
A simple program to try the TESTBED evaluation KIT http://www.lextronic.fr/P22450-platine-devaluation-testbed.html
Fork of TestBed-LCD20x4 by
main.cpp
00001 // essai LPC1768 sut carte TESTBED 00002 // http://www.lextronic.fr/P22450-platine-devaluation-testbed.html 00003 // test IO, ADC, serial, LCD, interrupt 00004 00005 #include "mbed.h" 00006 #include "TextLCD.h" 00007 00008 // LED1 = P5.5 LED2 = P5.6 sur carte testbed 00009 // 2 Leds sur carte testBed 00010 DigitalOut ledG(p6); 00011 DigitalOut ledR(p5); 00012 00013 // Potentiomètre sur p15 (carte testbed) 00014 AnalogIn pot(p15); 00015 00016 // le port serie emulé sur la connexion USB (USBTX et USBRX), necessite un driver sous Windows 00017 Serial pc(USBTX, USBRX); 00018 00019 //the object "lcd" is initialized to act as a TextLCD with 20x4 characters 00020 TextLCD lcd(p26, p25, p24, p23, p22, p20, p19, TextLCD::LCD20x4); 00021 00022 // interruption sur BTN1 00023 InterruptIn btn1(p8); 00024 00025 // IT periodique 00026 Ticker tempo; 00027 00028 // prototypes fonctions d'IT 00029 void BTN1_interruption(); 00030 void Rx_interruption(); 00031 void flash_interruption(); 00032 00033 int main() 00034 { 00035 //each line of the LCD can be accessed directly using the .locate(column, line) function 00036 lcd.locate(0,0); 00037 lcd.printf("Tests mbed"); 00038 lcd.locate(0,1); 00039 lcd.printf("---------"); 00040 lcd.locate(0,2); 00041 lcd.printf("CD.01-2015"); 00042 lcd.locate(5,3); 00043 lcd.printf("LLAP"); 00044 wait(1); 00045 00046 //the LCD is cleared using function .cls() 00047 lcd.cls(); 00048 // communications 00049 pc.baud(9600); 00050 00051 // interruption sur RX 00052 pc.attach(&Rx_interruption); 00053 00054 // interruption sur BTN1 front descendant, appui 00055 btn1.fall(&BTN1_interruption); 00056 00057 // IT sur timer toutes les 0,5s 00058 tempo.attach_us(&flash_interruption, 500000); 00059 00060 while(1) { 00061 lcd.locate(0,0); 00062 lcd.printf("pot=%f %d \n",pot.read(),pot.read_u16 ()); 00063 lcd.printf("BTN1:IT RXIT affiche\n"); 00064 pc.printf("pot=%f %d\n",pot.read(),pot.read_u16 ()); 00065 wait(0.5); 00066 } 00067 //__WFI(); 00068 } 00069 00070 void BTN1_interruption() 00071 { 00072 static char c=0; 00073 if (!c) { 00074 lcd.locate(5,3); 00075 lcd.printf("INTERRUPTION"); 00076 } else { 00077 lcd.locate(5,3); 00078 lcd.printf(" "); 00079 } 00080 c=~c; 00081 pc.printf("\n ------------INTERRUPTION BTN1----------\n"); 00082 return; 00083 } 00084 00085 void Rx_interruption() 00086 { 00087 lcd.locate(5,3); 00088 lcd.printf("%c ",pc.getc()); 00089 return; 00090 } 00091 00092 void flash_interruption() 00093 { 00094 ledG=!ledG; 00095 ledR=!ledG; 00096 return; 00097 }
Generated on Wed Jul 13 2022 02:47:51 by
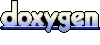