
Simple demo with GPIO MQTT protocol test on STM32 broker tests.mosquitto.org WIFI interface ESP8266 Issue of topic0 by pressing the button If reception of ', switching of the led If received from 'q' end of program
main.cpp
00001 /******************************************************************************* 00002 * Copyright (c) 2014, 2015 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 * Ian Craggs - make sure QoS2 processing works, and add device headers 00016 * 00017 * Adaptation STM32 NUCLEO and mosquitto.org : C.Dupaty 00018 * 06/2020 00019 * 00020 This demo works on NUCLEO STM32. 00021 WIFI link with ESP8266 connected 00022 Configuration in mbed_app.json 00023 target_overrides for the UART connection of the ESP8266 and the WIFI connection (SSID / PASS) 00024 MQTT parameters are configured in #define below 00025 if receive payload with first q -> exit 00026 if receive payload with first l -> toggle LED1 on NUCLEO 00027 * 00028 *******************************************************************************/ 00029 00030 #include "easy-connect.h" 00031 #include "MQTTNetwork.h" 00032 #include "MQTTmbed.h" 00033 #include "MQTTClient.h" 00034 00035 DigitalOut led(LED1); // LED verte (user) 00036 Serial pc(USBTX, USBRX); 00037 //DigitalIn btn(USER_BUTTON); //PC13 sur F411 00038 DigitalIn btn(PA_8,PullUp); // button connected with internal pullup 00039 00040 #define board "NUCLEO_F411RE" 00041 /* 00042 MQTT QOS, There are 3 QoS levels in MQTT 00043 At most once (MQTT::QOS0) 00044 At least once (MQTT::QOS1) 00045 Exactly once (MQTT::QOS2). 00046 */ 00047 #define quality MQTT::QOS0 00048 /* 00049 retained flag : The broker stores the last retained message and the corresponding QoS for that topic. 00050 Each client that subscribes to a topic pattern that matches the topic of the retained 00051 message receives the retained message immediately after they subscribe. 00052 The broker stores only one retained message per topic. 00053 */ 00054 #define ret false 00055 /* 00056 dup flag : The flag indicates that the message is a duplicate 00057 and was resent because the intended recipient (client or broker) did not acknowledge the original message. 00058 */ 00059 #define dupli false 00060 00061 const char* hostname = "test.mosquitto.org"; 00062 const int port = 1883; 00063 char* ID ="mbedSTM32Fourcade"; 00064 char* user =NULL; // user & pass = NULL for broker with no credential. 00065 char* pass =NULL; 00066 const char* topic = "topic0"; 00067 int arrivedcount=0; 00068 bool quit=false; 00069 00070 NetworkInterface* network = easy_connect(true); 00071 MQTTNetwork mqttNetwork(network); 00072 MQTT::Client<MQTTNetwork, Countdown> client(mqttNetwork); 00073 MQTT::Message message; 00074 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00075 00076 void messageArrived(MQTT::MessageData& md) 00077 { 00078 MQTT::Message &message = md.message; 00079 pc.printf("\x1B[33m"); // yellow 00080 pc.printf("Message arrived -> qos %d, retained %d, dup %d, packetid %d\r\n", message.qos, message.retained, message.dup, message.id); 00081 pc.printf("Payload -> %.*s\r\n", message.payloadlen, (char*)message.payload); 00082 ++arrivedcount; 00083 if (*(char*)message.payload=='q') 00084 { 00085 pc.printf("\x1B[31m"); // red 00086 pc.printf("Exit command received\n\r"); 00087 quit=true; 00088 } 00089 if (*(char*)message.payload=='l') 00090 { 00091 led=!led; 00092 pc.printf("\x1B[31m"); // red 00093 pc.printf("toggle LED\n\r"); 00094 } 00095 pc.printf("\x1B[0m"); // raz 00096 } 00097 00098 void connection(void) 00099 { 00100 int rc; 00101 pc.printf("\x1B[32m"); // green 00102 pc.printf("Connecting to MQTT broker %s:%d\r\n", hostname, port); 00103 00104 if ((rc = client.connect(data)) != 0) 00105 pc.printf("rc from MQTT connect is %d\r\n", rc); 00106 else pc.printf("connection MQTT OK ID:%s USER:%s PASS:%s\r\n",ID,user,pass); 00107 00108 if ((rc = client.subscribe(topic, quality, messageArrived)) != 0) 00109 pc.printf("rc from MQTT subscribe is %d\r\n", rc); 00110 else pc.printf("Subscribe MQTT OK topic:%s quality:%d\r\n",topic,quality); 00111 pc.printf("\x1B[0m"); // raz 00112 } 00113 00114 void deconnection(void) 00115 { 00116 int rc; 00117 pc.printf("\x1B[36m"); // cyan 00118 if ((rc = client.unsubscribe(topic)) != 0) pc.printf("rc from unsubscribe was %d\r\n", rc); 00119 else pc.printf("unsubscribe OK \r\n"); 00120 if ((rc = client.disconnect()) != 0) pc.printf("rc from disconnect was %d\r\n", rc); 00121 else pc.printf("disconnect OK\r\n"); 00122 mqttNetwork.disconnect(); 00123 pc.printf("\x1B[0m"); // raz 00124 } 00125 00126 int main(int argc, char* argv[]) 00127 { 00128 int rc; 00129 int cpt=0; 00130 char buf[100]; 00131 00132 //pc.printf("\x1B[2J"); //efface ecran 00133 //pc.printf("\x1B[0;0H"); // curseur en 0,0 00134 pc.printf("\x1B[1m"); // brillant 00135 pc.printf("\r\nMQTT test on %s\r\n",board); 00136 pc.printf("--------------------------\r\n\r\n"); 00137 pc.printf("\x1B[33m"); // yellow 00138 wait(1); 00139 00140 // TCP connection 00141 mqttNetwork.connect(hostname, port); 00142 if (rc != 0) pc.printf("rc from TCP connect is %d\r\n", rc); 00143 else pc.printf("WIFI network connection OK\r\n"); 00144 00145 // MQTT data 00146 data.MQTTVersion = 3; 00147 data.clientID.cstring = ID; 00148 data.username.cstring = user; 00149 data.password.cstring = pass; 00150 message.qos = quality; 00151 message.retained = ret; 00152 message.dup = dupli; 00153 message.payload = (void*)buf; 00154 connection(); 00155 while(!quit) 00156 { 00157 pc.printf("---> Press button to send : %s<--- \n\r",topic); 00158 while(btn); 00159 while(!btn); 00160 //connection(); 00161 sprintf(buf, "Message from %s number -> %d \r\n", board,++cpt); 00162 pc.printf("Send message -> %s\n\r",buf); 00163 message.payloadlen = strlen(buf)+1; 00164 if ((rc = client.publish(topic, message)) !=0) pc.printf("rc publication error %d\r\n", rc); 00165 client.yield(100); 00166 //deconnection(); 00167 } 00168 00169 deconnection(); 00170 pc.printf("Disconnect from MQTT network. End of program, %d messages arrived\r\n",arrivedcount); 00171 00172 return 0; 00173 }
Generated on Fri Jul 22 2022 15:58:27 by
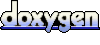