
tgtest
Dependencies: BufferedSerial Parameters mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 //#include "mbed_events.h" 00003 #include "rtos.h" 00004 #include "BufferedSerial.h" 00005 #include "Parameters.h" 00006 00007 00008 //------------------------------------ 00009 // Hyperterminal configuration 00010 // 9600 bauds, 8-bit data, no parity 00011 //------------------------------------ 00012 00013 Serial pc(SERIAL_TX, SERIAL_RX); 00014 BufferedSerial esp(D1,D0); 00015 00016 DigitalOut myled(LED1); 00017 DigitalOut led2(LED2); 00018 00019 //PwmOut servoPwm(D9); 00020 00021 //EventQueue queue(3 * EVENTS_EVENT_SIZE); 00022 Thread event_queue_thread; 00023 00024 00025 Thread esp_read_thread; 00026 void read_esp(); 00027 void check_serial1(); 00028 00029 Thread control_and_actuate_thread; 00030 void control_and_actuate(); 00031 00032 00033 00034 00035 int main() 00036 { 00037 esp.baud(115200); 00038 wait(0.1); 00039 esp_read_thread.start(control_and_actuate); 00040 wait(0.1); 00041 control_and_actuate_thread.start(control_and_actuate); 00042 wait(0.1); 00043 wait(0.1); 00044 wait(1); 00045 00046 int i = 1; 00047 while(1) { 00048 wait(1); 00049 char tmp = 't'; 00050 pc.printf("Main loop, %d , %c \r\n", i++, tmp); 00051 esp.printf("test\n"); 00052 myled = !myled; 00053 } 00054 } 00055 00056 void control_and_actuate() 00057 { 00058 //servoPwm.period(0.015f); 00059 //servoPwm.pulsewidth(0.0015f); 00060 00061 while(1) 00062 { 00063 00064 } 00065 } 00066 00067 void read_esp() 00068 { 00069 while(1) 00070 { 00071 //check_serial1(); 00072 } 00073 } 00074 00075 void check_serial1() 00076 { 00077 char c; 00078 static char param[20] = {""}; 00079 static char value[10] = {""}; 00080 static uint8_t value_index = 0; 00081 static uint8_t param_index = 0; 00082 static int count = 0; 00083 static enum State {READ_PARAM,READ_VALUE,SEARCH} state; 00084 while (esp.readable()) { 00085 // read the incoming byte: 00086 c = esp.getc(); 00087 switch (state) 00088 { 00089 case READ_PARAM: 00090 //pc.printf("State read param"); 00091 count++; 00092 if(c=='='){ 00093 count = 0; 00094 value[0] = '\0'; 00095 value_index = 0; 00096 state = READ_VALUE; 00097 } 00098 else if(c=='&') {count = 0;param[0] = '\0';} 00099 else 00100 { 00101 param[param_index] = c; 00102 param_index++; 00103 if (param_index >= 20) 00104 { 00105 error("Param buffer ovf"); 00106 param_index = 0; 00107 state = SEARCH; 00108 } 00109 param[param_index] = '\0'; 00110 } 00111 break; 00112 /* Reading value from serial *** */ 00113 case READ_VALUE: 00114 if(c=='=') { 00115 value[0] = '\0'; 00116 } 00117 else if(c=='&'){ 00118 pc.printf("Found param value:"); 00119 pc.printf(param);pc.printf(value); 00120 pms.try_write(param,value); 00121 } 00122 else 00123 { 00124 value[value_index++] = c; 00125 if (param_index >= 20) 00126 { 00127 error("Value buffer ovf"); 00128 param_index = 0; 00129 state = SEARCH; 00130 } 00131 value[value_index] = '\0'; 00132 } 00133 break; 00134 00135 /***** Searching for & *************/ 00136 case SEARCH: 00137 if(c=='&'){ 00138 param[0] = '\0'; 00139 param_index = 0; 00140 value[0] = '\0'; 00141 value_index = 0; 00142 state = READ_PARAM; 00143 } 00144 break; 00145 } 00146 pc.putc(c); 00147 } 00148 } 00149
Generated on Fri Jul 15 2022 13:40:34 by
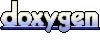