
striscia led
Dependencies: X_NUCLEO_LED61A1 mbed
Fork of HelloWorld_LED61A1 by
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @author Davide Aliprandi, STMicroelectronics 00005 * @version V1.0.0 00006 * @date February 4h, 2016 00007 * @brief mbed test application for the STMicroelectronics X-NUCLEO-LED61A1 00008 * LED expansion board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 00042 /* mbed specific header files. */ 00043 #include "mbed.h" 00044 00045 /* Component specific header files. */ 00046 #include "Led6001.h" 00047 00048 00049 /* Definitions ---------------------------------------------------------------*/ 00050 00051 /* PI. */ 00052 #ifndef M_PI 00053 #define M_PI (3.14159265358979323846f) 00054 #endif 00055 00056 /* Loop period in micro-seconds. */ 00057 #define LOOP_PERIOD_us (5E5) /* 0.5 seconds. */ 00058 00059 /* Sin period in micro-seconds. */ 00060 #define LED_SIN_PERIOD_us (1E7) /* 10 seconds. */ 00061 00062 00063 /* Variables -----------------------------------------------------------------*/ 00064 00065 /* Main loop's ticker. */ 00066 static Ticker ticker; 00067 00068 /* LED Control Component. */ 00069 Led6001 *led; 00070 00071 /* Interrupt flags. */ 00072 static volatile bool ticker_irq_triggered = false; 00073 static volatile bool xfault_irq_triggered = false; 00074 00075 00076 /* Functions -----------------------------------------------------------------*/ 00077 00078 /** 00079 * @brief Handling the LED capabilities. 00080 * @param None. 00081 * @retval None. 00082 */ 00083 void led_handler(void) 00084 { 00085 static int tick = 0; 00086 00087 /* Handling the LED dimming when powered ON. */ 00088 float dimming = 0.5f * sin(2 * M_PI * (tick++ * LOOP_PERIOD_us) / LED_SIN_PERIOD_us) + 0.5f; 00089 tick %= (int) (LED_SIN_PERIOD_us / LOOP_PERIOD_us); 00090 00091 /* Printing to the console. */ 00092 printf("Sinusoidal PWM Dimming --> %0.2f\r", dimming); 00093 00094 /* 00095 Writing PWM dimming values to the LED. 00096 00097 Notes: 00098 + Use "set_pwm_dimming()" for a PWM control, or "set_analog_dimming()" 00099 for an analog control. 00100 */ 00101 led->set_analog_dimming(dimming); 00102 } 00103 00104 /** 00105 * @brief Interrupt Request for the main loop's ticker related interrupt. 00106 * @param None. 00107 * @retval None. 00108 */ 00109 void ticker_irq(void) 00110 { 00111 ticker_irq_triggered = true; 00112 } 00113 00114 /** 00115 * @brief Interrupt Request for the component's XFAULT interrupt. 00116 * @param None. 00117 * @retval None. 00118 */ 00119 void xfault_irq(void) 00120 { 00121 xfault_irq_triggered = true; 00122 led->disable_xfault_irq(); 00123 } 00124 00125 /** 00126 * @brief Interrupt Handler for the component's XFAULT interrupt. 00127 * @param None. 00128 * @retval None. 00129 */ 00130 void xfault_handler(void) 00131 { 00132 /* Printing to the console. */ 00133 printf("XFAULT Interrupt detected! Re-initializing LED driver..."); 00134 00135 /* Re-starting-up LED Control Component. */ 00136 led->start_up(); 00137 00138 /* Printing to the console. */ 00139 printf("Done.\r\n\n"); 00140 00141 led->enable_xfault_irq(); 00142 } 00143 00144 00145 /* Main ----------------------------------------------------------------------*/ 00146 00147 int main() 00148 { 00149 /*----- Initialization. -----*/ 00150 00151 /* Printing to the console. */ 00152 printf("LED Control Application Example\r\n\n"); 00153 00154 /* Initializing LED Control Component. */ 00155 led = new Led6001(D4, A3, D6, D5); 00156 if (led->init() != COMPONENT_OK) { 00157 exit(EXIT_FAILURE); 00158 } 00159 00160 /* Attaching interrupt request functions. */ 00161 led->attach_xfault_irq(&xfault_irq); 00162 led->enable_xfault_irq(); 00163 ticker.attach_us(ticker_irq, LOOP_PERIOD_us); 00164 00165 /* Starting-up LED Control Component. */ 00166 led->start_up(); 00167 00168 00169 /*----- LED Control. -----*/ 00170 00171 /* Either performing the component handler, interrupt handlers, or waiting for events. */ 00172 while (true) { 00173 if (ticker_irq_triggered) { 00174 ticker_irq_triggered = false; 00175 led_handler(); 00176 } else if (xfault_irq_triggered) { 00177 xfault_irq_triggered = false; 00178 xfault_handler(); 00179 } else { 00180 /* It is recommended that SEVONPEND in the System Control Register is NOT set. */ 00181 __WFE(); 00182 } 00183 } 00184 }
Generated on Thu Jul 14 2022 15:28:51 by
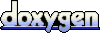