
CVtoOSC Converter for Nucleo F303K8
Dependencies: DebouncedInterrupt MIDI mbed osc-cnmat BufferedSerial
main.cpp
00001 /* 00002 CVtoOSC&MIDI Converter for Nucleo F303K8 00003 https://developer.mbed.org/platforms/ST-Nucleo-F303K8/ 00004 */ 00005 00006 #pragma O3 00007 #pragma Otime 00008 00009 #include <stdlib.h> 00010 #include <ctype.h> 00011 #include <math.h> 00012 #include <string> 00013 00014 #include "mbed.h" 00015 #include "BufferedSerial.h" 00016 #include "DebouncedInterrupt.h" 00017 #include "MIDI.h" 00018 #include "OSCBundle.h" 00019 00020 //------------------------------------------------------------- 00021 // Macros 00022 00023 #define MIDI_OFFSET 4 00024 #define MAP_OFFSET 0 00025 #define CALIB_OFFSET 37 00026 00027 #define QUAN_RES1 116 // Quantize voltage Steps 00028 #define QUAN_RES2 68 00029 #define QUAN_RES3 46 00030 #define QUAN_RES4 40 00031 #define QUAN_RES5 68 00032 #define QUAN_RES6 68 00033 #define QUAN_RES7 16 00034 #define QUAN_RES8 58 00035 00036 #define Lin 0 // Linear LinearCV 00037 #define Chr 1 // Chromatic 00038 #define Maj 2 // Major 00039 #define M7 3 // Major7 00040 #define Min7 4 // Minor7 00041 #define Dor 5 // Dorian 00042 #define Min 6 // Minor 00043 #define S5th 7 // 5th 00044 #define Wht 8 // Wholetone 00045 00046 #define SCALE_TOTAL 9 00047 00048 #define SEND_BUFF_SIZE 1024 // OSC send buffer size 00049 #define WAIT_SEND 20 // wait time(ms) for esp8266 transmission complete 00050 00051 #define MIDI_CHANNEL 1 // MIDI channel 00052 00053 //------------------------------------------------------------- 00054 // Global Variables 00055 00056 // Silentway Calibration Data Mapping 00057 // http://www.expert-sleepers.co.uk/silentway.html 00058 00059 // Chromatic Scale 00060 const float calibMap1[QUAN_RES1] = { 00061 0.00076928, 0.00900736, 0.01724544, 0.02548352, 0.03372160, 00062 0.04195968, 0.05019776, 0.05843584, 0.06667392, 0.07491200, 00063 0.08315008, 0.09138816, 0.09962624, 0.10786432, 0.11610240, 00064 0.12434047, 0.13258974, 0.14083999, 0.14909023, 0.15734047, 00065 0.16559070, 0.17384095, 0.18209119, 0.19034143, 0.19859168, 00066 0.20684192, 0.21509215, 0.22334240, 0.23159264, 0.23984288, 00067 0.24809311, 0.25634655, 0.26460093, 0.27285531, 0.28110969, 00068 0.28936407, 0.29761845, 0.30587283, 0.31412721, 0.32238159, 00069 0.33063596, 0.33889034, 0.34714472, 0.35539910, 0.36365348, 00070 0.37190786, 0.38017464, 0.38844886, 0.39672306, 0.40499726, 00071 0.41327149, 0.42154568, 0.42981988, 0.43809411, 0.44636831, 00072 0.45464250, 0.46291673, 0.47119093, 0.47946513, 0.48773935, 00073 0.49601355, 0.50430328, 0.51260746, 0.52091163, 0.52921581, 00074 0.53751999, 0.54582411, 0.55412829, 0.56243247, 0.57073665, 00075 0.57904083, 0.58734500, 0.59564912, 0.60395330, 0.61225748, 00076 0.62056166, 