USB CDC library for MBED on STM32
Embed:
(wiki syntax)
Show/hide line numbers
usbd_def.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file usbd_def.h 00004 * @author MCD Application Team 00005 * @version V2.4.2 00006 * @date 11-December-2015 00007 * @brief General defines for the usb device library 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT 2015 STMicroelectronics</center></h2> 00012 * 00013 * Licensed under MCD-ST Liberty SW License Agreement V2, (the "License"); 00014 * You may not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at: 00016 * 00017 * http://www.st.com/software_license_agreement_liberty_v2 00018 * 00019 * Unless required by applicable law or agreed to in writing, software 00020 * distributed under the License is distributed on an "AS IS" BASIS, 00021 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00022 * See the License for the specific language governing permissions and 00023 * limitations under the License. 00024 * 00025 ****************************************************************************** 00026 */ 00027 00028 /* Define to prevent recursive inclusion -------------------------------------*/ 00029 #ifndef __USBD_DEF_H 00030 #define __USBD_DEF_H 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 /* Includes ------------------------------------------------------------------*/ 00037 #include "usbd_conf.h" 00038 00039 /** @addtogroup STM32_USBD_DEVICE_LIBRARY 00040 * @{ 00041 */ 00042 00043 /** @defgroup USB_DEF 00044 * @brief general defines for the usb device library file 00045 * @{ 00046 */ 00047 00048 /** @defgroup USB_DEF_Exported_Defines 00049 * @{ 00050 */ 00051 00052 #ifndef NULL 00053 #define NULL 0 00054 #endif 00055 00056 00057 #define USB_LEN_DEV_QUALIFIER_DESC 0x0A 00058 #define USB_LEN_DEV_DESC 0x12 00059 #define USB_LEN_CFG_DESC 0x09 00060 #define USB_LEN_IF_DESC 0x09 00061 #define USB_LEN_EP_DESC 0x07 00062 #define USB_LEN_OTG_DESC 0x03 00063 #define USB_LEN_LANGID_STR_DESC 0x04 00064 #define USB_LEN_OTHER_SPEED_DESC_SIZ 0x09 00065 00066 #define USBD_IDX_LANGID_STR 0x00 00067 #define USBD_IDX_MFC_STR 0x01 00068 #define USBD_IDX_PRODUCT_STR 0x02 00069 #define USBD_IDX_SERIAL_STR 0x03 00070 #define USBD_IDX_CONFIG_STR 0x04 00071 #define USBD_IDX_INTERFACE_STR 0x05 00072 00073 #define USB_REQ_TYPE_STANDARD 0x00 00074 #define USB_REQ_TYPE_CLASS 0x20 00075 #define USB_REQ_TYPE_VENDOR 0x40 00076 #define USB_REQ_TYPE_MASK 0x60 00077 00078 #define USB_REQ_RECIPIENT_DEVICE 0x00 00079 #define USB_REQ_RECIPIENT_INTERFACE 0x01 00080 #define USB_REQ_RECIPIENT_ENDPOINT 0x02 00081 #define USB_REQ_RECIPIENT_MASK 0x03 00082 00083 #define USB_REQ_GET_STATUS 0x00 00084 #define USB_REQ_CLEAR_FEATURE 0x01 00085 #define USB_REQ_SET_FEATURE 0x03 00086 #define USB_REQ_SET_ADDRESS 0x05 00087 #define USB_REQ_GET_DESCRIPTOR 0x06 00088 #define USB_REQ_SET_DESCRIPTOR 0x07 00089 #define USB_REQ_GET_CONFIGURATION 0x08 00090 #define USB_REQ_SET_CONFIGURATION 0x09 00091 #define USB_REQ_GET_INTERFACE 0x0A 00092 #define USB_REQ_SET_INTERFACE 0x0B 00093 #define USB_REQ_SYNCH_FRAME 0x0C 00094 00095 #define USB_DESC_TYPE_DEVICE 1 00096 #define USB_DESC_TYPE_CONFIGURATION 2 00097 #define USB_DESC_TYPE_STRING 3 00098 #define USB_DESC_TYPE_INTERFACE 4 00099 #define USB_DESC_TYPE_ENDPOINT 5 00100 #define USB_DESC_TYPE_DEVICE_QUALIFIER 6 00101 #define USB_DESC_TYPE_OTHER_SPEED_CONFIGURATION 7 00102 #define