
4 thread with comments
Dependencies: C12832 LM75B mbed-rtos mbed
Fork of rtos_basic by
main.cpp
00001 #include "mbed.h" //Include the mbed.h file 00002 #include "rtos.h" //include the RTOS.h file 00003 #include "LM75B.h" //include the LM75B.h file 00004 #include "C12832.h" //include the C12832.h file 00005 00006 C12832 lcd(p5, p7, p6, p8, p11); //Setup the LCD 00007 00008 LM75B sensor(p28,p27); //Setup the Temp Sensor I2C 00009 Serial pc(USBTX,USBRX); //Setup the serial comm 00010 00011 float Temp_Value; //global var for temp 00012 00013 PwmOut spkr(p26); //PWM speaker 00014 PwmOut LED_red(p23); //PWM LED red 00015 PwmOut LED_blue(p25); //PWM LED blue 00016 00017 DigitalOut led1(LED1); //Setup LED1 to the varible name led1 00018 DigitalOut led2(LED2); //Setup LED2 to the varible name led2 00019 00020 Mutex Temp_Mutex; //Setup Mutex 00021 00022 00023 void Tempature_Senor(void const *args) { //Function or the thread to be called 00024 00025 00026 if (sensor.open()) { //Try to open the LM75B 00027 printf("Device detected!\n"); //printf if device connects 00028 00029 while (1) { 00030 lcd.cls(); //Clears LCD 00031 lcd.locate(0,3); //Locartion on LCD display 00032 Temp_Mutex.lock(); //Mutex Lock sensor 00033 lcd.printf("Temp = %.3f\n", (float)sensor); //Print Temp value on LCD 00034 Temp_Value = (float)sensor; //read sonsor and store in Temp_value 00035 Temp_Mutex.unlock(); //Mutex unlock sensor 00036 Thread::wait(1.0); //Thread wait 00037 } 00038 00039 } else { 00040 error("Device not detected!\n"); //Printf on serial if error 00041 } //End Super loop 00042 } 00043 00044 void Speaker(void const *args) { //Function or the thread to be called 00045 while (true) { //Super loop 00046 spkr.period(1.0/5000); //set period 00047 spkr = 0.25; //set PWM 00048 Thread::wait(800); //Thread wait 00049 spkr.period(1.0/3000); //set period 00050 spkr = 0.25; //set PWM 00051 Thread::wait(800); //Thread wait 00052 } //End Super loop 00053 } 00054 00055 void led_R_B_flash(void const *args) { //Function or the thread to be called 00056 while (true) { //Super loop 00057 00058 LED_red = 0.5; //PWM LED to half brightness 00059 LED_blue = 1; //Turn blue LED off 00060 Thread::wait(800); 00061 LED_red = 1; //Turn red LED off 00062 LED_blue = 0.5; //PWM LED to half brightness 00063 Thread::wait(800); //Thread wait 00064 } //End Super loop 00065 } //End Function / thread 00066 00067 int main() { //Main 00068 float local_Temp_V=0; //SETup local var 00069 Thread thread_Temp(Tempature_Senor); //start thread 00070 Thread thread_LED_Flash(led_R_B_flash); //start thread 00071 Thread thread_Speaker_Sound(Speaker); //start thread 00072 00073 while (1) { 00074 Temp_Mutex.lock(); //Mutex lock temp value 00075 local_Temp_V = Temp_Value; //store temp value in local variable 00076 Temp_Mutex.unlock(); //mutex unlock 00077 printf("Temp = %.3f\n", local_Temp_V ); //printf on serial of local temp value 00078 Thread::wait(1.0); //thread wait 00079 } 00080 00081 00082 } //End main
Generated on Sun Jul 17 2022 01:44:44 by
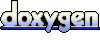