USB CDC library for MBED on STM32
Embed:
(wiki syntax)
Show/hide line numbers
usbd_desc.c
00001 /** 00002 ****************************************************************************** 00003 * @file Demonstrations/Src/usbd_desc.c 00004 * @author MCD Application Team 00005 * @brief This file provides the USBD descriptors and string formating method. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© Copyright (c) 2017 STMicroelectronics International N.V. 00010 * All rights reserved.</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without 00013 * modification, are permitted, provided that the following conditions are met: 00014 * 00015 * 1. Redistribution of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of other 00021 * contributors to this software may be used to endorse or promote products 00022 * derived from this software without specific written permission. 00023 * 4. This software, including modifications and/or derivative works of this 00024 * software, must execute solely and exclusively on microcontroller or 00025 * microprocessor devices manufactured by or for STMicroelectronics. 00026 * 5. Redistribution and use of this software other than as permitted under 00027 * this license is void and will automatically terminate your rights under 00028 * this license. 00029 * 00030 * THIS SOFTWARE IS PROVIDED BY STMICROELECTRONICS AND CONTRIBUTORS "AS IS" 00031 * AND ANY EXPRESS, IMPLIED OR STATUTORY WARRANTIES, INCLUDING, BUT NOT 00032 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A 00033 * PARTICULAR PURPOSE AND NON-INFRINGEMENT OF THIRD PARTY INTELLECTUAL PROPERTY 00034 * RIGHTS ARE DISCLAIMED TO THE FULLEST EXTENT PERMITTED BY LAW. IN NO EVENT 00035 * SHALL STMICROELECTRONICS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00036 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00037 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, 00038 * OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00039 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00040 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, 00041 * EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00042 * 00043 ****************************************************************************** 00044 */ 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "usbd_core.h" 00048 #include "usbd_desc.h" 00049 #include "usbd_conf.h" 00050 00051 /** @addtogroup STM32_USB_OTG_DEVICE_LIBRARY 00052 * @{ 00053 */ 00054 00055 00056 /** @defgroup USBD_DESC 00057 * @brief USBD descriptors module 00058 * @{ 00059 */ 00060 00061 /** @defgroup USBD_DESC_Private_TypesDefinitions 00062 * @{ 00063 */ 00064 /** 00065 * @} 00066 */ 00067 00068 00069 /** @defgroup USBD_DESC_Private_Defines 00070 * @{ 00071 */ 00072 00073 #define USBD_VID 0x0483 00074 #define USBD_PID 0x572B 00075 00076 #define USBD_LANGID_STRING 0x409 00077 #define USBD_MANUFACTURER_STRING "STMicroelectronics" 00078 00079 00080 #define USBD_PRODUCT_HS_STRING "CDC Virtual COM" 00081 #define USBD_SERIALNUMBER_HS_STRING "00000000001A" 00082 #define USBD_PRODUCT_FS_STRING "CDC Virtual COM" 00083 #define USBD_SERIALNUMBER_FS_STRING "00000000001B" 00084 #define USBD_CONFIGURATION_HS_STRING "CDC Config" 00085 #define USBD_INTERFACE_HS_STRING "CDC Interface" 00086 #define USBD_CONFIGURATION_FS_STRING "CDC Config" 00087 #define USBD_INTERFACE_FS_STRING "CDC Interface" 00088 /** 00089 * @} 00090 */ 00091 00092 00093 /** @defgroup USBD_DESC_Private_Macros 00094 * @{ 00095 */ 00096 /** 00097 * @} 00098 */ 00099 00100 00101 /** @defgroup USBD_DESC_Private_Variables 