OSCtoCV Library
Embed:
(wiki syntax)
Show/hide line numbers
OSCtoCV_Sequencer.cpp
00001 /* 00002 OSCtoCV_Sequencer.cpp 00003 */ 00004 00005 #include "mbed.h" 00006 #include "OSCtoCV_Sequencer.h" 00007 #include "OSCtoCV.h" 00008 00009 //------------------------------------------------------------- 00010 // Sequence & Shift Out CV 00011 00012 void ShiftCVSeq(int trigger, bool reset, uint8_t channelRange) 00013 { 00014 int i, j; 00015 static bool triggerState = false; 00016 static bool stepFoward = false; 00017 static bool _reset = false; 00018 static uint8_t currentStep; 00019 static int resetCount; 00020 static uint8_t gateMode; 00021 static uint8_t _gateMode[16]; 00022 static uint8_t ch; 00023 static float glidecv[8], shiftcv[8]; 00024 unsigned int cv; 00025 static float qcv; 00026 static int jitter, jitterCount; 00027 00028 switch (CheckQuantizeMode()) 00029 { 00030 case Lin: 00031 00032 glidecv[0] = glidecv[0] * gSlide[currentStep] + gSeq_cv[currentStep] * (1.0f - gSlide[currentStep]); 00033 00034 break; 00035 00036 case Chr: 00037 00038 qcv = calibMap1[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES1 - 1))]; 00039 00040 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00041 00042 break; 00043 00044 case Maj: 00045 00046 qcv = calibMap2[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES2 - 1))]; 00047 00048 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00049 00050 break; 00051 00052 case M7: 00053 00054 qcv = calibMap3[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES3 - 1))]; 00055 00056 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00057 00058 break; 00059 00060 case Min7: 00061 00062 qcv = calibMap4[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES4 - 1))]; 00063 00064 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00065 00066 break; 00067 00068 case Dor: 00069 00070 qcv = calibMap5[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES5 - 1))]; 00071 00072 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00073 00074 break; 00075 00076 case Min: 00077 00078 qcv = calibMap6[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES6 - 1))]; 00079 00080 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00081 00082 break; 00083 00084 case S5th: 00085 00086 qcv = calibMap7[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES7 - 1))]; 00087 00088 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00089 00090 break; 00091 00092 case Wht: 00093 00094 qcv = calibMap8[(unsigned int)MapFloat(gSeq_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES8 - 1))]; 00095 00096 glidecv[0] = glidecv[0] * gSlide[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide[currentStep]); 00097 00098 break; 00099 } 00100 00101 CheckAnalogMode(); 00102 00103 if (!gCtrlSW[4]) 00104 { 00105 jitter = 0; 00106 00107 } else if (gCtrlSW[4] && jitterCount % 64 == 0) { // ASR Analog Mode 00108 00109 jitter = ANALOG_JITTER; 00110 } 00111 00112 cv = (unsigned int)(glidecv[0] + jitter); 00113 00114 UpdateCV(WRITE_UPDATE_N, 0, &cv); 00115 00116 for (i = 1; i < channelRange; ++i) 00117 { 00118 glidecv[i] = glidecv[i] * gSlide[currentStep] + shiftcv[i] * (1.0f - gSlide[currentStep]); 00119 cv = (unsigned int)(glidecv[i] + jitter); 00120 00121 UpdateCV(WRITE_UPDATE_N, i, &cv); 00122 } 00123 00124 if (trigger && !triggerState) // trigger ON 00125 { 00126 stepFoward = triggerState = true; 00127 00128 } else if (!trigger) { // trigger OFF 00129 00130 if (gateMode != HOLD) 00131 { 00132 gGATES[0] = false; 00133 } 00134 00135 triggerState = false; 00136 } 00137 00138 // check & update touchOSC ctrl parameter 00139 if (_gateMode[ch] != (gGateMode[ch] * 3)) 00140 { 00141 _gateMode[ch] = (gGateMode[ch] * 3); 00142 00143 if (_gateMode[ch] == MULTI) 00144 { 00145 _gateMode[ch] = HOLD; 00146 } 00147 00148 SendCtrlState(ch, _gateMode[ch], 8, SHIFTSEQ); 00149 } 00150 00151 if (reset && !