 00025 pc.printf("Hello World !\n"); 00026 ------------------------------------------------------------------------------*/ 00027 Serial pc(SERIAL_TX, SERIAL_RX); 00028 I2C i2c(PB_7, PB_8); 00029 DigitalOut led(LED1); 00030 //BME280 bme(PB_7, PB_8); 00031 //Adafruit_SGP30 sgp(PB_7, PB_8); 00032 CCS811 ccs(i2c, pc); 00033 00034 uint16_t eco2, tvoc; 00035 //float temp, hum, pres; 00036 00037 void CCS811Initialize(void){ 00038 bool ccs_status = false; 00039 while(ccs_status == false){ 00040 printf("Initialize CCS811 \n"); 00041 ccs.init(); 00042 ccs_status = !ccs.checkHW(); 00043 cout << "CCS Status: " << ccs_status << endl; 00044 } 00045 } 00046 00047 void CCS811Callback(void){ 00048 ccs.readData(&eco2, &tvoc); 00049 printf("eCO2 reading :%dppm, TVOC reading :%dppb\r\n", eco2, tvoc); 00050 } 00051 00052 //void BME280Initialize(void){ 00053 // bool bme_status = false; 00054 // while(bme_status == false){ 00055 // printf("Initialize BME280 \n"); 00056 // bme.initialize(); 00057 // bme_status = true; 00058 // wait(3); 00059 // } 00060 //} 00061 // 00062 // 00063 //void BME280Callback(void){ 00064 //bme.start(); 00065 // cout << "BME Read Status: " << bme.get(temp, pres, hum); 00066 // bme.stop(); 00067 // cout << "Temperature: " << temp << " Pressure: " << pres << " Humidity: " << hum << endl; 00068 // cout << "Temperature: " << bme.getTemperature() ; 00069 // cout << " Pressure: " << bme.getPressure(); 00070 // cout << " Humidity: " << bme.getHumidity() << "\r"<< endl; 00071 //} 00072 00073 //void SGP30Initialize(void){ 00074 // bool sgp_status = false; 00075 // while(sgp_status == false){ 00076 // printf("Initialize SGP30 \n"); 00077 // sgp.begin(); 00078 // sgp_status = sgp.IAQinit(); 00079 // wait(3); 00080 // } 00081 //} 00082 // 00083 //void SGP30Callback(void){ 00084 // sgp.IAQmeasure(); 00085 // cout << "eCO2: " << sgp.eCO2 << " ppm"; 00086 // cout << " TVOC: " << sgp.TVOC << " ppb \r" << endl; 00087 //} 00088 00089 00090 int main() 00091 { 00092 printf("Hello World !\n"); 00093 00094 // SGP30Initialize(); 00095 // BME280Initialize(); 00096 CCS811Initialize(); 00097 00098 00099 while(true) { 00100 wait(3); // second 00101 led = !led; // Toggle LED 00102 // BME280Callback(); 00103 // SGP30Callback(); 00104 CCS811Callback(); 00105 } 00106 }
Generated on Tue Aug 2 2022 21:02:21 by
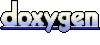