OSCtoCV Library
Embed:
(wiki syntax)
Show/hide line numbers
OSCtoCV_Euclidean.cpp
00001 /* 00002 OSCtoCV_Euclidean.cpp 00003 */ 00004 00005 #include "mbed.h" 00006 #include "OSCtoCV_Euclidean.h" 00007 #include "OSCtoCV.h" 00008 00009 00010 //------------------------------------------------------------- 00011 // Global Variables 00012 00013 int channels = MAXCHANNELS; 00014 unsigned int beat_holder[MAXCHANNELS]; 00015 unsigned int channelbeats[MAXCHANNELS][5]; 00016 00017 bool pulses_active = false; // is active while a beat pulse is playing 00018 bool lights_active = false; 00019 int pulse_length = TRIGGER_DURATION; //pulse length 00020 00021 unsigned int last_read[MAXCHANNELS]; 00022 unsigned int last_changed[MAXCHANNELS]; 00023 unsigned int last_sync; 00024 00025 unsigned int euc_time; 00026 00027 int masterclock; 00028 00029 //------------------------------------------------------------- 00030 // Euclidean Sequencer 00031 00032 unsigned int EuclideanSeq(int trigger, bool reset, bool gatesoff, bool auto_offset) { 00033 /* 00034 What's in the loop: 00035 Update euc_time variable 00036 Check to see if it is euc_time go go to sleep 00037 Changes routine - update beat_holder when channelbeats changes - triggered by changes == true 00038 Trigger routines - on trigget update displays and pulse 00039 Read encoders 00040 Read switches 00041 */ 00042 00043 int offset; 00044 static uint8_t nn, kk, oo; 00045 static unsigned int cnt_auto_offset; 00046 static uint8_t changes[MAXCHANNELS] = {0}; 00047 static int nknob, kknob, oknob; 00048 static int _nknob, _kknob, _oknob; 00049 static bool triggerState = false; 00050 00051 uint8_t i, ch; 00052 uint8_t maxn = MAXSTEPS; // maximums and minimums for n and k 00053 uint8_t minn = 1; 00054 uint8_t mink = 1; 00055 uint8_t mino = 0; 00056 00057 static uint8_t active_channel; 00058 00059 euc_time = gTimer.read_ms(); 00060 00061 nn = channelbeats[active_channel][0]; 00062 kk = channelbeats[active_channel][1]; 00063 oo = channelbeats[active_channel][3]; 00064 00065 // Stop & Reset (gCtrlSW[0]) 00066 if (reset) { 00067 00068 for (ch = 0; ch < channels; ++ch) { 00069 00070 for (i = 0; i < MAXSTEPS; ++i) { 00071 00072 for (ch = 0; ch < channels; ++ch) { 00073 sendMes.setTopAddress(SetMatrixAddress(ch * 2 + 1, i, EUCLID)); // ch * 2 + 1 00074 sendMes.setArgs("i", 0); 00075 osc.sendOsc(&sendMes); 00076 } 00077 00078 } 00079 00080 } 00081 00082 masterclock = cnt_auto_offset = active_channel = channelbeats[ch][2] = 0; 00083 00084 return 0; 00085 } 00086 00087 // UPDATE BEAT HOLDER WHEN KNOBS ARE MOVED 00088 if (changes[active_channel]) { 00089 00090 beat_holder[active_channel] = Euclid(nn, kk, oo); 00091 00092 switch (changes[active_channel]) 00093 { 00094 case 1: 00095 case 3: 00096 for (int i = 0; i < MAXSTEPS; ++i) { 00097 00098 if (BitRead(beat_holder[active_channel], nn - 1 - i) && (i < nn)) { 00099 sendMes.setTopAddress(SetMatrixAddress(active_channel * 2, i, EUCLID)); 00100 sendMes.setArgs("i", 1); 00101 osc.sendOsc(&sendMes); 00102 00103 } else { 00104 00105 sendMes.setTopAddress(SetMatrixAddress(active_channel * 2, i, EUCLID)); 00106 sendMes.setArgs("i", 0); 00107 osc.sendOsc(&sendMes); 00108 } 00109 } 00110 00111 break; 00112 00113 case 2: 00114 for (int i = 0; i < MAXSTEPS; ++i) { 00115 00116 if (i < nn) { 00117 sendMes.setTopAddress(SetMatrixAddress(active_channel * 2, i, EUCLID)); 00118 sendMes.setArgs("i", 1); 00119 osc.sendOsc(&sendMes); 00120 00121 } else { 00122 00123 sendMes.setTopAddress(SetMatrixAddress(active_channel * 2, i, EUCLID)); 00124 sendMes.setArgs("i", 0); 00125 osc.sendOsc(&sendMes); 00126 } 00127 } 00128 00129 break; 00130 00131 default: 00132 break; 00133 } 00134 00135 changes[active_channel] = 0; 00136 last_changed[active_channel] = gTimer.read_ms(); 00137 } 00138 00139 // ANALOG PULSE TRIGGER 00140 if (trigger && !triggerState) { 00141 00142 Sync(active_channel, gatesoff); 00143 triggerState = true; 00144 00145 if (auto_offset) { 00146 00147 cnt_auto_offset++; 00148 00149 if (cnt_auto_offset == 64) // auto offset 00150 { 00151 cnt_auto_offset = 0; 00152 00153 for (i = 0; i < MAXCHANNELS; ++i) 00154 { 00155 offset = (MAXSTEPS / 2) - (rand() % (MAXSTEPS - 1)); 00156 00157 if ((oo + offset) > (nn - 1)) { 00158 00159 offset = 0; 00160 oo = (nn - 1); 00161 00162 } else if ((oo + offset) < mino) { 00163 00164 offset = 0; 00165 oo = mino; 00166 } 00167 00168 channelbeats[i][3] = (oo + offset); 00169 00170 last_read[i] = gTimer.read_ms(); 00171 changes[i] = 3; // o change = 3 00172 } 00173 } 00174 00175 } 00176 00177 00178 } else if (!trigger) { 00179 00180 triggerState = false; 00181 } 00182 00183 // READ K KNOB 00184 kknob = EncodeReadK(active_channel); 00185 00186 if (_kknob != kknob) { 00187 00188 _kknob = kknob; 00189 00190 if (kknob != 0 && (euc_time - last_read[active_channel] > READ_DELAY)) { 00191 00192 if ((kk + kknob) > nn) { 00193 00194 kknob = 0; 00195 kk = nn; 00196 00197 } else if ((kk + kknob) < mink) { 00198 00199 kknob = 0; 00200 kk = mink; 00201 }; 00202 00203 kk = channelbeats[active_channel][1] = (kk + kknob); // update with encoder reading 00204 00205 last_read[active_channel] = gTimer.read_ms(); 00206 changes[active_channel] = 1; // k change = 1 00207 } 00208 } 00209 00210 // READ N KNOB 00211 nknob = EncodeReadN(active_channel); 00212 00213 if (_nknob != nknob) { 00214 00215 _nknob = nknob; 00216 00217 if (nknob != 0 && (euc_time - last_read[active_channel] > READ_DELAY)) { 00218 00219 if ((nn + nknob) > maxn) { 00220 00221 nknob = 0; 00222 nn = maxn; 00223 00224 } else if ((nn + nknob) < minn) { 00225 00226 nknob = 0; 00227 nn = minn; 00228 }; 00229 00230 if (kk > (nn + nknob)) {// check if new n is lower than k + reduce K if it is 00231 channelbeats[active_channel][1] = (nn + nknob); 00232 }; 00233 00234 if (oo > (nn + nknob - 1)) {// check if new n is lower than o + reduce o if it is 00235 channelbeats[active_channel][3] = (nn + nknob - 1); 00236 }; 00237 00238 nn = channelbeats[active_channel][0] = (nn + nknob); // update with encoder reading 00239 oo = channelbeats[active_channel][3]; 00240 00241 last_read[active_channel] = gTimer.read_ms(); 00242 changes[active_channel] = 2; // n change = 2 00243 } 00244 00245 } 00246 00247 // READ O KNOB 00248 oknob = EncodeReadO(active_channel); 00249 00250 if (_oknob != oknob) { 00251 00252 _oknob = oknob; 00253 00254 if (oknob != 0 && (euc_time - last_read[active_channel] > READ_DELAY)) { 00255 // Sense check o encoder reading to prevent crashes 00256 00257 if ((oo + oknob) > (nn - 1)) { 00258 00259 oknob = 0; 00260 oo = (nn - 1); 00261 00262 } else if ((oo + oknob) < mino) { 00263 00264 oknob = 0; 00265 oo = mino; 00266 } 00267 00268 channelbeats[active_channel][3] = (oo + oknob); 00269 00270 last_read[active_channel] = gTimer.read_ms(); 00271 changes[active_channel] = 3; // o change = 3 00272 } 00273 00274 } 00275 00276 // ENABLE RESET BUTTON ** ADD FLASH RESET HERE *** 00277 if (gCtrlSW[1] && channelbeats[active_channel][2]) { 00278 00279 for (ch = 0; ch < channels; ++ch) { 00280 channelbeats[ch][2] = 0; 00281 } 00282 } 00283 00284 // TURN OFF ANY LIGHTS THAT ARE ON 00285 if ((euc_time - last_sync) > pulse_length && lights_active) { 00286 00287 for (ch = 0; ch < channels; ++ch) { 00288 sendMes.setTopAddress(SetMatrixAddress((MAXCHANNELS * 2), 3 - ch, EUCLID)); 00289 sendMes.setArgs("i", 0); 00290 osc.sendOsc(&sendMes); 00291 00292 sendMes.setTopAddress(SetMatrixAddress((MAXCHANNELS * 2), 5, EUCLID)); 00293 sendMes.setArgs("i", 0); 00294 osc.sendOsc(&sendMes); 00295 00296 } 00297 00298 lights_active = false; 00299 } 00300 00301 // FINISH ANY PULSES THAT ARE ACTIVE - PULSES LAST 1/4 AS LONG AS LIGHTS 00302 if (euc_time - last_sync > (pulse_length / 4) && pulses_active) { 00303 00304 for (ch = 0; ch < channels; ++ch) { 00305 00306 if (!gatesoff) 00307 { 00308 gGATES[ch] = false; 00309 gCLOCKOUT = false; 00310 //digitalWrite(sparepin, LOW); 00311 } 00312 } 00313 00314 pulses_active = false; 00315 } 00316 00317 ++active_channel; 00318 active_channel &= (channels - 1); 00319 00320 return triggerState; 00321 } 00322 00323 //------------------------------------------------------------- 00324 // Init Euclidean Sequencer 00325 00326 void InitEuclideanSeq(void) { 00327 // Initialize Euclid Sequencer 00328 channelbeats[0][0] = 16; 00329 channelbeats[0][1] = 8; 00330 channelbeats[0][2] = 0; 00331 channelbeats[0][3] = 0; 00332 00333 channelbeats[1][0] = 16; 00334 channelbeats[1][1] = 9; 00335 channelbeats[1][2] = 0; 00336 channelbeats[1][3] = 0; 00337 00338 channelbeats[2][0] = 16; 00339 channelbeats[2][1] = 7; 00340 channelbeats[2][2] = 0; 00341 channelbeats[2][3] = 0; 00342 00343 channelbeats[3][0] = 16; 00344 channelbeats[3][1] = 9; 00345 channelbeats[3][2] = 0; 00346 channelbeats[3][3] = 0; 00347 00348 for (int i = 0; i < channels; ++i) 00349 { 00350 beat_holder[i] = Euclid(channelbeats[i][0], channelbeats[i][1], channelbeats[i][3]); 00351 } 00352 } 00353 00354 00355 //------------------------------------------------------------- 00356 // Euclid calculation function 00357 00358 unsigned int Euclid(int n, int k, int o) { // inputs: n=total, k=beats, o = offset 00359 int pauses = (n - k); 00360 int pulses = k; 00361 int offset = o; 00362 int steps = n; 00363 int per_pulse = (pauses / k); 00364 int remainder = (pauses % pulses); 00365 unsigned int workbeat[n]; 00366 unsigned int outbeat; 00367 uint16_t outbeat2; 00368 int workbeat_count = n; 00369 int a_remainder, b_remainder; 00370 int groupa, groupb; 00371 int i, j; 00372 int trim_count; 00373 00374 