OSCtoCV Library
Embed:
(wiki syntax)
Show/hide line numbers
OSCtoCV_GateSequencer.cpp
00001 /* 00002 OSCtoCV_GateSequencer.cpp 00003 */ 00004 00005 #include "mbed.h" 00006 #include "OSCtoCV_GateSequencer.h" 00007 #include "OSCtoCV.h" 00008 00009 //------------------------------------------------------------- 00010 // Gate Sequencer beat(Note values) length(Gate time) invert(invert Gate) 00011 00012 int GateSeq(uint16_t bpm, uint16_t beat, uint8_t ch, float length, bool invert, bool gatesOff, bool syncoff) 00013 { 00014 int i; 00015 static int gatetime[GATE_TOTAL], oldgatetime[GATE_TOTAL]; 00016 static int _bpm, bar, sync24, oldsynctime; 00017 00018 int time = gTimer.read_us(); 00019 00020 if (_bpm != bpm) 00021 { 00022 if (!bpm) 00023 { 00024 beat = NRESET; 00025 00026 } else { 00027 00028 bar = (60.0f / bpm) * 4000000; 00029 //sync24 = (bar / 4) / 24; // sync24 not tested 00030 00031 _bpm = bpm; 00032 } 00033 } 00034 00035 switch (beat) // Calculate Note values 00036 { 00037 case NDOT2: 00038 00039 gatetime[ch] = (bar / 4) * 3; 00040 break; 00041 00042 case NDOT4: 00043 00044 gatetime[ch] = (bar / 8) * 3; 00045 break; 00046 00047 case NDOT8: 00048 00049 gatetime[ch] = (bar / 16) * 3; 00050 break; 00051 00052 case NDOT16: 00053 00054 gatetime[ch] = (bar / 32) * 3; 00055 break; 00056 00057 case NDOT32: 00058 00059 gatetime[ch] = (bar / 64) * 3; 00060 break; 00061 00062 case NRESET: 00063 00064 gTimer.reset(); 00065 00066 for (i = 0; i < GATE_TOTAL; ++i) // Reset 00067 { 00068 oldsynctime = oldgatetime[i] = gatetime[i] = NRESET; 00069 } 00070 00071 return 0; 00072 00073 default: 00074 00075 gatetime[ch] = bar / beat; 00076 sync24 = bar / 16; 00077 break; 00078 } 00079 00080 if (time > oldsynctime + sync24) // sync24 not tested 00081 { 00082 if (!syncoff) 00083 { 00084 oldsynctime = time; 00085 gCLOCKOUT = true; 00086 00087 midi.sendRealTime(Clock); // MIDI Clock 00088 } 00089 00090 } else if (time > oldsynctime - (sync24 - 2)) { 00091 00092 if (!syncoff) 00093 { 00094 gCLOCKOUT = false; 00095 } 00096 } 00097 00098 if (ch == GATE_TOTAL) 00099 { 00100 return -1; 00101 00102 } else if (time > oldgatetime[ch] + gatetime[ch] && !invert) { 00103 00104 oldgatetime[ch] = time; 00105 00106 if (!gatesOff) 00107 { 00108 gGATES[ch] = true; 00109 00110 } else if (ch == SUBGATE) { 00111 00112 gSUBGATE = true; 00113 } 00114 00115 return 1; 00116 00117 } else if (time > oldgatetime[ch] + gatetime[ch] && invert) { 00118 00119 oldgatetime[ch] = time; 00120 00121 if (!gatesOff) 00122 { 00123 gGATES[ch] = false; 00124 00125 } else if (ch == SUBGATE) { 00126 00127 gSUBGATE = false; 00128 } 00129 00130 return 0; 00131 00132 } else if (time > oldgatetime[ch] + (gatetime[ch] - gatetime[ch] / length) && !invert) { 00133 00134 if (!gatesOff) 00135 { 00136 gGATES[ch] = false; 00137 00138 } else if (ch == SUBGATE) { 00139 00140 gSUBGATE = false; 00141 } 00142 00143 return 0; 00144 00145 } else if (time > oldgatetime[ch] + (gatetime[ch] - gatetime[ch] / length) && invert) { 00146 00147 if (!gatesOff) 00148 { 00149 gGATES[ch] = true; 00150 00151 } else if (ch == SUBGATE) { 00152 00153 gSUBGATE = true; 00154 } 00155 00156 return 1; 00157 00158 } else { 00159 00160 return -1; 00161 } 00162 }
Generated on Tue Jul 12 2022 22:47:49 by
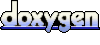