_reset) // Stop & Reset 00152 { 00153 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, SHIFTSEQ)); 00154 sendMes.setArgs("i", 0); 00155 osc.sendOsc(&sendMes); 00156 00157 currentStep = 0; 00158 00159 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, SHIFTSEQ)); 00160 sendMes.setArgs("i", 1); 00161 osc.sendOsc(&sendMes); 00162 00163 _reset = true; 00164 stepFoward = triggerState = false; 00165 00166 } else if (!reset) { 00167 00168 _reset = false; 00169 } 00170 00171 if (stepFoward) 00172 { 00173 if (gateMode != HOLD) // shift CV 00174 { 00175 for (j = 1; j < 8; ++j) 00176 { 00177 shiftcv[j] = glidecv[j-1]; 00178 } 00179 } 00180 00181 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, SHIFTSEQ)); 00182 sendMes.setArgs("i", 0); 00183 osc.sendOsc(&sendMes); 00184 00185 ++currentStep; 00186 00187 if (gCtrlSW[2]) // 4step break 00188 { 00189 resetCount = 3; 00190 00191 } else { 00192 00193 resetCount = gCtrl[4] * 15; 00194 } 00195 00196 CheckResetCount(resetCount, SHIFTSEQ); 00197 00198 if (currentStep > resetCount) // reset 00199 { 00200 currentStep = 0; 00201 } 00202 00203 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, SHIFTSEQ)); 00204 sendMes.setArgs("i", 1); 00205 osc.sendOsc(&sendMes); 00206 00207 if (currentStep < 8) 00208 { 00209 UpdateCVMeter(currentStep, &cv); 00210 00211 } else { 00212 00213 UpdateCVMeter((currentStep - 8), &cv); 00214 } 00215 00216 gateMode = (gGateMode[currentStep] * 3); 00217 00218 if (gateMode == MULTI) // omit MULTI mode 00219 { 00220 gateMode = HOLD; 00221 } 00222 00223 if (gateMode != MUTE) 00224 { 00225 gGATES[0] = true; 00226 } 00227 00228 if (gAccent[currentStep]) // accent 00229 { 00230 gGATES[2] = true; 00231 00232 } else { 00233 00234 gGATES[2] = false; 00235 } 00236 00237 stepFoward = false; 00238 } 00239 00240 ++ch; 00241 ch &= 0x0F; 00242 00243 ++jitterCount; 00244 jitterCount &= 0x1FF; 00245 } 00246 00247 //------------------------------------------------------------- 00248 // M185 Sequencer 00249 00250 void M185Seq(int trigger, bool reset, uint8_t channelRange) 00251 { 00252 int i, j; 00253 static bool triggerState = false; 00254 static bool stepFoward = false; 00255 static bool _reset = false; 00256 static uint8_t currentStep; 00257 static int stepCount; 00258 static int resetCount; 00259 static uint8_t gateMode; 00260 static uint8_t _gateMode[8]; 00261 static uint8_t _pulseCount[8]; 00262 static uint8_t ch; 00263 static float glidecv[8], shiftcv[8]; 00264 unsigned int cv; 00265 static float qcv; 00266 static int jitterCount; 00267 static int jitter; 00268 00269 switch (CheckQuantizeMode()) 00270 { 00271 case Lin: 00272 00273 glidecv[0] = glidecv[0] * gSlide185[currentStep] + g185_cv[currentStep] * (1.0f - gSlide185[currentStep]); 00274 00275 break; 00276 00277 case Chr: 00278 00279 qcv = calibMap1[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES1 - 1))]; 00280 00281 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00282 00283 break; 00284 00285 case Maj: 00286 00287 qcv = calibMap2[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES2 - 1))]; 00288 00289 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00290 00291 break; 00292 00293 case M7: 00294 00295 qcv = calibMap3[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES3 - 1))]; 00296 00297 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00298 00299 break; 00300 00301 case Min7: 00302 00303 qcv = calibMap4[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES4 - 1))]; 00304 00305 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00306 00307 break; 00308 00309 case Dor: 00310 00311 qcv = calibMap5[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES5 - 1))]; 00312 00313 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00314 00315 break; 00316 00317 case Min: 00318 00319 qcv = calibMap6[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES6 - 1))]; 00320 00321 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00322 00323 break; 00324 00325 case S5th: 00326 00327 qcv = calibMap7[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES7 - 1))]; 00328 00329 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00330 00331 break; 00332 00333 case Wht: 00334 00335 qcv = calibMap8[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES8 - 1))]; 00336 00337 glidecv[0] = glidecv[0] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00338 00339 break; 00340 } 00341 00342 CheckAnalogMode(); 00343 00344 if (!gCtrlSW[4]) 00345 { 00346 jitter = 0; 00347 00348 } else if (gCtrlSW[4] && jitterCount % 64 == 0) { // ASR Analog Mode 00349 00350 jitter = ANALOG_JITTER; 00351 } 00352 00353 cv = (unsigned int)(glidecv[0] + jitter); 00354 00355 UpdateCV(WRITE_UPDATE_N, 0, &cv); 00356 00357 for (i = 1; i < channelRange; ++i) 00358 { 00359 glidecv[i] = glidecv[i] * gSlide185[currentStep] + shiftcv[i] * (1.0f - gSlide185[currentStep]); 00360 cv = (unsigned int)(glidecv[i] + jitter); 00361 00362 UpdateCV(WRITE_UPDATE_N, i, &cv); 00363 } 00364 00365 if (trigger && !triggerState) // trigger ON 00366 { 00367 if (gateMode == MULTI) 00368 { 00369 gGATES[0] = true; 00370 00371 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00372 sendMes.setArgs("i", 1); 00373 osc.sendOsc(&sendMes); 00374 } 00375 00376 stepFoward = triggerState = true; 00377 00378 } else if (!trigger) { // trigger OFF 00379 00380 if (gateMode != HOLD) 00381 { 00382 gGATES[0] = false; 00383 } 00384 00385 if (gateMode == MULTI) 00386 { 00387 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00388 sendMes.setArgs("i", 0); 00389 osc.sendOsc(&sendMes); 00390 } 00391 00392 triggerState = false; 00393 } 00394 00395 // check & update touchOSC ctrl parameter 00396 if (_gateMode[ch] != gGateMode185[ch] * 3 || _pulseCount[ch] != gPulseCount[ch] * 7) 00397 { 00398 _gateMode[ch] = (gGateMode185[ch] * 3); 00399 _pulseCount[ch] = (gPulseCount[ch] * 7); 00400 00401 SendCtrlState(ch, _gateMode[ch], _pulseCount[ch], M185SEQ); 00402 } 00403 00404 if (reset && !_reset) // Stop & Reset 00405 { 00406 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00407 sendMes.setArgs("i", 0); 00408 osc.sendOsc(&sendMes); 00409 00410 currentStep = 0; 00411 00412 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00413 sendMes.setArgs("i", 1); 00414 osc.sendOsc(&sendMes); 00415 00416 _reset = true; 00417 stepFoward = triggerState = false; 00418 00419 } else if (!reset) { 00420 00421 _reset = false; 00422 } 00423 00424 if (stepFoward) 00425 { 00426 if (gateMode != HOLD) // shift CV 00427 { 00428 for (j = 1; j < 8; ++j) 00429 { 00430 shiftcv[j] = glidecv[j-1]; 00431 } 00432 } 00433 00434 --stepCount; 00435 00436 if (stepCount == -1) 00437 { 00438 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00439 sendMes.setArgs("i", 0); 00440 osc.sendOsc(&sendMes); 00441 00442 ++currentStep; 00443 00444 if (gCtrlSW[2]) // 4step break 00445 { 00446 resetCount = 3; 00447 00448 } else { 00449 00450 resetCount = gCtrl[5] * 7; 00451 } 00452 00453 CheckResetCount(resetCount, M185SEQ); 00454 00455 if (currentStep > resetCount) // reset 00456 { 00457 currentStep = 0; 00458 } 00459 00460 