for (i = 0; i < n; ++i) { // Populate workbeat with unsorted pulses and pauses 00375 00376 if (i < pulses) { 00377 00378 workbeat[i] = 1; 00379 00380 } else { 00381 00382 workbeat[i] = 0; 00383 } 00384 } 00385 00386 if (per_pulse > 0 && remainder < 2) { // Handle easy cases where there is no or only one remainer 00387 00388 for (i = 0; i < pulses; ++i) { 00389 00390 for (j = (workbeat_count - 1); j > (workbeat_count - per_pulse - 1); --j) { 00391 workbeat[i] = ConcatBin(workbeat[i], workbeat[j]); 00392 } 00393 00394 workbeat_count = (workbeat_count - per_pulse); 00395 00396 } 00397 00398 outbeat = 0; // Concatenate workbeat into outbeat - according to workbeat_count 00399 00400 for (i = 0; i < workbeat_count; ++i) { 00401 outbeat = ConcatBin(outbeat, workbeat[i]); 00402 } 00403 00404 00405 if (offset != 0) { 00406 00407 outbeat2 = BitReadOffset(offset, outbeat, steps); // Add offset to the step pattern 00408 00409 } else { 00410 00411 outbeat2 = outbeat; 00412 } 00413 00414 return outbeat2; 00415 00416 } else { 00417 00418 groupa = pulses; 00419 groupb = pauses; 00420 00421 while (groupb > 1) { //main recursive loop 00422 00423 if (groupa > groupb) { // more Group A than Group B 00424 00425 a_remainder = (groupa - groupb); // what will be left of groupa once groupB is interleaved 00426 trim_count = 0; 00427 00428 for (i = 0; i < (groupa - a_remainder); ++i) { //count through the matching sets of A, ignoring remaindered 00429 workbeat[i] = ConcatBin(workbeat[i], workbeat[workbeat_count - 1 - i]); 00430 ++trim_count; 00431 } 00432 00433 workbeat_count = (workbeat_count - trim_count); 00434 00435 groupa = groupb; 00436 groupb = a_remainder; 00437 00438 } else if (groupb > groupa) { // More Group B than Group A 00439 00440 b_remainder = (groupb - groupa); // what will be left of group once group A is interleaved 00441 trim_count = 0; 00442 00443 for (i = workbeat_count-1; i >= (groupa + b_remainder); --i) { //count from right back through the Bs 00444 workbeat[workbeat_count - i - 1] = ConcatBin(workbeat[workbeat_count - 1 - i], workbeat[i]); 00445 ++trim_count; 00446 } 00447 00448 workbeat_count = (workbeat_count - trim_count); 00449 groupb = b_remainder; 00450 00451 } else if (groupa == groupb) { // groupa = groupb 00452 00453 trim_count = 0; 00454 00455 for (i = 0; i < groupa; ++i) { 00456 workbeat[i] = ConcatBin(workbeat[i], workbeat[workbeat_count - 1 - i]); 00457 ++trim_count; 00458 } 00459 00460 workbeat_count = (workbeat_count - trim_count); 00461 groupb = 0; 00462 00463 } 00464 } 00465 00466 outbeat = 0; // Concatenate workbeat into outbeat - according to workbeat_count 00467 00468 for (i = 0; i < workbeat_count; ++i) { 00469 00470 outbeat = ConcatBin(outbeat, workbeat[i]); 00471 } 00472 00473 if (offset != 0) { 00474 00475 outbeat2 = BitReadOffset(offset, outbeat, steps); // Add offset to the step pattern 00476 00477 } else { 00478 00479 outbeat2 = outbeat; 00480 } 00481 00482 return outbeat2; 00483 } 00484 } 00485 00486 //------------------------------------------------------------- 00487 // Reads a bit of a number 00488 00489 int BitRead(uint16_t b, int bitPos) { 00490 int x; 00491 00492 x = b & (1 << bitPos); 00493 00494 return x == 0 ? 