0.62890279, 0.63728637, 0.64566994, 0.65405351, 00077 0.66243708, 0.67082065, 0.67920423, 0.68758780, 0.69597137, 00078 0.70435494, 0.71273851, 0.72112209, 0.72950566, 0.73788923, 00079 0.74627280, 0.75476575, 0.76334614, 0.77192658, 0.78050703, 00080 0.78908741, 0.79766786, 0.80624831, 0.81482869, 0.82340914, 00081 0.83198959, 0.84056997, 0.84915042, 0.85773087, 0.86631125, 00082 0.87489170, 0.88425636, 0.89363104, 0.90300572, 0.91238040, 00083 0.92175508, 0.93112975, 0.94050443, 0.94987911, 0.95925385, 00084 0.96862853 00085 }; 00086 00087 // Major Scale 00088 const float calibMap2[QUAN_RES2] = { 00089 calibMap1[0], calibMap1[2], calibMap1[4], calibMap1[5], calibMap1[7], 00090 calibMap1[9], calibMap1[11], calibMap1[12], calibMap1[14], calibMap1[16], 00091 calibMap1[17], calibMap1[19], calibMap1[21], calibMap1[23], calibMap1[24], 00092 calibMap1[26], calibMap1[28], calibMap1[29], calibMap1[31], calibMap1[33], 00093 calibMap1[35], calibMap1[36], calibMap1[38], calibMap1[40], calibMap1[41], 00094 calibMap1[43], calibMap1[45], calibMap1[47], calibMap1[48], calibMap1[50], 00095 calibMap1[52], calibMap1[53], calibMap1[55], calibMap1[57], calibMap1[59], 00096 calibMap1[60], calibMap1[62], calibMap1[64], calibMap1[65], calibMap1[67], 00097 calibMap1[69], calibMap1[71], calibMap1[72], calibMap1[74], calibMap1[76], 00098 calibMap1[77], calibMap1[79], calibMap1[81], calibMap1[83], calibMap1[84], 00099 calibMap1[86], calibMap1[88], calibMap1[89], calibMap1[91], calibMap1[93], 00100 calibMap1[95], calibMap1[96], calibMap1[98], calibMap1[100], calibMap1[101], 00101 calibMap1[103], calibMap1[105], calibMap1[107], calibMap1[108], calibMap1[110], 00102 calibMap1[112], calibMap1[113], calibMap1[115] 00103 }; 00104 00105 // M7(9) 00106 const float calibMap3[QUAN_RES3] = { 00107 calibMap1[0], calibMap1[4], calibMap1[7], calibMap1[11], calibMap1[12], 00108 calibMap1[14], calibMap1[16], calibMap1[19], calibMap1[23], calibMap1[24], 00109 calibMap1[26], calibMap1[28], calibMap1[31], calibMap1[35], calibMap1[36], 00110 calibMap1[38], calibMap1[40], calibMap1[43], calibMap1[47], calibMap1[48], 00111 calibMap1[50], calibMap1[52], calibMap1[55], calibMap1[59], calibMap1[60], 00112 calibMap1[62], calibMap1[64], calibMap1[67], calibMap1[71], calibMap1[72], 00113 calibMap1[76], calibMap1[79], calibMap1[83], calibMap1[84], calibMap1[86], 00114 calibMap1[88], calibMap1[91], calibMap1[95], calibMap1[96], calibMap1[100], 00115 calibMap1[103], calibMap1[107], calibMap1[108], calibMap1[110], calibMap1[112], 00116 calibMap1[115] 00117 }; 00118 00119 // m7(9) 00120 const float calibMap4[QUAN_RES4] = { 00121 calibMap1[0], calibMap1[3], calibMap1[7], calibMap1[10], calibMap1[12], 00122 calibMap1[15], calibMap1[19], calibMap1[22], calibMap1[26], calibMap1[27], 00123 