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00461 sendMes.setArgs("i", 1); 00462 osc.sendOsc(&sendMes); 00463 00464 UpdateCVMeter(currentStep, &cv); 00465 00466 // check Pulse Count & Gate Mode 00467 stepCount = (gPulseCount[currentStep] * 7); 00468 00469 gateMode = (gGateMode185[currentStep] * 3); 00470 00471 if (gateMode != MUTE) 00472 { 00473 gGATES[0] = true; 00474 } 00475 00476 if (gAccent185[currentStep]) // accent 00477 { 00478 gGATES[2] = true; 00479 00480 } else { 00481 00482 gGATES[2] = false; 00483 } 00484 } 00485 00486 stepFoward = false; 00487 } 00488 00489 ++ch; 00490 ch &= 0x07; 00491 00492 ++jitterCount; 00493 jitterCount &= 0x1FF; 00494 } 00495 00496 //------------------------------------------------------------- 00497 // M185 Sequencer 00498 00499 void PolyM185Seq(int trigger, bool reset, uint8_t channelRange) 00500 { 00501 int i, j; 00502 static bool triggerState = false; 00503 static bool stepFoward = false; 00504 static bool _reset = false; 00505 static uint8_t currentStep; 00506 static int stepCount; 00507 static int resetCount; 00508 static uint8_t gateMode; 00509 static uint8_t _gateMode[8]; 00510 static uint8_t _pulseCount[8]; 00511 static uint8_t ch; 00512 static float glidecv[8], shiftcv[8]; 00513 unsigned int cv; 00514 static float qcv; 00515 static int jitterCount; 00516 static int jitter; 00517 00518 switch (CheckQuantizeMode()) 00519 { 00520 case Lin: 00521 00522 glidecv[4] = glidecv[4] * gSlide185[currentStep] + g185_cv[currentStep] * (1.0f - gSlide185[currentStep]); 00523 00524 break; 00525 00526 case Chr: 00527 00528 qcv = calibMap1[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES1 - 1))]; 00529 00530 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00531 00532 break; 00533 00534 case Maj: 00535 00536 qcv = calibMap2[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES2 - 1))]; 00537 00538 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00539 00540 break; 00541 00542 case M7: 00543 00544 qcv = calibMap3[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES3 - 1))]; 00545 00546 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00547 00548 break; 00549 00550 case Min7: 00551 00552 qcv = calibMap4[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES4 - 1))]; 00553 00554 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00555 00556 break; 00557 00558 case Dor: 00559 00560 qcv = calibMap5[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES5 - 1))]; 00561 00562 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00563 00564 break; 00565 00566 case Min: 00567 00568 qcv = calibMap6[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES6 - 1))]; 00569 00570 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00571 00572 break; 00573 00574 case S5th: 00575 00576 qcv = calibMap7[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES7 - 1))]; 00577 00578 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00579 00580 break; 00581 00582 case Wht: 00583 00584 qcv = calibMap8[(unsigned int)MapFloat(g185_cv[currentStep], 0, SCALING_N, 0, (QUAN_RES8 - 1))]; 00585 00586 glidecv[4] = glidecv[4] * gSlide185[currentStep] + (qcv * SCALING_N) * (1.0f - gSlide185[currentStep]); 00587 00588 break; 00589 } 00590 00591 CheckAnalogMode(); 00592 00593 if (!gCtrlSW[4]) 00594 { 00595 jitter = 0; 00596 00597 } else if (gCtrlSW[4] && jitterCount % 64 == 0) { // ASR Analog Mode 00598 00599 jitter = ANALOG_JITTER; 00600 } 00601 00602 cv = (unsigned int)(glidecv[4] + jitter); 00603 00604 UpdateCV(WRITE_UPDATE_N, 4, &cv); 00605 00606 for (i = 5; i < channelRange; ++i) 00607 { 00608 glidecv[i] = glidecv[i] * gSlide185[currentStep] + shiftcv[i] * (1.0f - gSlide185[currentStep]); 