00102 * @{ 00103 */ 00104 00105 uint8_t * USBD_CDC_DeviceDescriptor( USBD_SpeedTypeDef speed , uint16_t *length); 00106 uint8_t * USBD_CDC_LangIDStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length); 00107 uint8_t * USBD_CDC_ManufacturerStrDescriptor ( USBD_SpeedTypeDef speed , uint16_t *length); 00108 uint8_t * USBD_CDC_ProductStrDescriptor ( USBD_SpeedTypeDef speed , uint16_t *length); 00109 uint8_t * USBD_CDC_SerialStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length); 00110 uint8_t * USBD_CDC_ConfigStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length); 00111 uint8_t * USBD_CDC_InterfaceStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length); 00112 00113 #ifdef USB_SUPPORT_USER_STRING_DESC 00114 uint8_t * USBD_CDC_USRStringDesc (USBD_SpeedTypeDef speed, uint8_t idx , uint16_t *length); 00115 #endif /* USB_SUPPORT_USER_STRING_DESC */ 00116 00117 00118 USBD_DescriptorsTypeDef USB_Desc = 00119 { 00120 USBD_CDC_DeviceDescriptor, 00121 USBD_CDC_LangIDStrDescriptor, 00122 USBD_CDC_ManufacturerStrDescriptor, 00123 USBD_CDC_ProductStrDescriptor, 00124 USBD_CDC_SerialStrDescriptor, 00125 USBD_CDC_ConfigStrDescriptor, 00126 USBD_CDC_InterfaceStrDescriptor, 00127 00128 }; 00129 00130 /* USB Standard Device Descriptor */ 00131 #if defined ( __ICCARM__ ) /*!< IAR Compiler */ 00132 #pragma data_alignment=4 00133 #endif 00134 __ALIGN_BEGIN uint8_t hUSBDDeviceDesc[USB_LEN_DEV_DESC] __ALIGN_END = 00135 { 00136 0x12, /*bLength */ 00137 USB_DESC_TYPE_DEVICE, /*bDescriptorType*/ 00138 0x10, /*bcdUSB */ 00139 0x01, 00140 0x00, /*bDeviceClass*/ 00141 0x00, /*bDeviceSubClass*/ 00142 0x00, /*bDeviceProtocol*/ 00143 USB_MAX_EP0_SIZE, /*bMaxPacketSize*/ 00144 LOBYTE(USBD_VID), /*idVendor*/ 00145 HIBYTE(USBD_VID), /*idVendor*/ 00146 LOBYTE(USBD_PID), /*idVendor*/ 00147 HIBYTE(USBD_PID), /*idVendor*/ 00148 0x00, /*bcdDevice rel. 1.00*/ 00149 0x01, 00150 USBD_IDX_MFC_STR, /*Index of manufacturer string*/ 00151 USBD_IDX_PRODUCT_STR, /*Index of product string*/ 00152 USBD_IDX_SERIAL_STR, /*Index of serial number string*/ 00153 USBD_MAX_NUM_CONFIGURATION /*bNumConfigurations*/ 00154 } ; /* USB_DeviceDescriptor */ 00155 00156 /* USB Standard Device Descriptor */ 00157 #if defined ( __ICCARM__ ) /*!< IAR Compiler */ 00158 #pragma data_alignment=4 00159 #endif 00160 __ALIGN_BEGIN uint8_t USBD_LangIDDesc[USB_LEN_LANGID_STR_DESC] __ALIGN_END = 00161 { 00162 USB_LEN_LANGID_STR_DESC, 00163 USB_DESC_TYPE_STRING, 00164 LOBYTE(USBD_LANGID_STRING), 00165 HIBYTE(USBD_LANGID_STRING), 00166 }; 00167 00168 #if defined ( __ICCARM__ ) /*!< IAR Compiler */ 00169 #pragma data_alignment=4 00170 #endif 00171 __ALIGN_BEGIN uint8_t USBD_StrDesc[USBD_MAX_STR_DESC_SIZ] __ALIGN_END; 00172 /** 00173 * @} 00174 */ 00175 00176 00177 /** @defgroup USBD_DESC_Private_FunctionPrototypes 00178 * @{ 00179 */ 00180 /** 00181 * @} 00182 */ 00183 00184 00185 /** @defgroup USBD_DESC_Private_Functions 00186 * @{ 00187 */ 00188 00189 /** 00190 * @brief USBD_CDC_DeviceDescriptor 00191 * return the device descriptor 00192 * @param speed : current device speed 00193 * @param length : pointer to data length variable 00194 * @retval pointer to descriptor buffer 00195 */ 00196 uint8_t * USBD_CDC_DeviceDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00197 { 00198 *length = sizeof(hUSBDDeviceDesc); 00199 return hUSBDDeviceDesc; 00200 } 00201 00202 /** 00203 * @brief USBD_CDC_LangIDStrDescriptor 00204 * return the LangID string descriptor 00205 * @param speed : current device speed 00206 * @param length : pointer to