0 : 1; 00495 } 00496 00497 //------------------------------------------------------------- 00498 // Function to right rotate n by d bits 00499 00500 uint16_t BitReadOffset(int shift, uint16_t value, uint16_t pattern_length) { 00501 uint16_t mask = ((1 << pattern_length) - 1); 00502 value &= mask; 00503 00504 return ((value >> shift) | (value << (pattern_length - shift))) & mask; 00505 } 00506 00507 //------------------------------------------------------------- 00508 // Function to find the binary length of a number by counting bitwise 00509 00510 int findlength(unsigned int bnry) { 00511 bool lengthfound = false; 00512 int i; 00513 int length = 1; // no number can have a length of zero - single 0 has a length of one, but no 1s for the sytem to count 00514 00515 for (i = 32; i >= 0; i--) { 00516 00517 if ((BitRead(bnry, i)) && !lengthfound) { 00518 length = (i + 1); 00519 lengthfound = true; 00520 } 00521 00522 } 00523 00524 return length; 00525 } 00526 00527 //------------------------------------------------------------- 00528 // Function to concatenate two binary numbers bitwise 00529 00530 unsigned int ConcatBin(unsigned int bina, unsigned int binb) { 00531 int binb_len = findlength(binb); 00532 unsigned int sum = (bina << binb_len); 00533 00534 sum = sum | binb; 00535 00536 return sum; 00537 } 00538 00539 //------------------------------------------------------------- 00540 // routine triggered by each beat 00541 00542 void Sync(int active_channel, bool gatesoff) { 00543 int read_head, erase; 00544 int rand_vel, rand_len; 00545 int ch, i; 00546 00547 if (masterclock % 2 == 0) { 00548 sendMes.setTopAddress(SetMatrixAddress((MAXCHANNELS * 2), 7, EUCLID)); 00549 sendMes.setArgs("i", 1); 00550 osc.sendOsc(&sendMes); 00551 00552 } else { 00553 00554 sendMes.setTopAddress(SetMatrixAddress((MAXCHANNELS * 2), 7, EUCLID)); 00555 sendMes.setArgs("i", 0); 00556 osc.sendOsc(&sendMes); 00557 } 00558 00559 // Cycle through channels 00560 for (ch = 0; ch < channels; ++ch) { 00561 00562 read_head = (channelbeats[ch][0] - channelbeats[ch][2] - 1); 00563 00564 if (ch != active_channel || (euc_time - last_changed[active_channel]) > DISPLAY_UPDATE) { 00565 00566 if (channelbeats[ch][2] < MAXSTEPS) { 00567 00568 for (i = 0; i < MAXSTEPS; ++i) { 00569 00570 if (BitRead(beat_holder[ch],channelbeats[ch][0] - 1 - i) && i < channelbeats[ch][0]) { 00571 00572 sendMes.setTopAddress(SetMatrixAddress(ch * 2, i, EUCLID)); 00573 sendMes.setArgs("i", 1); 00574 osc.sendOsc(&sendMes); 00575 00576 } else { 00577 00578 sendMes.setTopAddress(SetMatrixAddress(ch * 2, i, EUCLID)); 00579 sendMes.setArgs("i", 0); 00580 osc.sendOsc(&sendMes); 00581 } 00582 00583 } 00584 } 00585 } 00586 00587 if (!masterclock) { 00588 00589 erase = MAXSTEPS - 1; 00590 00591 } else { 00592 00593 erase = masterclock - 1; 00594 } 00595 00596 sendMes.setTopAddress(SetMatrixAddress((ch * 2) + 1, erase, EUCLID)); 00597 sendMes.setArgs("i", 0); 00598 osc.sendOsc(&sendMes); 00599 00600 sendMes.setTopAddress(SetMatrixAddress((ch * 2) + 1, masterclock, EUCLID)); 00601 sendMes.setArgs("i", 1); 00602 osc.sendOsc(&sendMes); 00603 00604 // turn on pulses on channels