00609 cv = (unsigned int)(glidecv[i] + jitter); 00610 00611 UpdateCV(WRITE_UPDATE_N, i, &cv); 00612 } 00613 00614 if (trigger && !triggerState) // trigger ON 00615 { 00616 if (gateMode == MULTI) 00617 { 00618 gGATES[3] = true; 00619 00620 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00621 sendMes.setArgs("i", 1); 00622 osc.sendOsc(&sendMes); 00623 } 00624 00625 stepFoward = triggerState = true; 00626 00627 } else if (!trigger) { // trigger OFF 00628 00629 if (gateMode != HOLD) 00630 { 00631 gGATES[3] = false; 00632 } 00633 00634 if (gateMode == MULTI) 00635 { 00636 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00637 sendMes.setArgs("i", 0); 00638 osc.sendOsc(&sendMes); 00639 } 00640 00641 triggerState = false; 00642 } 00643 00644 // check & update touchOSC ctrl parameter 00645 if (_gateMode[ch] != gGateMode185[ch] * 3 || _pulseCount[ch] != gPulseCount[ch] * 7) 00646 { 00647 _gateMode[ch] = (gGateMode185[ch] * 3); 00648 _pulseCount[ch] = (gPulseCount[ch] * 7); 00649 00650 SendCtrlState(ch, _gateMode[ch], _pulseCount[ch], M185SEQ); 00651 } 00652 00653 if (reset && !_reset) // Stop & Reset 00654 { 00655 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00656 sendMes.setArgs("i", 0); 00657 osc.sendOsc(&sendMes); 00658 00659 currentStep = 0; 00660 00661 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00662 sendMes.setArgs("i", 1); 00663 osc.sendOsc(&sendMes); 00664 00665 _reset = true; 00666 stepFoward = triggerState = false; 00667 00668 } else if (!reset) { 00669 00670 _reset = false; 00671 } 00672 00673 if (stepFoward) 00674 { 00675 if (gateMode != HOLD) // shift CV 00676 { 00677 for (j = 5; j < 8; ++j) 00678 { 00679 shiftcv[j] = glidecv[j-1]; 00680 } 00681 } 00682 00683 --stepCount; 00684 00685 if (stepCount == -1) 00686 { 00687 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00688 sendMes.setArgs("i", 0); 00689 osc.sendOsc(&sendMes); 00690 00691 ++currentStep; 00692 00693 if (gCtrlSW[2]) // 4step break 00694 { 00695 resetCount = 3; 00696 00697 } else { 00698 00699 resetCount = gCtrl[5] * 7; 00700 } 00701 00702 CheckResetCount(resetCount, M185SEQ); 00703 00704 if (currentStep > resetCount) // reset 00705 { 00706 currentStep = 0; 00707 } 00708 00709 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, M185SEQ)); 00710 sendMes.setArgs("i", 1); 00711 osc.sendOsc(&sendMes); 00712 00713 // check Pulse Count & Gate Mode 00714 stepCount = (gPulseCount[currentStep] * 7); 00715 00716 gateMode = (gGateMode185[currentStep] * 3); 00717 00718 if (gateMode != MUTE) 00719 { 00720 gGATES[3] = true; 00721 } 00722 00723 } 00724 00725 stepFoward = false; 00726 } 00727 00728 ++ch; 00729 ch &= 0x07; 00730 00731 ++jitterCount; 00732 jitterCount &= 0x1FF; 00733 } 00734 00735 //------------------------------------------------------------- 00736 // shift cv seq ch1 ~ ch4 m185 seq ch5 ~ ch8 00737 00738 void PolyCVSeq(int trigger, bool reset) 00739 { 00740 ShiftCVSeq(trigger, reset, CV_CHANNEL4); 00741 PolyM185Seq(trigger, reset, CV_CHANNEL8); 00742 } 00743 00744 //------------------------------------------------------------- 00745 // 8ch drum track sequecer 00746 bool BeatsSeq(int trigger, bool reset, bool gatesOff) 00747 { 00748 int rndVel; 00749 static bool triggerState = false; 00750 static bool stepFoward = false; 00751 static bool _reset = false; 00752 static uint8_t currentStep; 00753 static int stepCount; 00754 static int resetCount; 00755 static uint8_t _pulseCount[8]; 00756 static uint8_t ch; 00757 static float decay[8] = {1}; 00758 00759 if (trigger && !triggerState) // trigger ON 00760 { 00761 if (stepCount != 0) 00762 { 00763 for (ch = 0; ch < 8; ++ch) 00764 { 