
embernet project fire detection system sensor test
Dependencies: mbed CCS811 BME280
Adafruit_SGP30.h
00001 /*! 00002 * @file Adafruit_SGP30.h 00003 * 00004 * This is the documentation for Adafruit's SGP30 driver for the 00005 * Arduino platform. It is designed specifically to work with the 00006 * Adafruit SGP30 breakout: http://www.adafruit.com/products/3709 00007 * 00008 * These sensors use I2C to communicate, 2 pins (SCL+SDA) are required 00009 * to interface with the breakout. 00010 * 00011 * Adafruit invests time and resources providing this open source code, 00012 * please support Adafruit and open-source hardware by purchasing 00013 * products from Adafruit! 00014 * 00015 * Written by Ladyada for Adafruit Industries. 00016 * 00017 * BSD license, all text here must be included in any redistribution. 00018 * 00019 */ 00020 00021 #include "mbed.h" 00022 00023 // the i2c address 00024 #define SGP30_I2CADDR_DEFAULT 0x58 ///< SGP30 has only one I2C address 00025 00026 // commands and constants 00027 #define SGP30_FEATURESET 0x0020 ///< The required set for this library 00028 #define SGP30_CRC8_POLYNOMIAL 0x31 ///< Seed for SGP30's CRC polynomial 00029 #define SGP30_CRC8_INIT 0xFF ///< Init value for CRC 00030 #define SGP30_WORD_LEN 2 ///< 2 bytes per word 00031 00032 /**************************************************************************/ 00033 /*! Class that stores state and functions for interacting with SGP30 Gas Sensor */ 00034 /**************************************************************************/ 00035 class Adafruit_SGP30 { 00036 public: 00037 Adafruit_SGP30(PinName sda, PinName scl); 00038 bool begin(); 00039 bool IAQinit(void); 00040 bool IAQmeasure(void); 00041 00042 bool getIAQBaseline(uint16_t *eco2_base, uint16_t *tvoc_base); 00043 bool setIAQBaseline(uint16_t eco2_base, uint16_t tvoc_base); 00044 bool getIAQRaw(uint16_t *H2_raw, uint16_t *Eth_raw); 00045 00046 /** 00047 * The last measurement of the IAQ-calculated Total Volatile Organic Compounds in ppb. This value is set when you call {@link IAQmeasure()} 00048 */ 00049 uint16_t TVOC; 00050 00051 /** 00052 * The last measurement of the IAQ-calculated equivalent CO2 in ppm. This value is set when you call {@link IAQmeasure()} 00053 */ 00054 uint16_t eCO2; 00055 00056 /** 00057 * The 48-bit serial number, this value is set when you call {@link begin()} 00058 */ 00059 uint16_t serialnumber[3]; 00060 private: 00061 I2C _i2c; 00062 int _i2caddr; 00063 00064 void write(uint8_t address, uint8_t *data, uint8_t n); 00065 void read(uint8_t address, uint8_t *data, uint8_t n); 00066 bool readWordFromCommand(uint8_t command[], uint8_t commandLength, uint16_t delay, uint16_t *readdata = NULL, uint8_t readlen = 0); 00067 uint8_t generateCRC(uint8_t data[], uint8_t datalen); 00068 };
Generated on Tue Aug 2 2022 21:02:21 by
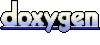