USB_DESC_TYPE_BOS 0x0F 00103 00104 #define USB_CONFIG_REMOTE_WAKEUP 2 00105 #define USB_CONFIG_SELF_POWERED 1 00106 00107 #define USB_FEATURE_EP_HALT 0 00108 #define USB_FEATURE_REMOTE_WAKEUP 1 00109 #define USB_FEATURE_TEST_MODE 2 00110 00111 #define USB_DEVICE_CAPABITY_TYPE 0x10 00112 00113 #define USB_HS_MAX_PACKET_SIZE 512 00114 #define USB_FS_MAX_PACKET_SIZE 64 00115 #define USB_MAX_EP0_SIZE 64 00116 00117 /* Device Status */ 00118 #define USBD_STATE_DEFAULT 1 00119 #define USBD_STATE_ADDRESSED 2 00120 #define USBD_STATE_CONFIGURED 3 00121 #define USBD_STATE_SUSPENDED 4 00122 00123 00124 /* EP0 State */ 00125 #define USBD_EP0_IDLE 0 00126 #define USBD_EP0_SETUP 1 00127 #define USBD_EP0_DATA_IN 2 00128 #define USBD_EP0_DATA_OUT 3 00129 #define USBD_EP0_STATUS_IN 4 00130 #define USBD_EP0_STATUS_OUT 5 00131 #define USBD_EP0_STALL 6 00132 00133 #define USBD_EP_TYPE_CTRL 0 00134 #define USBD_EP_TYPE_ISOC 1 00135 #define USBD_EP_TYPE_BULK 2 00136 #define USBD_EP_TYPE_INTR 3 00137 00138 00139 /** 00140 * @} 00141 */ 00142 00143 00144 /** @defgroup USBD_DEF_Exported_TypesDefinitions 00145 * @{ 00146 */ 00147 00148 typedef struct usb_setup_req 00149 { 00150 00151 uint8_t bmRequest; 00152 uint8_t bRequest; 00153 uint16_t wValue; 00154 uint16_t wIndex; 00155 uint16_t wLength; 00156 }USBD_SetupReqTypedef; 00157 00158 struct _USBD_HandleTypeDef; 00159 00160 typedef struct _Device_cb 00161 { 00162 uint8_t (*Init) (struct _USBD_HandleTypeDef *pdev , uint8_t cfgidx); 00163 uint8_t (*DeInit) (struct _USBD_HandleTypeDef *pdev , uint8_t cfgidx); 00164 /* Control Endpoints*/ 00165 uint8_t (*Setup) (struct _USBD_HandleTypeDef *pdev , USBD_SetupReqTypedef *req); 00166 uint8_t (*EP0_TxSent) (struct _USBD_HandleTypeDef *pdev ); 00167 uint8_t (*EP0_RxReady) (struct _USBD_HandleTypeDef *pdev ); 00168 /* Class Specific Endpoints*/ 00169 uint8_t (*DataIn) (struct _USBD_HandleTypeDef *pdev , uint8_t epnum); 00170 uint8_t (*DataOut) (struct _USBD_HandleTypeDef *pdev , uint8_t epnum); 00171 uint8_t (*SOF) (struct _USBD_HandleTypeDef *pdev); 00172 uint8_t (*IsoINIncomplete) (struct _USBD_HandleTypeDef *pdev , uint8_t epnum); 00173 uint8_t (*IsoOUTIncomplete) (struct _USBD_HandleTypeDef *pdev , uint8_t epnum); 00174 00175 uint8_t *(*GetHSConfigDescriptor)(uint16_t *length); 00176 uint8_t *(*GetFSConfigDescriptor)(uint16_t *length); 00177 uint8_t *(*GetOtherSpeedConfigDescriptor)(uint16_t *length); 00178 uint8_t *(*GetDeviceQualifierDescriptor)(uint16_t *length); 00179 #if (USBD_SUPPORT_USER_STRING == 1) 00180 uint8_t *(*GetUsrStrDescriptor)(struct _USBD_HandleTypeDef *pdev ,uint8_t index, uint16_t *length); 00181 #endif 00182 00183 } USBD_ClassTypeDef; 00184 00185 /* Following USB Device Speed */ 00186 typedef enum 00187 { 00188 USBD_SPEED_HIGH = 0, 00189 USBD_SPEED_FULL = 1, 00190 USBD_SPEED_LOW = 2, 00191 }USBD_SpeedTypeDef; 00192 00193 /* Following USB Device status */ 00194 typedef enum { 00195 USBD_OK = 0, 00196 USBD_BUSY, 00197 USBD_FAIL, 00198 }USBD_StatusTypeDef; 00199 00200 /* USB Device descriptors structure */ 00201 typedef struct 00202 { 00203 uint8_t *(*GetDeviceDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00204 uint8_t *(*GetLangIDStrDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00205 uint8_t *(*GetManufacturerStrDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00206 uint8_t *(*GetProductStrDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00207 