where a beat is present 00605 if (BitRead(beat_holder[ch], read_head)) { 00606 00607 if (!gatesoff) 00608 { 00609 gGATES[ch] = true; // pulse out 00610 } 00611 00612 sendMes.setTopAddress(SetMatrixAddress((MAXCHANNELS * 2), 3 - ch, EUCLID)); 00613 sendMes.setArgs("i", 1); 00614 osc.sendOsc(&sendMes); 00615 00616 lights_active = pulses_active = true; 00617 00618 if (!ch || (ch == 2)) { 00619 00620 rand_vel = 127 - (rand() % 20); // random velocity ch1, ch3 00621 00622 } else { 00623 00624 rand_vel = 95 - (rand() % 10); // random velocity ch2, ch4 00625 } 00626 00627 rand_len = 127 - (rand() % 17); // random Amp EG Decay 00628 00629 midi.sendControlChange(0x07, rand_vel, (ch + 1)); // volca sample Vol 00630 midi.sendControlChange(0x30, rand_len, (ch + 1)); // volca sample Amp EG Decay 00631 midi.sendNoteOn(0, 127, (ch + 1)); // volca sample trriger on 00632 } 00633 00634 // send off pulses to spare output for the first channel 00635 if (!(BitRead(beat_holder[ch], read_head)) && !ch) { // only relates to first channel 00636 00637 if (!gatesoff) 00638 { 00639 gCLOCKOUT = true; 00640 } 00641 00642 sendMes.setTopAddress(SetMatrixAddress((MAXCHANNELS * 2), 5, EUCLID)); 00643 sendMes.setArgs("i", 1); 00644 osc.sendOsc(&sendMes); 00645 00646 lights_active = pulses_active = true; 00647 } 00648 00649 // move counter to next position, ready for next pulse 00650 ++channelbeats[ch][2]; 00651 00652 if ((channelbeats[ch][2]) >= (channelbeats[ch][0])) { 00653 channelbeats[ch][2] = 0; 00654 } 00655 } 00656 00657 ++masterclock; 00658 masterclock &= (MAXSTEPS - 1); 00659 00660 pulse_length = ((euc_time - last_sync) / 5); 00661 last_sync = euc_time; 00662 } 00663 00664 /* 3 functions to read each encoder 00665 returns +1, 0 or -1 dependent on direction 00666 Contains no internal debounce, so calls should be delayed 00667 */ 00668 00669 //------------------------------------------------------------- 00670 // Check Euclidean Seq N(length) Value 00671 00672 int EncodeReadN(int ch) { 00673 static float _enc[4]; 00674 int result = 0; 00675 00676 switch (ch) 00677 { 00678 case 0: 00679 00680 if (gEucA[0] == 0) { 00681 _enc[ch] = result = 0; 00682 00683 } else if (gEucA[0] < _enc[ch]) { 00684 result = -1; 00685 _enc[ch] = gEucA[0]; 00686 00687 } else if (gEucA[0] > _enc[ch]) { 00688 result = 1; 00689 _enc[ch] = gEucA[0]; 00690 } 00691 00692 break; 00693 00694 case 1: 00695 00696 if (gEucA[3] == 0) { 00697 _enc[ch] = result = 0; 00698 00699 } else if (gEucA[3] < _enc[ch]) { 00700 result = -1; 00701 _enc[ch] = gEucA[3]; 00702 00703 } else if (gEucA[3] > _enc[ch]) { 00704 result = 1; 00705 _enc[ch] = gEucA[3]; 00706 } 00707 00708 break; 00709 00710 case 2: 00711 00712 if (gEucB[0] == 0) { 00713 _enc[ch] = result = 0; 00714 00715 } else if (gEucB[0] < _enc[ch]) { 00716 result = -1; 00717 _enc[ch] = gEucB[0]; 00718 00719 } else if (gEucB[0] > _enc[ch]) { 00720 result = 1; 00721 _enc[ch] = gEucB[0]; 00722 } 00723 00724 break; 00725 00726 case 3: 00727 00728 if (gEucB[3] == 0) { 00729 _enc[ch] = result = 0; 00730 00731 } else if (gEucB[3] < _enc[ch]) { 00732 result = -1; 00733 _enc[ch] = gEucB[3]; 00734 00735 } else if (gEucB[3] > _enc[ch]) { 00736 result = 1; 00737 _enc[ch] = gEucB[3]; 00738 } 00739 00740 break; 00741 00742 default: 00743 break; 00744 } 00745 00746 return result; 00747 } 00748 00749 //------------------------------------------------------------- 00750 // Check Euclidean Seq K(Density) Value 00751 00752 int EncodeReadK(int ch) { 00753 static float _enc[4]; 00754 int result = 0; 00755 00756 switch (ch) 00757 { 00758 case 0: 00759 00760 if (gEucA[1] == 0) { 00761 _enc[ch] = result = 0; 00762 00763 } else if (gEucA[1] < _enc[ch]) { 00764 result = -1; 00765 _enc[ch] = gEucA[1]; 00766 00767 } else if (gEucA[1] > _enc[ch]) { 00768 result = 1; 00769 _enc[ch] = gEucA[1]; 00770 } 00771 00772 break; 00773 00774 case 1: 00775 00776 if (gEucA[4] == 0) { 00777 _enc[ch] = result = 0; 00778 00779 } else if (gEucA[4] < _enc[ch]) { 00780 result = -4; 00781 _enc[ch] = gEucA[4]; 00782 00783 } else if (gEucA[4] > _enc[ch]) { 00784 result = 4; 00785 _enc[ch] = gEucA[4]; 00786 } 00787 00788 break; 00789 00790 case 2: 00791 00792 if (gEucB[1] == 0) { 00793 _enc[ch] = result = 0; 00794 00795 } else if (gEucB[1] < _enc[ch]) { 00796 result = -1; 00797 _enc[ch] = gEucB[1]; 00798 00799 } else if (gEucB[1] > _enc[ch]) { 00800 result = 1; 00801 _enc[ch] = gEucB[1]; 00802 } 00803 00804 break; 00805 00806 case 3: 00807 00808 if (gEucB[4] == 0) { 00809 _enc[ch] = result = 0; 00810 00811 } else if (gEucB[4] < _enc[ch]) { 00812 result = -1; 00813 _enc[ch] = gEucB[4]; 00814 00815 } else if (gEucB[4] > _enc[ch]) { 00816 result = 1; 00817 _enc[ch] = gEucB[4]; 00818 } 00819 00820 break; 00821 00822 default: 00823 break; 00824 } 00825 00826 return result; 00827 } 00828 00829 //------------------------------------------------------------- 00830 // Check Euclidean Seq O(Offset) Value 00831 00832 int EncodeReadO(int ch) { 00833 static float _enc[4]; 00834 int result = 0; 00835 00836 switch (ch) 00837 { 00838 case 0: 00839 00840 if (gEucA[2] == 0) { 00841 _enc[ch] = result = 0; 00842 00843 } else if (gEucA[2] < _enc[ch]) { 00844 result = -1; 00845 _enc[ch] = gEucA[2]; 00846 00847 } else if (gEucA[2] > _enc[ch]) { 00848 result = 1; 00849 _enc[ch] = gEucA[2]; 00850 } 00851 00852 break; 00853 00854 case 1: 00855 00856 if (gEucA[5] == 0) { 00857 _enc[ch] = result = 0; 00858 00859 } else if (gEucA[5] < _enc[ch]) { 00860 result = -1; 00861 _enc[ch] = gEucA[5]; 00862 00863 } else if (gEucA[5] > _enc[ch]) { 00864 result = 1; 00865 _enc[ch] = gEucA[5]; 00866 } 00867 00868 break; 00869 00870 case 2: 00871 00872 if (gEucB[2] == 0) { 00873 _enc[ch] = result = 0; 00874 00875 } else if (gEucB[2] < _enc[ch]) { 00876 result = -1; 00877 _enc[ch] = gEucB[2]; 00878 00879 } else if (gEucB[2] > _enc[ch]) { 00880 result = 1; 00881 _enc[ch] = gEucB[2]; 00882 } 00883 00884 break; 00885 00886 case 3: 00887 00888 if (gEucB[5] == 0) { 00889 _enc[ch] = result = 0; 00890 00891 } else if (gEucB[5] < _enc[ch]) { 00892 result = -1; 00893 _enc[ch] = gEucB[5]; 00894 00895 } else if (gEucB[5] > _enc[ch]) { 00896 result = 1; 00897 _enc[ch] = gEucB[5]; 00898 } 00899 00900 break; 00901 00902 default: 00903 break; 00904 } 00905 00906 return result; 00907 }
Generated on Tue Jul 12 2022 22:47:49 by
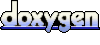