calibMap1[31], calibMap1[34], calibMap1[36], calibMap1[38], calibMap1[39], 00124 calibMap1[43], calibMap1[46], calibMap1[50], calibMap1[53], calibMap1[55], 00125 calibMap1[58], calibMap1[60], calibMap1[63], calibMap1[67], calibMap1[70], 00126 calibMap1[72], calibMap1[74], calibMap1[75], calibMap1[79], calibMap1[82], 00127 calibMap1[86], calibMap1[89], calibMap1[91], calibMap1[94], calibMap1[96], 00128 calibMap1[99], calibMap1[103], calibMap1[106], calibMap1[110], calibMap1[113] 00129 }; 00130 00131 // Dorian Scale 00132 const float calibMap5[QUAN_RES5] = { 00133 calibMap1[0], calibMap1[2], calibMap1[3], calibMap1[5], calibMap1[7], 00134 calibMap1[9], calibMap1[10], calibMap1[12], calibMap1[14], calibMap1[15], 00135 calibMap1[17], calibMap1[19], calibMap1[20], calibMap1[21], calibMap1[24], 00136 calibMap1[26], calibMap1[27], calibMap1[29], calibMap1[31], calibMap1[33], 00137 calibMap1[34], calibMap1[36], calibMap1[38], calibMap1[39], calibMap1[41], 00138 calibMap1[43], calibMap1[45], calibMap1[46], calibMap1[48], calibMap1[50], 00139 calibMap1[51], calibMap1[53], calibMap1[55], calibMap1[57], calibMap1[58], 00140 calibMap1[60], calibMap1[62], calibMap1[63], calibMap1[65], calibMap1[67], 00141 calibMap1[69], calibMap1[70], calibMap1[72], calibMap1[74], calibMap1[75], 00142 calibMap1[77], calibMap1[79], calibMap1[81], calibMap1[82], calibMap1[84], 00143 calibMap1[86], calibMap1[87], calibMap1[89], calibMap1[91], calibMap1[93], 00144 calibMap1[94], calibMap1[96], calibMap1[98], calibMap1[99], calibMap1[101], 00145 calibMap1[103], calibMap1[105], calibMap1[106], calibMap1[108], calibMap1[110], 00146 calibMap1[111], calibMap1[113], calibMap1[115] 00147 }; 00148 00149 // Minor Scale 00150 const float calibMap6[QUAN_RES6] = { 00151 calibMap1[0], calibMap1[2], calibMap1[3], calibMap1[5], calibMap1[7], 00152 calibMap1[8], calibMap1[10], calibMap1[12], calibMap1[14], calibMap1[15], 00153 calibMap1[17], calibMap1[19], calibMap1[20], calibMap1[22], calibMap1[24], 00154 calibMap1[26], calibMap1[27], calibMap1[29], calibMap1[31], calibMap1[32], 00155 calibMap1[34], calibMap1[36], calibMap1[38], calibMap1[39], calibMap1[41], 00156 calibMap1[43], calibMap1[44], calibMap1[46], calibMap1[48], calibMap1[50], 00157 calibMap1[51], calibMap1[53], calibMap1[55], calibMap1[56], calibMap1[58], 00158 calibMap1[60], calibMap1[62], calibMap1[63], calibMap1[65], calibMap1[67], 00159 calibMap1[68], calibMap1[70], calibMap1[72], calibMap1[74], calibMap1[75], 00160 calibMap1[77], calibMap1[79], calibMap1[80], calibMap1[82], calibMap1[84], 00161 calibMap1[86], calibMap1[87], calibMap1[89], calibMap1[91], calibMap1[92], 00162 calibMap1[94], calibMap1[96], calibMap1[98], calibMap1[99], calibMap1[101], 00163 calibMap1[103], calibMap1[104], calibMap1[106], calibMap1[108], calibMap1[110], 