uint8_t *(*GetSerialStrDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00208 uint8_t *(*GetConfigurationStrDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00209 uint8_t *(*GetInterfaceStrDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00210 #if (USBD_LPM_ENABLED == 1) 00211 uint8_t *(*GetBOSDescriptor)( USBD_SpeedTypeDef speed , uint16_t *length); 00212 #endif 00213 } USBD_DescriptorsTypeDef; 00214 00215 /* USB Device handle structure */ 00216 typedef struct 00217 { 00218 uint32_t status; 00219 uint32_t total_length; 00220 uint32_t rem_length; 00221 uint32_t maxpacket; 00222 } USBD_EndpointTypeDef; 00223 00224 /* USB Device handle structure */ 00225 typedef struct _USBD_HandleTypeDef 00226 { 00227 uint8_t id; 00228 uint32_t dev_config; 00229 uint32_t dev_default_config; 00230 uint32_t dev_config_status; 00231 USBD_SpeedTypeDef dev_speed; 00232 USBD_EndpointTypeDef ep_in[15]; 00233 USBD_EndpointTypeDef ep_out[15]; 00234 uint32_t ep0_state; 00235 uint32_t ep0_data_len; 00236 uint8_t dev_state; 00237 uint8_t dev_old_state; 00238 uint8_t dev_address; 00239 uint8_t dev_connection_status; 00240 uint8_t dev_test_mode; 00241 uint32_t dev_remote_wakeup; 00242 00243 USBD_SetupReqTypedef request; 00244 USBD_DescriptorsTypeDef *pDesc; 00245 USBD_ClassTypeDef *pClass; 00246 void *pClassData; 00247 void *pUserData; 00248 void *pData; 00249 } USBD_HandleTypeDef; 00250 00251 /** 00252 * @} 00253 */ 00254 00255 00256 00257 /** @defgroup USBD_DEF_Exported_Macros 00258 * @{ 00259 */ 00260 #define SWAPBYTE(addr) (((uint16_t)(*((uint8_t *)(addr)))) + \ 00261 (((uint16_t)(*(((uint8_t *)(addr)) + 1))) << 8)) 00262 00263 #define LOBYTE(x) ((uint8_t)(x & 0x00FF)) 00264 #define HIBYTE(x) ((uint8_t)((x & 0xFF00) >>8)) 00265 #define MIN(a, b) (((a) < (b)) ? (a) : (b)) 00266 #define MAX(a, b) (((a) > (b)) ? (a) : (b)) 00267 00268 00269 #if defined ( __GNUC__ ) 00270 #ifndef __weak 00271 #define __weak __attribute__((weak)) 00272 #endif /* __weak */ 00273 #ifndef __packed 00274 #define __packed __attribute__((__packed__)) 00275 #endif /* __packed */ 00276 #endif /* __GNUC__ */ 00277 00278 00279 /* In HS mode and when the DMA is used, all variables and data structures dealing 00280 with the DMA during the transaction process should be 4-bytes aligned */ 00281 00282 #if defined (__GNUC__) /* GNU Compiler */ 00283 #define __ALIGN_END __attribute__ ((aligned (4))) 00284 #define __ALIGN_BEGIN 00285 #else 00286 #define __ALIGN_END 00287 #if defined (__CC_ARM) /* ARM Compiler */ 00288 #define __ALIGN_BEGIN __align(4) 00289 #elif defined (__ICCARM__) /* IAR Compiler */ 00290 #define __ALIGN_BEGIN 00291 #elif defined (__TASKING__) /* TASKING Compiler */ 00292 #define __ALIGN_BEGIN __align(4) 00293 #endif /* __CC_ARM */ 00294 #endif /* __GNUC__ */ 00295 00296 00297 /** 00298 * @} 00299 */ 00300 00301 /** @defgroup USBD_DEF_Exported_Variables 00302 * @{ 00303 */ 00304 00305 /** 00306 * @} 00307 */ 00308 00309 /** @defgroup USBD_DEF_Exported_FunctionsPrototype 00310 * @{ 00311 */ 00312 00313 /** 00314 * @} 00315 */ 00316 00317 #ifdef __cplusplus 00318 } 00319 #endif 00320 00321 #endif /* __USBD_DEF_H */ 00322 00323 /** 00324 * @} 00325 */ 00326 00327 /** 00328 * @} 00329 */ 00330 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00331
Generated on Thu Jul 14 2022 15:47:45 by
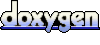