00765 if (gBeatsMatrix[ch][currentStep]) 00766 { 00767 midi.sendNoteOn(0, 127, (ch + 1)); // volca sample trriger on 00768 00769 if (!gatesOff && ch < 4) 00770 { 00771 gGATES[ch] = true; 00772 } 00773 } 00774 } 00775 00776 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, BEATSSEQ)); 00777 sendMes.setArgs("i", 1); 00778 osc.sendOsc(&sendMes); 00779 } 00780 00781 stepFoward = triggerState = true; 00782 00783 } else if (!trigger) { // trigger OFF 00784 00785 if (!gatesOff) 00786 { 00787 gGATES[0] = gGATES[1] = gGATES[2] = gGATES[3] = false; 00788 } 00789 00790 if (stepCount != 0) 00791 { 00792 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, BEATSSEQ)); 00793 sendMes.setArgs("i", 0); 00794 osc.sendOsc(&sendMes); 00795 } 00796 00797 triggerState = false; 00798 } 00799 00800 // check & update touchOSC ctrl parameter 00801 for (ch = 0; ch < 16; ++ch) 00802 { 00803 if (_pulseCount[ch] != gPulseCountBeats[ch] * 7) 00804 { 00805 _pulseCount[ch] = (gPulseCountBeats[ch] * 7); 00806 00807 SendCtrlState(ch, 0, _pulseCount[ch], BEATSSEQ); 00808 } 00809 } 00810 00811 if (reset && !_reset) // Stop & Reset 00812 {// current step indeicator Reset 00813 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, BEATSSEQ)); 00814 sendMes.setArgs("i", 0); 00815 osc.sendOsc(&sendMes); 00816 00817 currentStep = 0; 00818 00819 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, BEATSSEQ)); 00820 sendMes.setArgs("i", 1); 00821 osc.sendOsc(&sendMes); 00822 00823 _reset = true; 00824 stepFoward = triggerState = false; 00825 00826 } else if (!reset) { 00827 00828 _reset = false; 00829 } 00830 00831 if (stepFoward) 00832 { 00833 --stepCount; 00834 00835 if (stepCount == -1) 00836 {// last current step indeicator ON 00837 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, BEATSSEQ)); 00838 sendMes.setArgs("i", 0); 00839 osc.sendOsc(&sendMes); 00840 00841 ++currentStep; 00842 00843 if (gCtrlSW[2]) // 4step break 00844 { 00845 resetCount = 3; 00846 00847 } else { 00848 00849 resetCount = gCtrl[7] * 15; 00850 } 00851 00852 CheckResetCount(resetCount, BEATSSEQ); 00853 00854 if (currentStep > resetCount) // reset 00855 { 00856 currentStep = 0; 00857 } 00858 00859 // current step indeicator ON 00860 sendMes.setTopAddress(SetMatrixAddress(0, currentStep, BEATSSEQ)); 00861 sendMes.setArgs("i", 1); 00862 osc.sendOsc(&sendMes); 00863 00864 // check Pulse Count & Gate Mode 00865 stepCount = (gPulseCountBeats[currentStep] * 7); 00866 00867 for (ch = 0; ch < 8; ++ch) 00868 { 00869 if (gBeatsMatrix[ch][currentStep]) 00870 { 00871 if (gCtrlSW[5]) //random Vel Mode /ctrlsw6 00872 { 00873 if (ch == 0 || ch == 2) 00874 { 00875 00876 rndVel = 95 - (rand() % 14); // random velocity ch1, ch3 00877 00878 } else if (ch == 1 || ch == 3) { 00879 00880 rndVel = 80 - (rand() % 10); // random velocity ch2, ch4 00881 00882 } else { 00883 00884 rndVel = 80; 00885 } 00886 00887 } else { 00888 00889 rndVel = 95; 00890 } 00891 00892 if (gBeatsMatrix[8][currentStep]) // ch9 accent Velocity +20 00893 { 00894 rndVel += 32; 00895 } 00896 00897 midi.sendControlChange(0x07, (gBeatsLevel[ch] * rndVel), (ch + 1)); // volca sample Level 00898 00899 if (decay[ch] != gBeatsDecay[ch]) 00900 { 00901 decay[ch] = gBeatsDecay[ch]; 00902 midi.sendControlChange(0x30, (decay[ch] * 127), (ch + 1)); // volca sample Amp EG Decay 00903 } 00904 00905 midi.sendNoteOn(0, 127, (ch + 1)); // volca sample trriger on 00906 00907 if (!gatesOff && ch < 4) 00908 { 00909 gGATES[ch] = true; 00910 } 00911 } 00912 } 00913 } 00914 00915 stepFoward = false; 00916 } 00917 00918 return triggerState; 00919 00920 } 00921 00922 //------------------------------------------------------------- 