00164 calibMap1[111], calibMap1[113], calibMap1[115] 00165 }; 00166 00167 // 5th 00168 const float calibMap7[QUAN_RES7] = { 00169 calibMap1[0], calibMap1[7], calibMap1[14], calibMap1[21], calibMap1[28], 00170 calibMap1[35], calibMap1[42], calibMap1[49], calibMap1[56], calibMap1[63], 00171 calibMap1[70], calibMap1[77], calibMap1[84], calibMap1[91], calibMap1[98], 00172 calibMap1[105] 00173 }; 00174 00175 // Whole tone 00176 const float calibMap8[QUAN_RES8] = { 00177 calibMap1[0], calibMap1[1], calibMap1[2], calibMap1[6], calibMap1[8], 00178 calibMap1[10], calibMap1[12], calibMap1[14], calibMap1[16], calibMap1[18], 00179 calibMap1[20], calibMap1[22], calibMap1[24], calibMap1[26], calibMap1[28], 00180 calibMap1[30], calibMap1[32], calibMap1[34], calibMap1[36], calibMap1[38], 00181 calibMap1[40], calibMap1[42], calibMap1[44], calibMap1[46], calibMap1[48], 00182 calibMap1[50], calibMap1[52], calibMap1[54], calibMap1[56], calibMap1[58], 00183 calibMap1[60], calibMap1[62], calibMap1[64], calibMap1[66], calibMap1[68], 00184 calibMap1[70], calibMap1[72], calibMap1[74], calibMap1[76], calibMap1[78], 00185 calibMap1[80], calibMap1[82], calibMap1[84], calibMap1[86], calibMap1[88], 00186 calibMap1[90], calibMap1[92], calibMap1[94], calibMap1[96], calibMap1[98], 00187 calibMap1[100], calibMap1[102], calibMap1[104], calibMap1[106], calibMap1[108], 00188 calibMap1[110], calibMap1[112], calibMap1[114] 00189 }; 00190 00191 // Major Scale 00192 const uint8_t calibMapMidi2[QUAN_RES2] = { 00193 0, 2, 4, 5, 7, 9, 11, 00194 12, 14, 16, 17, 19, 21, 23, 00195 24, 26, 28, 29, 31, 33, 35, 00196 36, 38, 40, 41, 43, 45, 47, 00197 48, 50, 52, 53, 55, 57, 59, 00198 60, 62, 64, 65, 67, 69, 71, 00199 72, 74, 76, 77, 79, 81, 83, 00200 84, 86, 88, 89, 91, 93, 95, 00201 96, 98, 100, 101, 103, 105, 107, 00202 108, 110, 112, 113, 115 00203 }; 00204 00205 // M7(9) 00206 const uint8_t calibMapMidi3[QUAN_RES3] = { 00207 0, 4, 7, 11, 00208 12, 14, 16, 19, 23, 00209 24, 26, 28, 31, 35, 00210 36, 38, 40, 43, 47, 00211 48, 50, 52, 55, 59, 00212 60, 62, 64, 67, 71, 00213 72, 76, 79, 83, 00214 84, 86, 88, 91, 95, 00215 96, 100, 103, 107, 00216 108, 110, 112, 115 00217 }; 00218 00219 // m7(9) 00220 const uint8_t calibMapMidi4[QUAN_RES4] = { 00221 0, 3, 7, 10, 00222 12, 15, 19, 22, 00223 26, 27, 31, 34, 00224 36, 38, 39, 43, 46, 00225 50, 53, 55, 58, 00226 60, 63, 67, 70, 00227 72, 74, 75, 79, 82, 00228 86, 89, 91, 94, 00229 96, 99, 103, 106, 110, 113 00230 }; 00231 00232 // Dorian Scale 00233 const uint8_t calibMapMidi5[QUAN_RES5] = { 00234 0, 2, 3, 5, 7, 9, 10, 00235 12, 14, 15, 17, 19, 20, 21, 00236 24, 26, 27, 29, 31, 33, 34, 00237 36, 38, 39, 41, 43, 45, 46, 00238 48, 50, 51, 53, 55, 57, 58, 00239 60, 62, 63, 65, 67, 69, 70, 00240 72, 74, 75, 77, 79, 81, 82, 00241 84, 86, 