Lightning detector AS3935 library from arduino ported to mbed. Hardware I2C
Dependents: BLE_Lightning_sensor
AS3935.hpp
00001 /* 00002 AS3935.h - AS3935 Franklin Lightning Sensor™ IC by AMS library 00003 Copyright (c) 2012 Raivis Rengelis (raivis [at] rrkb.lv). All rights reserved. 00004 Portée sur MBED par Valentin 00005 00006 This library is free software; you can redistribute it and/or 00007 modify it under the terms of the GNU Lesser General Public 00008 License as published by the Free Software Foundation; either 00009 version 3 of the License, or (at your option) any later version. 00010 00011 This library is distributed in the hope that it will be useful, 00012 but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00014 Lesser General Public License for more details. 00015 00016 You should have received a copy of the GNU Lesser General Public 00017 License along with this library; if not, write to the Free Software 00018 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00019 */ 00020 00021 #ifndef _AS3935_HPP 00022 #define _AS3935_HPP 00023 00024 #include "mbed.h" 00025 //#include "i2c.hpp" 00026 00027 // register access macros - register address, bitmask 00028 #define AS3935_AFE_GB 0x00, 0x3E 00029 #define AS3935_PWD 0x00, 0x01 00030 #define AS3935_NF_LEV 0x01, 0x70 00031 #define AS3935_WDTH 0x01, 0x0F 00032 #define AS3935_CL_STAT 0x02, 0x40 00033 #define AS3935_MIN_NUM_LIGH 0x02, 0x30 00034 #define AS3935_SREJ 0x02, 0x0F 00035 #define AS3935_LCO_FDIV 0x03, 0xC0 00036 #define AS3935_MASK_DIST 0x03, 0x20 00037 #define AS3935_INT 0x03, 0x0F 00038 #define AS3935_DISTANCE 0x07, 0x3F 00039 #define AS3935_DISP_LCO 0x08, 0x80 00040 #define AS3935_DISP_SRCO 0x08, 0x40 00041 #define AS3935_DISP_TRCO 0x08, 0x20 00042 #define AS3935_TUN_CAP 0x08, 0x0F 00043 00044 // other constants 00045 #define AS3935_AFE_INDOOR 0x12 00046 #define AS3935_AFE_OUTDOOR 0x0E 00047 00048 class AS3935 { 00049 00050 public: 00051 /* 00052 * Initializes I2C interface and IRQ pin 00053 */ 00054 AS3935(PinName sda, PinName scl, int adresse); 00055 00056 //destruction 00057 //~AS3935(); 00058 00059 //write to specified register specified data using specified bitmask, 00060 //the rest of the register remains intact 00061 void registerWrite(char reg, char mask, char data); 00062 00063 //read specified register using specified bitmask and return value aligned 00064 //to lsb, i.e. if value to be read is in a middle of register, function 00065 //reads register and then aligns lsb of value to lsb of byte 00066 char registerRead(char reg, char mask); 00067 00068 //reset all the registers on chip to default values 00069 void reset(); 00070 00071 //put chip into power down mode 00072 void powerDown(); 00073 00074 //bring chip out of power down mode and perform RCO calibration 00075 void powerUp(); 00076 00077 //return interrupt source, bitmask, 0b0001 - noise, 0b0100 - disturber, 00078 //0b1000 - lightning 00079 int interruptSource(); 00080 00081 //disable indication of disturbers 00082 void disableDisturbers(); 00083 00084 //enable indication of distrubers 00085 void enableDisturbers(); 00086 00087 //return number of lightnings that need to be detected in 17 minute period 00088 //before interrupt is issued 00089 int getMinimumLightnings(); 00090 00091 //set number of lightnings that need to be detected in 17 minute period 00092 //before interrupt is issued 00093 int setMinimumLightnings(int minlightning); 00094 00095 //return distance to lightning in kilometers, 1 means storm is overhead, 00096 //63 means it is too far to reliably calculate distance 00097 int lightningDistanceKm(); 00098 00099 // load gain preset to operate indoors 00100 void setIndoors(); 00101 00102 //load gain preset to operate outdoors 00103 void setOutdoors(); 00104 00105 //return noise floor setting - refer to datasheet for meaning and range 00106 int getNoiseFloor(); 00107 00108 //set noise floor setting 00109 int setNoiseFloor(int noisefloor); 00110 00111 //return spike rejection value - refer to datasheet for meaning and range 00112 int getSpikeRejection(); 00113 00114 //set spike rejection value 00115 int setSpikeRejection(int srej); 00116 00117 //return watchdog threshold value - refer to datasheet for meaning and range 00118 int getWatchdogThreshold(); 00119 00120 //set watchdog threshold value 00121 int setWatchdogThreshold(int wdth); 00122 00123 //return tune Capacity value 00124 int getTuneCap(); 00125 00126 //set tune Capacity value 00127 int setTuneCap(int cap); 00128 00129 //clear internal accumulated lightning statistics 00130 void clearStats(); 00131 00132 private: 00133 I2C i2c; 00134 //DigitalOut _irq; 00135 int _adress; 00136 char _rawRegisterRead(char reg); 00137 char _ffsz(char mask); 00138 }; 00139 00140 /* !_AS3935_HPP_ */ 00141 #endif
Generated on Sun Jul 31 2022 00:44:19 by
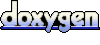