
Muestra texto en la matriz de LEDs
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Display de texto con scrolling 00002 // Basado en: https://github.com/hack-miniblip/hack-miniblip.github.io/tree/master/ejemplos/iluminandoLetras 00003 // Autores: @javacasm, @neon520, @juanAFernandez, @carlosgs 00004 00005 #include "mbed.h" 00006 #include "neopixel.h" 00007 00008 // Matrix led output pin 00009 #define DATA_PIN P0_9 00010 00011 void fill_pixel(neopixel::Pixel buffer[25], int x, int y, int red, int green, int blue){ 00012 00013 if(x<0) x=0; 00014 if(x>4) x=4; 00015 if(y<0) y=0; 00016 if(y>4) y=4; 00017 00018 00019 int posicion=x+y*5; 00020 buffer[posicion].red=red; 00021 buffer[posicion].green=green; 00022 buffer[posicion].blue=blue; 00023 00024 } 00025 00026 void void_matrix(neopixel::Pixel aux[25], int tam=25){ 00027 00028 for(int i=0;i<tam;i++){ 00029 aux[i].red=0; 00030 aux[i].green=0; 00031 aux[i].blue=0; 00032 } 00033 } 00034 00035 //r: intensidadRojo 00036 //g: intensidadVerde 00037 //b: intensidadAzul 00038 void generaLetra(neopixel::Pixel vector[], char letra, int red, int green, int blue, int scroll){ 00039 00040 /* 00041 * Cada letra tiene 5 valores en hexadecimal que representan en binario cada fila de la matrix 5x5 (aunque sobren 3 bits) 00042 */ 00043 unsigned char espacio[5]={0x0, 0x0, 0x0, 0x0, 0x0}; 00044 unsigned char a[5]={0x88, 0x88, 0xF8, 0x88, 0x70}; 00045 unsigned char b[5]={0xF0, 0x88, 0xF0, 0x88, 0xF0}; 00046 unsigned char c[5]={0x78, 0x80, 0x80, 0x80, 0x78}; 00047 unsigned char d[5]={0xF0, 0x88, 0x88, 0x88, 0xF0}; 00048 unsigned char e[5]={0xF8, 0x80, 0xF8, 0x80, 0xF8}; 00049 unsigned char f[5]={0x80, 0x80, 0xE0, 0x80, 0xF8}; 00050 unsigned char g[5]={0x78, 0x88, 0xB8, 0x80, 0x78}; 00051 unsigned char h[5]={0x88, 0x88, 0xF8, 0x88, 0x88}; 00052 unsigned char i[5]={0x70, 0x20, 0x20, 0x20, 0x70}; 00053 unsigned char j[5]={0x70, 0x88, 0x8, 0x8, 0x38}; 00054 unsigned char k[5]={0x88, 0x88, 0xF0, 0xA0, 0x90}; 00055 unsigned char l[5]={0xF8, 0x80, 0x80, 0x80, 0x80}; 00056 unsigned char m[5]={0x88, 0x88, 0xA8, 0xD8, 0x88}; 00057 unsigned char n[5]={0x98, 0xA8, 0xA8, 0xA8, 0xC8}; 00058 unsigned char gn[5]={0x98, 0xA8, 0xA8, 0xA8, 0xC8}; // es la ñ 00059 unsigned char o[5]={0x70, 0x88, 0x88, 0x88, 0x70}; 00060 unsigned char p[5]={0x80, 0x80, 0x78, 0x88, 0x78}; 00061 unsigned char q[5]={0x78, 0x90, 0x90, 0x90, 0x60}; 00062 unsigned char r[5]={0x88, 0x90, 0xF0, 0x88, 0xF0}; 00063 unsigned char s[5]={0xF0, 0x8, 0x70, 0x80, 0x78}; 00064 unsigned char t[5]={0x20, 0x20, 0x20, 0x20, 0xF8}; 00065 unsigned char u[5]={0x70,0x88,0x88,0x88,0x88}; 00066 unsigned char v[5]={0x20,0x50,0x50,0x88,0x88}; 00067 unsigned char w[5]={0x88,0xD8,0xA8,0x88,0x88}; 00068 unsigned char x[5]={0x88,0x50,0x20,0x50,0x88}; 00069 unsigned char y[5]={0x20,0x20,0x70,0x88,0x88}; 00070 unsigned char z[5]={0xF8,0x80,0x70,0x8,0xF8}; 00071 00072 00073 //unsigned char l0[0]= 00074 00075 00076 //Montamos un vector de vectores: 00077 unsigned char *vectorPunteros []={a, b, c, d, e, f, g, h, i, j, k, l, m, n, o, p, q, r, s, t, u, v, w, x, y, z}; 00078 00079 //Con la letra recibida le restamos 26 y tenemos la posicion del vector. 00080 //Para moverlo por los vectores. 00081 unsigned char *puntero; 00082 if (letra==32) //Se trata de un espacio 00083 puntero=espacio; 00084 else 00085 //a= al valor 97 00086 puntero=vectorPunteros[(letra-'a')]; 00087 00088 00089 //Vamos a recorrer todo el vector de bytes 00090 for(int i=0; i<5; i++){ 00091 00092 unsigned char elemento = puntero[i]; 00093 unsigned int mask=0x80; 00094 //Nos movemos por 5 bits de los 8 00095 for(int x=0; x<5; x++){ 00096 if (elemento & mask) 00097 if(x+scroll >= 0 && x+scroll < 5) 00098 fill_pixel(vector, x+scroll,i, red, green, blue); 00099 //Desplazamos 1 bit 00100 mask >>=1; 00101 } 00102 } 00103 } 00104 00105 void iluminaTexto(char cadena[], neopixel::PixelArray array, int r, int g, int b){ 00106 00107 //Creamos un vector de pixeles. 00108 neopixel::Pixel letra[25]; 00109 //Inicializamos el vector a 0 00110 void_matrix(letra); 00111 00112 char letraAnterior = ' '; 00113 00114 for(int i=0; i<strlen(cadena); i++){ 00115 00116 for(int scroll=0; scroll<7; scroll++){ 00117 //Generamos la letra en el vector letra 00118 generaLetra(letra, letraAnterior, r, g, b, -scroll-2); 00119 generaLetra(letra, cadena[i], r, g, b, 5-scroll); 00120 //Iluminamos la matriz 00121 array.update(letra, 25); 00122 //Esperamos 00123 wait_ms(60); 00124 //Reseteamos la matriz 00125 void_matrix(letra); 00126 } 00127 letraAnterior = cadena[i]; 00128 } 00129 00130 00131 } 00132 00133 PwmOut speaker(P0_8); 00134 00135 int main() 00136 { 00137 // Turn off miniblip buzzer 00138 speaker=0.0; 00139 00140 // Create a temporary DigitalIn so we can configure the pull-down resistor. 00141 DigitalIn(DATA_PIN, PullDown); 00142 00143 // The pixel array control class. 00144 //Se está creando un objeto de tipo PixelArray y se está nombrando como array a la que se le está pasando DATA_PIN 00145 neopixel::PixelArray array(DATA_PIN); 00146 00147 char greeting[] = "hola mundo "; 00148 00149 while (1) { 00150 int r = 0; 00151 int g = rand()%30; 00152 int b = rand()%30; 00153 00154 if (rand()%10 < 3){ 00155 r = rand()%30; 00156 g = 0; 00157 b = 0; 00158 } 00159 00160 iluminaTexto(greeting, array, r,g,b); 00161 wait_ms(500); 00162 } 00163 }
Generated on Thu Jul 14 2022 16:38:24 by
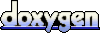