87, 89, 91, 93, 94, 00242 96, 98, 99, 101, 103, 105, 106, 00243 108, 110, 111, 113, 115 00244 }; 00245 00246 // Minor Scale 00247 const uint8_t calibMapMidi6[QUAN_RES6] = { 00248 0, 2, 3, 5, 7, 8, 10, 00249 12, 14, 15, 17, 19, 20, 22, 00250 24, 26, 27, 29, 31, 32, 34, 00251 36, 38, 39, 41, 43, 44, 46, 00252 48, 50, 51, 53, 55, 56, 58, 00253 60, 62, 63, 65, 67, 68, 70, 00254 72, 74, 75, 77, 79, 80, 82, 00255 84, 86, 87, 89, 91, 92, 94, 00256 96, 98, 99, 101, 103, 104, 106, 00257 108, 110, 111, 113, 115 00258 }; 00259 00260 // 5th 00261 const uint8_t calibMapMidi7[QUAN_RES7] = { 00262 0, 7, 14, 21, 28, 35, 00263 42, 49, 56, 63, 70, 77, 00264 84, 91, 98, 105 00265 }; 00266 00267 // Whole tone 00268 const uint8_t calibMapMidi8[QUAN_RES8] = { 00269 0, 1, 2, 6, 8, 10, 00270 12, 14, 16, 18,20, 22, 00271 24, 26, 28, 30, 32, 34, 00272 36, 38, 40, 42, 44, 46, 00273 48, 50, 52, 54, 56, 58, 00274 60, 62, 64, 66, 68, 70, 00275 72, 74, 76, 78, 80, 82, 00276 84, 86, 88, 90, 92, 94, 00277 96, 98, 100, 102, 104, 106, 00278 108, 110, 112, 114 00279 }; 00280 00281 volatile bool changeFlag = false; 00282 00283 //------------------------------------------------------------- 00284 // mbed Functions 00285 /* 00286 //Nucleo F303K8 //MCP3204 00287 CS : pin 10 -- pin 8 (CS/SHDN) 00288 MOSI: pin 11 -- pin 9 (D in) 00289 MISO: pin 12 -- pin 10(D out) 00290 SCK : pin 13 -- pin 11(CLK) 00291 */ 00292 SPI spi(D11, D12, D13); 00293 DigitalOut csPin(D10); 00294 00295 AnalogIn scalePin(A0); // Scale Pin 00296 00297 AnalogIn potPin1(A1); // Pot1~2 00298 AnalogIn potPin2(D6); 00299 00300 DebouncedInterrupt switch1(D8); // SW1 00301 DebouncedInterrupt switch2(D9); // SW2 00302 00303 // esp8266 wi-fi settings 00304 #define SSID "SSID" 00305 #define PASSWORD "PASSWORD" 00306 00307 #define REMOTE_ADDRESS "192.168.1.2" 00308 #define REMOTE_PORT "8000" 00309 00310 #define STATIC_IP // if you use DHCP comment out this line 00311 00312 #define LOCAL_ADDRESS "192.168.1.8" 00313 #define LOCAL_PORT "9000" 00314 00315 // Serial for esp8266 (tx, rx) 00316 BufferedSerial esp8266Serial(D1, D0); 00317 00318 // Serial for debug (tx, rx) 00319 Serial pc(USBTX, USBRX); 00320 00321 // MIDI (tx, rx) 00322 MIDI midi(A7, NC); 00323 00324 // declare the OSC bundle 00325 OSCBundle bndl; 00326 00327 // Timer 00328 Timer timer; 00329 00330 //------------------------------------------------------------- 00331 // Functions 00332 00333 int map(int, int, int, int, int); 00334 uint16_t adcRead(uint8_t); 00335 void interruptSW1(); 00336 void interruptSW2(); 00337 void getESP8266Response(); 00338 void connectWifi(); 00339 00340 00341 //------------------------------------------------------------- 00342 // Remaps value from one range to another range. 