00923 // check reset count & send osc 00924 00925 void CheckResetCount(uint8_t resetCount, uint8_t mode) 00926 { 00927 static uint8_t _resetCountShift, _resetCount185, _resetCountBeats; 00928 00929 switch (mode) 00930 { 00931 case SHIFTSEQ: 00932 00933 if (_resetCountShift != resetCount) 00934 { 00935 sendMes.setTopAddress(RESET_COUNTER_ADDRESS); 00936 sendMes.setArgs("i", (resetCount + 1)); 00937 osc.sendOsc(&sendMes); 00938 00939 sendMes.setTopAddress("/ctrl5"); 00940 sendMes.setArgs("f", gCtrl[4]); 00941 osc.sendOsc(&sendMes); 00942 00943 _resetCountShift = resetCount; 00944 } 00945 break; 00946 00947 case M185SEQ: 00948 00949 if (_resetCount185 != resetCount) 00950 { 00951 sendMes.setTopAddress(RESET185_COUNTER_ADDRESS); 00952 sendMes.setArgs("i", (resetCount + 1)); 00953 osc.sendOsc(&sendMes); 00954 00955 sendMes.setTopAddress("/ctrl6"); 00956 sendMes.setArgs("f", gCtrl[5]); 00957 osc.sendOsc(&sendMes); 00958 00959 _resetCount185 = resetCount; 00960 } 00961 break; 00962 00963 case BEATSSEQ: 00964 00965 if (_resetCountBeats != resetCount) 00966 { 00967 sendMes.setTopAddress(BEATS_COUNTER_ADDRESS); 00968 sendMes.setArgs("i", (resetCount + 1)); 00969 osc.sendOsc(&sendMes); 00970 00971 sendMes.setTopAddress("/ctrl8"); 00972 sendMes.setArgs("f", gCtrl[7]); 00973 osc.sendOsc(&sendMes); 00974 00975 _resetCountBeats = resetCount; 00976 } 00977 break; 00978 00979 default: 00980 break; 00981 } 00982 00983 } 00984 00985 //------------------------------------------------------------- 00986 // check analog mode & send osc 00987 00988 inline void CheckAnalogMode() 00989 { 00990 static bool _analogMode; 00991 00992 if (_analogMode != gCtrlSW[4]) 00993 { 00994 sendMes.setTopAddress("/ctrlsw5"); 00995 sendMes.setArgs("i", gCtrlSW[4]); 00996 osc.sendOsc(&sendMes); 00997 00998 _analogMode = gCtrlSW[4]; 00999 } 01000 01001 } 01002 01003 //------------------------------------------------------------- 01004 // Send Sequencer Status to touchOSC 01005 01006 void SendCtrlState(uint8_t step, uint8_t gateMode, uint8_t stepCount, uint8_t mode) 01007 { 01008 char pulseAddress[10] = PULSE_COUNT_ADDRESS; 01009 char beatsPulseAddress[10] = BEATS_PULSE_COUNT_ADDRESS; 01010 char gateModeAddress[10] = GATE_MODE_ADDRESS; 01011 char gate185ModeAddress[10] = GATE185_MODE_ADDRESS; 01012 char currentStep[2]; 01013 01014 sprintf(currentStep, "%d", step + 1); 01015 01016 if(stepCount != 8) 01017 { 01018 if (mode == BEATSSEQ) 01019 { 01020 strcat(beatsPulseAddress, currentStep); 01021 sendMes.setTopAddress(beatsPulseAddress); 01022 01023 } else { 01024 01025 strcat(pulseAddress, currentStep); 01026 sendMes.setTopAddress(pulseAddress); 01027 } 01028 01029 sendMes.setArgs("i", (stepCount + 1)); 01030 osc.sendOsc(&sendMes); 01031 } 01032 01033 if (mode != BEATSSEQ) 01034 { 01035 switch (mode) 01036 { 01037 case SHIFTSEQ: 01038 01039 strcat(gateModeAddress, currentStep); 01040 sendMes.setTopAddress(gateModeAddress); 01041 break; 01042 01043 case M185SEQ: 01044 01045 strcat(gate185ModeAddress, currentStep); 01046 sendMes.setTopAddress(gate185ModeAddress); 01047 break; 01048 01049 default: 01050 break; 01051 } 01052 01053 switch (gateMode) 01054 { 01055 case SINGLE: 01056 01057 sendMes.setArgs("s", "|"); 01058 01059 break; 01060 01061 case MUTE: 01062 01063 sendMes.setArgs("s", "O"); 01064 01065 break; 01066 01067 case MULTI: 01068 01069 sendMes.setArgs("s", "||"); 01070 01071 break; 01072 01073 case HOLD: 01074 01075 sendMes.setArgs("s", "|-"); 01076 01077 break; 01078 } 01079 01080 osc.sendOsc(&sendMes); 01081 } 01082 01083 } 01084 01085
Generated on Tue Jul 12 2022 22:47:49 by
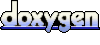