peak detection
Dependents: mbed-test-i2c-PCA-biquad-peakdet Mix-code-v2 mbed-os-step-counting the-lastest-code
peak.cpp
00001 #include "peak.h" 00002 #include <iostream> 00003 #include <algorithm> 00004 using namespace std; 00005 #define max(a, b) (((a) > (b)) ? (a) : (b)) 00006 #define min(a, b) (((a) > (b)) ? (b) : (a)) 00007 00008 int PEAK::findPeaks(vector<float> num,int count, float &height) 00009 { 00010 vector<int> sign; 00011 float min; 00012 float threshold; 00013 for(int i = 1;i<count;i++) 00014 { 00015 /*do difference between the near points: 00016 *result<0,sign = -1 00017 *result>0,sign = 1 00018 *result=0,sign = 0 00019 */ 00020 float diff = num[i] - num[i-1]; 00021 //printf("difference:%f",diff); 00022 if(diff>0) 00023 { 00024 sign.push_back(1); 00025 } 00026 else if(diff<0) 00027 { 00028 sign.push_back(-1); 00029 } 00030 else 00031 { 00032 sign.push_back(0); 00033 } 00034 } 00035 /*do difference between the near sign 00036 *result < 0 save the max index 00037 *result > 0 save the min index 00038 */ 00039 vector<int> indMax; 00040 vector<int> indMin; 00041 vector<float> valMax; 00042 int cnt = 0; 00043 for(int j = 1;j<sign.size();j++) 00044 { 00045 int diff = sign[j]-sign[j-1]; 00046 if(diff<0) 00047 { 00048 indMax.push_back(j); 00049 } 00050 else if(diff>0) 00051 { 00052 indMin.push_back(j); 00053 } 00054 } 00055 //try to find the valid max (which the previous min is smaller than threshold) 00056 //begin with index 1 so there will have +1 or -1 error 00057 //for(int j = 1;j<indMax.size();j++) 00058 // { 00059 // 00060 // for(int i =0; i <indMin.size()-1; i++) 00061 // { 00062 // if(indMin[i]<indMax[j] && indMin[i+1]>indMax[j] && num[indMin[i]]< threshold) 00063 // { 00064 // cnt = cnt +1; 00065 // printf("found a valid max, index:%d, value: %f \n\r", indMax[j],num[indMax[j]]); 00066 // } 00067 // } 00068 // 00069 // } 00070 00071 for(int j = 0; j < indMax.size(); j++) 00072 { 00073 00074 if(num[indMax[j]] > height) 00075 { 00076 cnt = cnt +1; 00077 //push the max values into the vector 00078 valMax.push_back(num[indMax[j]]); 00079 } 00080 } 00081 00082 threshold = *min_element(valMax.begin(),valMax.end())* 0.3; 00083 00084 //printf("height in peak: %f\n\r", height); 00085 //printf("threshold: %f\n\r", threshold); 00086 00087 if(height > 0.1001) 00088 { 00089 threshold = min(threshold,height); 00090 //printf("I am in !!!!!!\n\r"); 00091 00092 } 00093 00094 threshold = max(threshold, 0.1); 00095 threshold = min(threshold, 0.2); 00096 height = threshold; 00097 return cnt; 00098 00099 //printf("num of max: %d\n\r", indMax.size()); 00100 //printf("num of step: %d\n\r", cnt); 00101 00102 // cout<<"max values:"<<endl; 00103 // for(int m = 0;m<indMax.size();m++) 00104 // { 00105 // printf("index:%d, value: %f ", indMax[m],num[indMax[m]]); 00106 // } 00107 // cout<<endl; 00108 // cout<<"min values:"<<endl; 00109 // for(int n = 0;n<indMin.size();n++) 00110 // { 00111 // cout<<num[indMin[n]]<<" "; 00112 // } 00113 // cout<<endl; 00114 } 00115
Generated on Wed Aug 17 2022 11:36:50 by
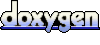