00343 00344 inline int map(int x, int in_min, int in_max, int out_min, int out_max) 00345 { 00346 return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min; 00347 } 00348 00349 //------------------------------------------------------------- 00350 // Read MCP3208 AD Converter 00351 00352 uint16_t adcRead(uint8_t ch) { 00353 uint8_t b1, b2; 00354 uint8_t address = 0B01100000 | ((ch & 0B11) << 2); 00355 00356 csPin = 0; // CS pin Low 00357 00358 spi.write(address); 00359 b1 = spi.write(0x00); 00360 b2 = spi.write(0x00); 00361 00362 csPin = 1; // CS pin Hi 00363 00364 return (b1 << 4) | (b2 >> 4); 00365 } 00366 00367 //------------------------------------------------------------- 00368 // Check SW Status 00369 00370 void interruptSW1() { 00371 volatile static uint8_t sw1State; 00372 00373 if (!sw1State) { 00374 00375 sw1State = 1; 00376 00377 } else { 00378 00379 sw1State = 0; 00380 } 00381 00382 bndl.add("/sw1").add(sw1State); 00383 changeFlag = true; 00384 } 00385 00386 void interruptSW2() { 00387 volatile static uint8_t sw2State; 00388 00389 if (!sw2State) { 00390 00391 sw2State = 1; 00392 00393 } else { 00394 00395 sw2State = 0; 00396 } 00397 00398 bndl.add("/sw2").add(sw2State); 00399 changeFlag = true; 00400 } 00401 00402 //------------------------------------------------------------- 00403 // get & print esp8266 response 00404 00405 void getESP8266Response(int timeout) 00406 { 00407 char resBuff[256] = {0}; 00408 00409 timer.start(); 00410 00411 uint8_t i = 0; 00412 00413 while (!(timer.read() > timeout)) { 00414 00415 if (esp8266Serial.readable()) { 00416 00417 resBuff[i++] = esp8266Serial.getc(); 00418 } 00419 } 00420 00421 pc.printf(resBuff); 00422 00423 timer.stop(); 00424 timer.reset(); 00425 } 00426 00427 //------------------------------------------------------------- 00428 // Setup esp8266 wi-fi module 00429 00430 void connectWifi() { 00431 00432 pc.printf("\n---------- Starting ESP8266 Config ----------\r\n"); 00433 wait(2); 00434 00435 // set station mode 00436 pc.printf("\n---------- Set Station Mode ----------\r\n"); 00437 00438 esp8266Serial.printf("AT+CWMODE=1\r\n"); 00439 00440 getESP8266Response(1); 00441 wait(1); 00442 00443 // restart 00444 pc.printf("\n---------- Reset ESP8266 ----------\r\n"); 00445 00446 esp8266Serial.printf("AT+RST\r\n"); 00447 00448 getESP8266Response(2); 00449 wait(1); 00450 00451 #ifdef STATIC_IP 00452 00453 // set static IP 00454 pc.printf("\n---------- Setting Static IP ----------\r\n"); 00455 00456 esp8266Serial.printf("AT+CIPSTA=\""); 00457 esp8266Serial.printf(LOCAL_ADDRESS); 00458 esp8266Serial.printf("\"\r\n"); 00459 00460 getESP8266Response(3); 00461 wait(1); 00462 00463 #endif 00464 00465 // connect wifi 00466 pc.printf("\n---------- Wi-fi Connect ----------\r\n"); 00467 00468 esp8266Serial.printf("AT+CWJAP=\""); 00469 