USB CDC library for MBED on STM32
Embed:
(wiki syntax)
Show/hide line numbers
usbd_cdc.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file usbd_cdc.h 00004 * @author MCD Application Team 00005 * @version V2.4.2 00006 * @date 11-December-2015 00007 * @brief header file for the usbd_cdc.c file. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT 2015 STMicroelectronics</center></h2> 00012 * 00013 * Licensed under MCD-ST Liberty SW License Agreement V2, (the "License"); 00014 * You may not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at: 00016 * 00017 * http://www.st.com/software_license_agreement_liberty_v2 00018 * 00019 * Unless required by applicable law or agreed to in writing, software 00020 * distributed under the License is distributed on an "AS IS" BASIS, 00021 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00022 * See the License for the specific language governing permissions and 00023 * limitations under the License. 00024 * 00025 ****************************************************************************** 00026 */ 00027 00028 /* Define to prevent recursive inclusion -------------------------------------*/ 00029 #ifndef __USB_CDC_H 00030 #define __USB_CDC_H 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 /* Includes ------------------------------------------------------------------*/ 00037 #include "usbd_ioreq.h" 00038 #include "usbd_desc.h" 00039 00040 /** @addtogroup STM32_USB_DEVICE_LIBRARY 00041 * @{ 00042 */ 00043 00044 /** @defgroup usbd_cdc 00045 * @brief This file is the Header file for usbd_cdc.c 00046 * @{ 00047 */ 00048 00049 00050 /** @defgroup usbd_cdc_Exported_Defines 00051 * @{ 00052 */ 00053 #define CDC_IN_EP 0x81 /* EP1 for data IN */ 00054 #define CDC_OUT_EP 0x01 /* EP1 for data OUT */ 00055 #define CDC_CMD_EP 0x82 /* EP2 for CDC commands */ 00056 00057 /* CDC Endpoints parameters: you can fine tune these values depending on the needed baudrates and performance. */ 00058 #define CDC_DATA_HS_MAX_PACKET_SIZE 512 /* Endpoint IN & OUT Packet size */ 00059 #define CDC_DATA_FS_MAX_PACKET_SIZE 64 /* Endpoint IN & OUT Packet size */ 00060 #define CDC_CMD_PACKET_SIZE 8 /* Control Endpoint Packet size */ 00061 00062 #define USB_CDC_CONFIG_DESC_SIZ 75 00063 #define CDC_DATA_HS_IN_PACKET_SIZE CDC_DATA_HS_MAX_PACKET_SIZE 00064 #define CDC_DATA_HS_OUT_PACKET_SIZE CDC_DATA_HS_MAX_PACKET_SIZE 00065 00066 #define CDC_DATA_FS_IN_PACKET_SIZE CDC_DATA_FS_MAX_PACKET_SIZE 00067 #define CDC_DATA_FS_OUT_PACKET_SIZE CDC_DATA_FS_MAX_PACKET_SIZE 00068 00069 /*---------------------------------------------------------------------*/ 00070 /* CDC definitions */ 00071 /*---------------------------------------------------------------------*/ 00072 #define CDC_SEND_ENCAPSULATED_COMMAND 0x00 00073 #define CDC_GET_ENCAPSULATED_RESPONSE 0x01 00074 #define CDC_SET_COMM_FEATURE 0x02 00075 #define CDC_GET_COMM_FEATURE 0x03 00076 #define CDC_CLEAR_COMM_FEATURE 0x04 00077 #define CDC_SET_LINE_CODING 0x20 00078 #define CDC_GET_LINE_CODING 0x21 00079 #define CDC_SET_CONTROL_LINE_STATE 0x22 00080 #define CDC_SEND_BREAK 0x23 00081 00082 /** 00083 * @} 00084 */ 00085 00086 00087 /** @defgroup USBD_CORE_Exported_TypesDefinitions 00088 * @{ 00089 */ 00090 00091 /** 00092 * @} 00093 */ 00094 typedef struct 00095 { 00096 uint32_t bitrate; 00097 uint8_t format; 00098 uint8_t paritytype; 00099 uint8_t datatype; 00100 }USBD_CDC_LineCodingTypeDef; 00101 00102 typedef struct _USBD_CDC_Itf 00103 { 00104 int8_t (* Init) (void); 00105 int8_t (* DeInit) (void); 00106 int8_t (* Control) (uint8_t, uint8_t * , uint16_t); 00107 int8_t (* Received) (uint8_t *, uint32_t *); 00108 int8_t (* Transmitted) (uint8_t *, uint32_t *); 00109 00110 }USBD_CDC_ItfTypeDef; 00111 00112 00113 typedef struct 00114 { 00115 uint32_t data[CDC_DATA_HS_MAX_PACKET_SIZE/4]; /* Force 32bits alignment */ 00116 uint8_t CmdOpCode; 00117 uint8_t CmdLength; 00118 uint8_t *RxBuffer; 00119 uint8_t *TxBuffer; 00120 uint32_t RxLength; 00121 uint32_t TxLength; 00122 00123 __IO uint32_t TxState; 00124 __IO uint32_t RxState; 00125 } 00126 USBD_CDC_HandleTypeDef; 00127 00128 00129 00130 /** @defgroup USBD_CORE_Exported_Macros 00131 * @{ 00132 */ 00133 00134 /** 00135 * @} 00136 */ 00137 00138 /** @defgroup USBD_CORE_Exported_Variables 00139 * @{ 00140 */ 00141 00142 extern USBD_ClassTypeDef USBD_CDC; 00143 #define USBD_CDC_CLASS &USBD_CDC 00144 /** 00145 * @} 00146 */ 00147 00148 /** @defgroup USB_CORE_Exported_Functions 00149 * @{ 00150 */ 00151 uint8_t USBD_CDC_RegisterInterface (USBD_HandleTypeDef *pdev, 00152 USBD_CDC_ItfTypeDef *fops); 00153 00154 uint8_t USBD_CDC_SetTxBuffer (USBD_HandleTypeDef *pdev, 00155 uint8_t *pbuff, 00156 uint16_t length); 00157 00158 uint8_t USBD_CDC_SetRxBuffer (USBD_HandleTypeDef *pdev, 00159 uint8_t *pbuff); 00160 00161 uint8_t USBD_CDC_ReceivePacket (USBD_HandleTypeDef *pdev); 00162 00163 uint8_t USBD_CDC_TransmitPacket (USBD_HandleTypeDef *pdev); 00164 /** 00165 * @} 00166 */ 00167 00168 #ifdef __cplusplus 00169 } 00170 #endif 00171 00172 #endif /* __USB_CDC_H */ 00173 /** 00174 * @} 00175 */ 00176 00177 /** 00178 * @} 00179 */ 00180 00181 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00182
Generated on Thu Jul 14 2022 15:47:45 by
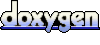