data length variable 00207 * @retval pointer to descriptor buffer 00208 */ 00209 uint8_t * USBD_CDC_LangIDStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00210 { 00211 *length = sizeof(USBD_LangIDDesc); 00212 return USBD_LangIDDesc; 00213 } 00214 00215 00216 /** 00217 * @brief USBD_CDC_ProductStrDescriptor 00218 * return the product string descriptor 00219 * @param speed : current device speed 00220 * @param length : pointer to data length variable 00221 * @retval pointer to descriptor buffer 00222 */ 00223 uint8_t * USBD_CDC_ProductStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00224 { 00225 if(speed == 0) 00226 { 00227 USBD_GetString ((uint8_t *)USBD_PRODUCT_HS_STRING, USBD_StrDesc, length); 00228 } 00229 else 00230 { 00231 USBD_GetString ((uint8_t *)USBD_PRODUCT_FS_STRING, USBD_StrDesc, length); 00232 } 00233 return USBD_StrDesc; 00234 } 00235 00236 /** 00237 * @brief USBD_CDC_ManufacturerStrDescriptor 00238 * return the manufacturer string descriptor 00239 * @param speed : current device speed 00240 * @param length : pointer to data length variable 00241 * @retval pointer to descriptor buffer 00242 */ 00243 uint8_t * USBD_CDC_ManufacturerStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00244 { 00245 USBD_GetString ((uint8_t *)USBD_MANUFACTURER_STRING, USBD_StrDesc, length); 00246 return USBD_StrDesc; 00247 } 00248 00249 /** 00250 * @brief USBD_CDC_SerialStrDescriptor 00251 * return the serial number string descriptor 00252 * @param speed : current device speed 00253 * @param length : pointer to data length variable 00254 * @retval pointer to descriptor buffer 00255 */ 00256 uint8_t * USBD_CDC_SerialStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00257 { 00258 if(speed == USBD_SPEED_HIGH) 00259 { 00260 USBD_GetString ((uint8_t *)USBD_SERIALNUMBER_HS_STRING, USBD_StrDesc, length); 00261 } 00262 else 00263 { 00264 USBD_GetString ((uint8_t *)USBD_SERIALNUMBER_FS_STRING, USBD_StrDesc, length); 00265 } 00266 return USBD_StrDesc; 00267 } 00268 00269 /** 00270 * @brief USBD_CDC_ConfigStrDescriptor 00271 * return the configuration string descriptor 00272 * @param speed : current device speed 00273 * @param length : pointer to data length variable 00274 * @retval pointer to descriptor buffer 00275 */ 00276 uint8_t * USBD_CDC_ConfigStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00277 { 00278 if(speed == USBD_SPEED_HIGH) 00279 { 00280 USBD_GetString ((uint8_t *)USBD_CONFIGURATION_HS_STRING, USBD_StrDesc, length); 00281 } 00282 else 00283 { 00284 USBD_GetString ((uint8_t *)USBD_CONFIGURATION_FS_STRING, USBD_StrDesc, length); 00285 } 00286 return USBD_StrDesc; 00287 } 00288 00289 00290 /** 00291 * @brief USBD_CDC_InterfaceStrDescriptor 00292 * return the interface string descriptor 00293 * @param speed : current device speed 00294 * @param length : pointer to data length variable 00295 * @retval pointer to descriptor buffer 00296 */ 00297 uint8_t * USBD_CDC_InterfaceStrDescriptor( USBD_SpeedTypeDef speed , uint16_t *length) 00298 { 00299 if(speed == 0) 00300 { 00301 USBD_GetString ((uint8_t *)USBD_INTERFACE_HS_STRING, USBD_StrDesc, length); 00302 } 00303 else 00304 { 00305 USBD_GetString ((uint8_t *)USBD_INTERFACE_FS_STRING, USBD_StrDesc, length); 00306 } 00307 return USBD_StrDesc; 00308 } 00309 00310 /** 00311 * @} 00312 */ 00313 00314 00315 /** 00316 * @} 00317 */ 00318 00319 00320 /** 00321 * @} 00322 */ 00323 00324 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00325 00326
Generated on Thu Jul 14 2022 15:47:45 by
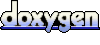