esp8266Serial.printf(SSID); 00470 esp8266Serial.printf("\",\""); 00471 esp8266Serial.printf(PASSWORD); 00472 esp8266Serial.printf("\"\r\n"); 00473 00474 getESP8266Response(3); 00475 wait(2); 00476 00477 // single connection 00478 pc.printf("\n---------- Setting Connection Mode ----------\r\n"); 00479 00480 esp8266Serial.printf("AT+CIPMUX=0\r\n"); 00481 00482 getESP8266Response(1); 00483 wait(1); 00484 00485 // set UDP connection 00486 pc.printf("\n---------- Setting UDP Connection ----------\r\n"); 00487 00488 esp8266Serial.printf("AT+CIPSTART="); 00489 esp8266Serial.printf("\"UDP\",\""); 00490 esp8266Serial.printf(REMOTE_ADDRESS); 00491 esp8266Serial.printf("\","); 00492 esp8266Serial.printf(REMOTE_PORT); 00493 esp8266Serial.printf(","); 00494 esp8266Serial.printf(LOCAL_PORT); 00495 esp8266Serial.printf(","); 00496 esp8266Serial.printf("0\r\n"); 00497 00498 getESP8266Response(3); 00499 wait(1); 00500 00501 // set data transmission mode 00502 pc.printf("\n---------- Setting Data Transmission Mode ----------\r\n"); 00503 00504 esp8266Serial.printf("AT+CIPMODE=1\r\n"); 00505 00506 getESP8266Response(1); 00507 wait(1); 00508 00509 // change Baud Rate 00510 pc.printf("\n---------- Change Baud Rate 4Mbps ----------\r\n"); 00511 00512 esp8266Serial.printf("AT+UART_CUR=4000000,8,1,0,0\r\n"); 00513 getESP8266Response(3); 00514 00515 esp8266Serial.baud(4000000); 00516 wait(1); 00517 00518 // ready to send data 00519 pc.printf("\n---------- Ready to Send Data ----------\r\n"); 00520 00521 esp8266Serial.printf("AT+CIPSEND\r\n"); 00522 00523 getESP8266Response(2); 00524 wait(1); 00525 } 00526 00527 //------------------------------------------------------------- 00528 // Main 00529 00530 int main() { 00531 00532 uint8_t i, idx; 00533 uint8_t oscBuff[SEND_BUFF_SIZE] = {0}; 00534 static uint8_t scale; 00535 uint8_t note[4] = {0}; 00536 static uint8_t _note[4]; 00537 uint16_t adc[4] = {0}; 00538 static uint16_t _adc[4]; 00539 float pot[2] = {0}; 00540 static float _pot[2] = {potPin1.read(), potPin2.read()}; 00541 00542 // Init SPI (4MHz clock) 00543 spi.format(8, 0); 00544 spi.frequency(4000000); 00545 csPin = 1; 00546 00547 // setup esp8266 wi-fi 00548 esp8266Serial.baud(115200); 00549 connectWifi(); 00550 00551 // start MIDI (ch1) 00552 midi.begin(MIDI_CHANNEL); 00553 00554 // start InterruptIn rising edge 00555 switch1.attach(&interruptSW1, IRQ_RISE, 10); 00556 switch2.attach(&interruptSW2, IRQ_RISE, 10); 00557 00558 // start timer (wait untile esp8266 transmission completed) 00559 timer.start(); 00560 00561 while (1) { 00562 00563 scale = map(scalePin.read_u16(), 0, 51890, 0, (SCALE_TOTAL - 1)); 00564 00565 for (i = 0; i < 4; ++i) { 00566 // read ADC 00567 adc[i] = adcRead(i); 00568 00569 if (abs(adc[i] - _adc[i]) > 10) { 00570 00571 changeFlag = true; 00572 00573 switch (i) { 00574 case 0: 00575 bndl.add("/acv1").add(adc[i]); 00576 break; 00577 case 1: 00578 bndl.add("/acv2").add(adc[i]); 00579 break; 00580 case 2: 00581 bndl.add("/acv3").add(adc[i]); 00582 break; 00583 case 3: 00584 bndl.add("/acv4").add(adc[i]); 00585 break; 00586 } 00587 } 00588 00589 if (abs(adc[i] - _adc[i]) > 31) { 00590 00591 // MIDI quantize 00592 switch (scale) { 00593 00594 case Lin: 00595 case Chr: 00596 note[i] = map(adc[i], 825, 3757, 0, (84 - MAP_OFFSET)) + CALIB_OFFSET; // C0(12) ~ C7(96) 00597 break; 00598 00599 case Maj: 00600 note[i] = calibMapMidi2[map(adc[i], 0, 4095, 0, QUAN_RES2 - 1)] + MIDI_OFFSET; 00601 break; 00602 00603 case M7: 00604 note[i] = calibMapMidi3[map(adc[i], 0, 4095, 0, QUAN_RES3 - 1)] + MIDI_OFFSET; 00605 break; 00606 00607 case Min7: 00608 note[i] = calibMapMidi4[map(adc[i], 0, 4095, 0, QUAN_RES4 - 1)] + MIDI_OFFSET; 00609 break; 00610 00611 case Dor: 00612 note[i] = calibMapMidi5[map(adc[i], 0, 4095, 0, QUAN_RES5 - 1)] + MIDI_OFFSET; 00613 break; 00614 00615 case Min: 00616 note[i] = calibMapMidi6[map(adc[i], 0, 4095, 0, QUAN_RES6 - 1)] + MIDI_OFFSET; 00617 break; 00618 00619 case S5th: 00620 note[i] = calibMapMidi7[map(adc[i], 0, 4095, 0, QUAN_RES7 - 1)] + MIDI_OFFSET; 00621 break; 00622 00623 case Wht: 00624 note[i] = calibMapMidi8[map(adc[i], 0, 4095, 0, QUAN_RES8 - 1)] + MIDI_OFFSET; 00625 break; 00626 00627 } 00628 00629 00630 if (_note[i] != note[i]) { 00631 00632 midi.sendNoteOff(_note[i], 0, MIDI_CHANNEL); 00633 midi.sendNoteOn(note[i], 100, MIDI_CHANNEL); 00634 00635 _note[i] = note[i]; 00636 } 00637 00638 } 00639 00640 _adc[i] = adc[i]; 00641 } 00642 00643 // check potentiometer value 00644 pot[0] = potPin1.read(); 00645 pot[1] = potPin2.read(); 00646 00647 if (fabs(pot[0] - _pot[0]) > 0.02f) { 00648 00649 bndl.add("/pot1").add(pot[0] * 1023.0f); 00650 midi.sendControlChange(0x16, map(potPin1.read_u16(), 0, 65535, 0, 127), MIDI_CHANNEL); // microKorg Noise Level 00651 00652 _pot[0] = pot[0]; 00653 changeFlag = true; 00654 00655 } else if (fabs(pot[1] - _pot[1]) > 0.02f) { 00656 00657 bndl.add("/pot2").add(pot[1] * 1023.0f); 00658 midi.sendControlChange(0x5E, map(potPin2.read_u16(), 0, 65535, 0, 127), MIDI_CHANNEL); // microKorg Delay Level 00659 00660 _pot[1] = pot[1]; 00661 changeFlag = true; 00662 } 00663 00664 if (changeFlag && timer.read_ms() > WAIT_SEND) { 00665 00666 idx = 0; 00667 int len = bndl.send(oscBuff); 00668 00669 while (len--) { 00670 // send OSC to esp8266 wifi 00671 esp8266Serial.putc(oscBuff[idx++]); 00672 } 00673 00674 changeFlag = false; 00675 bndl.empty(); // empty the bundle to free room for a new one 00676 00677 timer.reset(); 00678 } 00679 00680 } 00681 00682 }
Generated on Tue Jul 19 2022 11:37:29 by
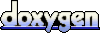